Heap and priority queue are commonly used data structures in C, and they both have important application value. This article will introduce and analyze the heap and priority queue respectively to help readers better understand and use them.
1. Heap
The heap is a special tree data structure that can be used to implement priority queues. In the heap, each node satisfies the following properties:
- Its value is not less than (or not greater than) the value of its parent node.
- Its left and right subtrees are also a heap.
We call a heap that is no smaller than its parent node a "min heap" and a heap that is no larger than its parent node a "max heap". In addition, the heap is usually a complete binary tree, that is, the nodes in other levels are full except for the last level, where the nodes are arranged from left to right.
In C, the STL library provides two classes, heap and priority_queue, to implement the heap. Among them, the heap class provides heap operation functions, such as make_heap, push_heap, pop_heap, etc., and the priority_queue class is a priority queue based on the heap implementation. Next, let's take a look at the usage of heap and priority_queue.
- heap class
(1) Create a heap
Before creating a heap, you need to create a vector type array to store the numbers to be sorted :
vector
Then, we can use the make_heap function to vector The array of type is converted to a heap:
make_heap(v.begin(), v.end(), greater
where greater
(2) Insert elements
To insert elements into the heap, you can use the push_heap function. Its usage is as follows:
v.push_back(7);
push_heap( v.begin(), v.end(), greater
(3) Delete elements
To delete elements in the heap, you can use the pop_heap function, which will The top element of the heap is moved to the last position of the heap and the element is removed from the heap. The usage of this function is as follows:
pop_heap(v.begin(), v.end(), greater
v.pop_back();
- priority_queue class
(1) Create a heap
Unlike the heap class, the priority_queue class can specify the type of heap when declaring the object, as follows:
priority_queue
This means creating a minimum heap.
(2) Insert elements
Inserting elements into priority_queue can directly use the push function, as shown below:
q.push(2);
q. push(4);
q.push(1);
q.push(9);
(3) Delete elements
You can use it directly to delete elements in priority_queue The pop function is as follows:
q.pop();
2. Priority queue
The priority queue is a special queue that sorts elements according to their priority. Sort and place the elements with the highest priority at the front of the queue and the elements with the lowest priority at the end of the queue. You can use a heap to implement a priority queue.
In C, the priority_queue class is a priority queue implemented based on the heap. It can use the functions in the above heap and priority_queue classes to perform element insertion and deletion operations. In addition, the top function is also provided in the priority_queue class, which is used to access the element with the highest priority, as shown below:
priority_queue Summary: This article introduces the heap and priority queue in C, and analyzes the usage and implementation principles of the heap and priority queue respectively. By studying this article, readers can better understand these two data structures and use them flexibly in actual programming. At the same time, readers should pay attention to the timing and precautions for using the heap and priority queue to ensure the correctness and efficiency of the code.
q.push(2);
q .push(4);
q.push(1);
q.push(9);
cout
The above is the detailed content of Heap and priority queue in C++. For more information, please follow other related articles on the PHP Chinese website!
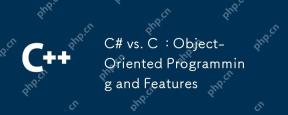
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
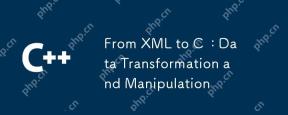
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
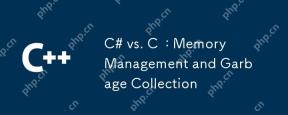
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
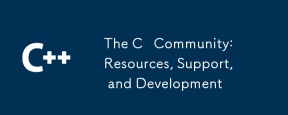
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
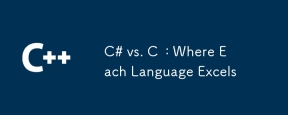
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
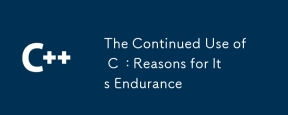
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
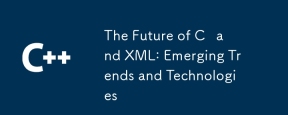
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
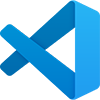
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
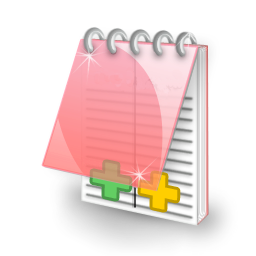
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function