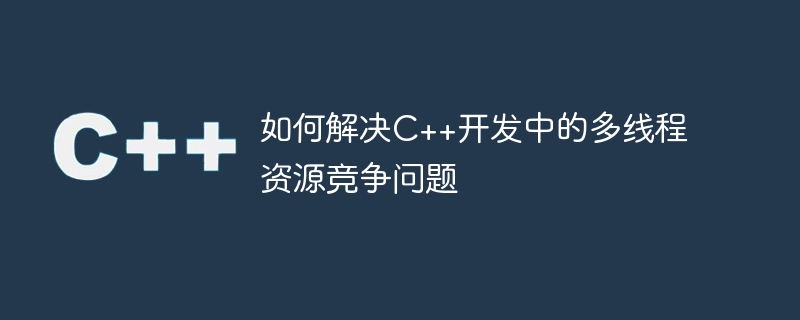
How to solve the multi-threaded resource competition problem in C development
Introduction:
In modern computer applications, multi-threading has become a common development technology. Multi-threading can improve the concurrent execution capabilities of a program and take full advantage of multi-core processors. However, concurrent execution of multiple threads will also bring some problems, the most common of which is resource competition. This article will introduce common multi-threaded resource competition problems in C development and provide some solutions.
1. What is the multi-threaded resource competition problem?
The multi-threaded resource competition problem refers to the problem that when multiple threads access shared resources at the same time, the data may be inconsistent or the program running results may not be consistent with expectations. Race conditions between multiple threads may include read and write operations on shared memory, access to files or databases, control of hardware devices, etc.
2. Common multi-thread resource competition issues
- Competition conditions
Competition conditions refer to multiple threads trying to access the same shared resources at the same time, resulting in uncertain execution result. For example, if multiple threads write to a global variable at the same time, the result may be that the last write operation overwrites the previous result. Race conditions usually occur when there is no reasonable synchronization mechanism between operations between two or more threads.
- Mutual exclusion condition
Mutual exclusion condition means that multiple threads try to access a resource that can only be accessed by a single thread at the same time, resulting in the execution order between multiple threads being disordered. For example, if multiple threads try to open the same file for writing at the same time, the result may be confusion in the file content. Mutual exclusion conditions can usually be resolved with a mutex lock.
- Deadlock
Deadlock refers to a situation where multiple threads are waiting for each other to release resources, causing the program to be unable to continue executing. Deadlock usually occurs when multiple threads compete for resources through mutex locks and wait for each other. To solve the deadlock problem, you need to pay attention to avoid waiting in cycles and release resources reasonably.
3. Common methods to solve multi-thread resource competition problems
- Synchronization mechanism
Using synchronization mechanism is one of the common methods to solve multi-thread resource competition problems. The synchronization mechanism can ensure the execution order between multiple threads and the mutual exclusivity of access to resources. Commonly used synchronization mechanisms include mutex locks, condition variables, semaphores, etc. By properly using synchronization mechanisms, you can avoid problems with race conditions and mutual exclusion conditions.
- Critical Section
Wrap the code segment that may cause a race condition in a critical section, and protect shared resources through a mutex so that only one thread can access this code at the same time. This can avoid data inconsistency problems caused by multiple threads accessing shared resources at the same time.
- Solution to deadlock
To solve the deadlock problem, you need to pay attention to avoid circular waiting and reasonably release resources. You can use the order of resource application to avoid circular waiting, and timely release of acquired resources to avoid deadlock.
- Use atomic operations
For simple data types, you can use atomic operations to ensure atomic access to shared resources. Atomic operations refer to operations that will not be interrupted and can ensure the integrity of the operation. C 11 introduced the atomic operation library, which can easily implement atomic operations.
4. Conclusion
Multi-threaded resource competition is one of the common challenges in C development. Through reasonable use of synchronization mechanisms, critical sections, deadlock resolution, and atomic operations, multi-thread resource competition problems can be effectively solved. In actual development, it is necessary to select appropriate solutions based on specific scenarios and conduct reasonable testing and tuning to ensure the correctness and performance of multi-threaded programs.
Reference:
- Scott Meyers, Effective Modern C, 2014
- Anthony Williams, C Concurrency in Action, 2012
The above is the detailed content of How to solve the multi-threaded resource competition problem in C++ development. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn