How to deal with encoding conversion issues in C development
In the C development process, we often encounter problems that require processing between different encodings. Because there are differences between different encoding formats, you need to pay attention to some details when performing encoding conversion. This article will introduce how to deal with encoding conversion problems in C development.
1. Understand different encoding formats
Before dealing with encoding conversion issues, you first need to understand different encoding formats. Common encoding formats include ASCII, UTF-8, GBK, etc. ASCII is the earliest encoding format, using one byte to represent a character, and only contains English characters and some special characters; UTF-8 is a variable-length encoding format, using 1-4 bytes to represent a character, which can represent the world Almost all characters; GBK is a Chinese character set encoding format that uses 2 bytes to represent a Chinese character.
2. Use appropriate libraries
In C development, you can use some open source libraries to handle encoding conversion problems. Commonly used libraries include iconv, libiconv, and boost. These libraries provide some interfaces and functions to facilitate conversion between encoding formats.
3. Conversion process
The general process for dealing with encoding conversion issues is as follows:
- Read the source text or file and determine the original encoding format.
- Create a conversion context.
- Set source encoding and target encoding.
- Call the conversion function to implement encoding conversion.
- Get the converted result and process it.
4. Sample code
The following is a sample code that handles encoding conversion problems:
#include <iostream> #include <iconv.h> std::string convertEncoding(const std::string& str, const char* from, const char* to) { iconv_t cd = iconv_open(to, from); if (cd == (iconv_t)(-1)) { std::cerr << "Failed to open iconv" << std::endl; return ""; } char* inbuf = const_cast<char*>(str.c_str()); size_t inbytesleft = str.length(); size_t outbytesleft = inbytesleft * 2; char* outbuf = new char[outbytesleft]; size_t ret = iconv(cd, &inbuf, &inbytesleft, &outbuf, &outbytesleft); if (ret == (size_t)(-1)) { std::cerr << "Failed to convert encoding" << std::endl; return ""; } std::string result(outbuf, outbuf + outbytesleft); delete[] outbuf; iconv_close(cd); return result; } int main() { std::string str = "你好,世界!"; std::string utf8Str = convertEncoding(str, "GBK", "UTF-8"); std::cout << utf8Str << std::endl; return 0; }
The above code realizes the conversion by using the iconv library and related functions. Convert GBK encoded string to UTF-8 encoded string. During the conversion process, you need to pay attention to the settings of the source encoding and target encoding, as well as the processing of the conversion results.
5. Precautions
When dealing with encoding conversion issues, you need to pay attention to the following points:
- Determine the source encoding and target encoding to ensure correct encoding conversion .
- Avoid memory leaks and release resources in a timely manner.
- Handle conversion failure to prevent program exceptions.
- Check and verify the conversion results to ensure the accuracy of the conversion results.
Summary: In C development, dealing with encoding conversion issues is a common task. By understanding the different encoding formats, using the appropriate libraries, following the conversion process, and paying attention to a few details, you can effectively handle encoding conversion problems and ensure that your program runs correctly. I hope this article will be helpful to readers on coding conversion issues when developing in C.
The above is the detailed content of How to deal with encoding conversion problems in C++ development. For more information, please follow other related articles on the PHP Chinese website!
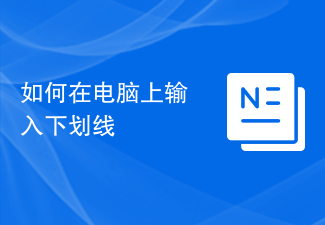
电脑下划线怎么打在电脑输入文字时,我们经常需要使用下划线来突出某些内容或进行标记。然而,对于一些不太熟悉电脑输入法的人来说,打出下划线可能会有些困惑。本文就将向大家介绍如何在电脑上打出下划线。在不同的电脑操作系统和软件中,输入下划线的方式可能会稍有不同。下面将分别介绍Windows操作系统和Mac操作系统上的常用方法。首先,我们先来看一下在Windows操作
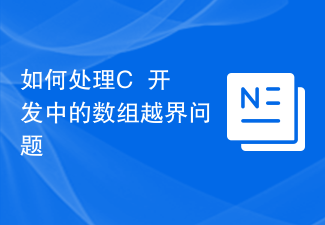
如何处理C++开发中的数组越界问题在C++开发中,数组越界是一个常见的错误,它能导致程序崩溃、数据损坏甚至安全漏洞。因此,正确处理数组越界问题是保证程序质量的重要一环。本文将介绍一些常见的处理方法和建议,帮助开发者避免数组越界问题。首先,了解数组越界问题的原因是关键。数组越界指的是访问数组时超出了其定义范围的索引。这通常发生在以下场景中:访问数组时使用了负数
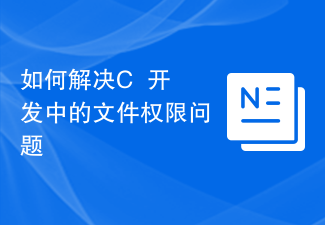
如何解决C++开发中的文件权限问题在C++开发过程中,文件权限问题是一个常见的挑战。在许多情况下,我们需要以不同的权限访问和操作文件,例如读取、写入、执行和删除文件。本文将介绍一些解决C++开发中文件权限问题的方法。一、了解文件权限在解决文件权限问题之前,我们首先需要了解文件权限的基本概念。文件权限指的是文件的拥有者、拥有组和其他用户对文件的访问权限。在Li
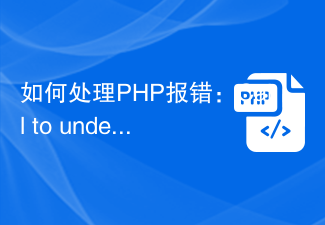
如何处理PHP报错:Calltoundefinedfunction的问题?在使用PHP开发过程中,经常会遇到各种报错。其中一个常见的报错是"Calltoundefinedfunction",意味着调用了一个未定义的函数。这种报错可能会导致代码运行失败,给开发者带来困扰。本文将介绍如何处理这种报错,并提供一些代码示例。检查函数是否被正确
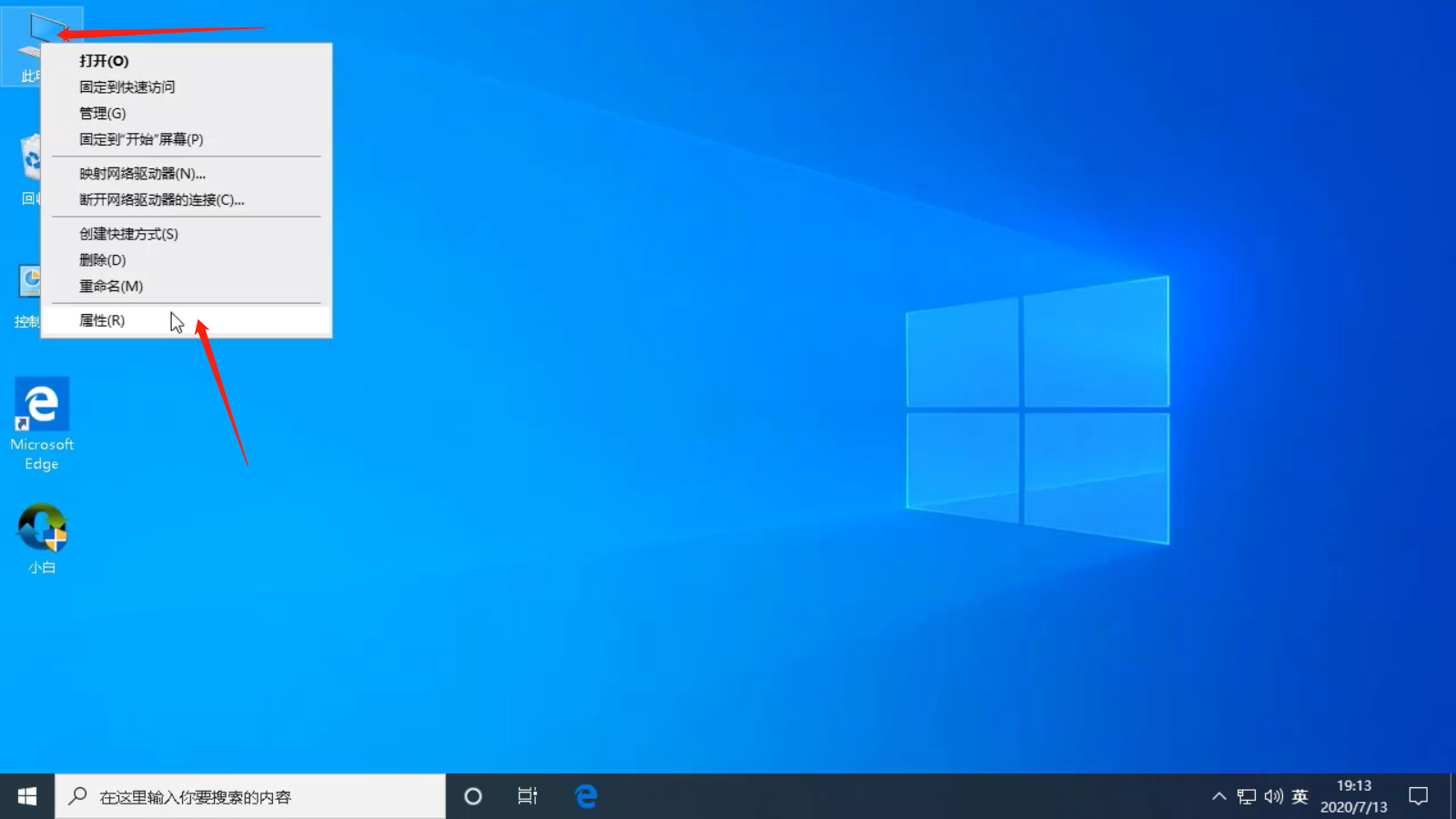
网络适配器是计算机连接网络的工具。没有网络适配器,计算机就无法连接网络。那么,当我们发现网络适配器不见了,我们该如何解决它呢?接下来,小编将为您带来解决网络适配器不见的方法。win10网络适配器不见了怎么办?1.首先,在电脑桌面上,鼠标右键单击打开这台电脑菜单栏,选择属性选项。2.在打开的系统界面中,选择左栏菜单中的设备管理器选项。3.然后打开设备管理器界面,点击三角图标展开网络适配器,右键单击正在使用的网卡驱动,选择更新驱动程序软件选项。4.在两个选项中,我们选择自动搜索更新驱动程序软件,让系
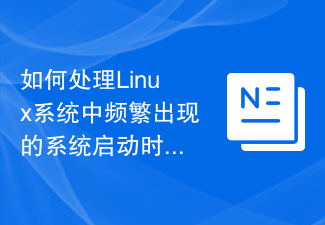
如何处理Linux系统中频繁出现的系统启动时间过长问题摘要:本文介绍了处理Linux系统中频繁出现的系统启动时间过长问题的常见方法和技巧,包括优化启动脚本、清理无用服务、简化启动流程和提升硬件性能等。关键词:Linux系统、系统启动、时间过长、优化方法引言:Linux作为一种自由开放的操作系统,应用广泛,特别在服务器环境中得到了广泛的应用。然而,有时我们会遇
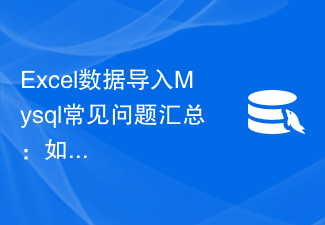
Excel数据导入MySQL是日常数据处理中常见的操作之一,但在实际操作中常会遇到导入速度过慢的问题。导入速度过慢会导致数据处理的效率降低,影响工作进程。本文将介绍一些解决导入速度过慢问题的方法,以帮助读者解决这一问题。首先,导入速度过慢的原因可能有很多,包括网络问题、硬件配置不足以及数据量过大等。因此,在解决导入速度过慢问题之前,首先需要确定导入过程中的瓶
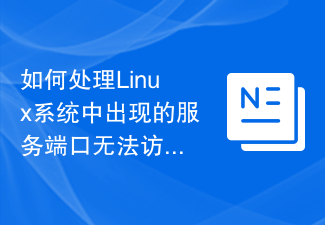
如何处理Linux系统中出现的服务端口无法访问问题在Linux系统中,服务端口无法访问是一个常见的问题。当我们需要使用特定的服务时,如果发现无法访问其相应的端口,就需要进行排查和解决。本文将介绍一些解决此问题的方法和步骤。首先,我们需要确认服务是否启动,可以使用以下命令来检查服务的运行情况:systemctlstatus<service_name&


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
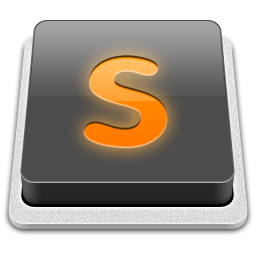
SublimeText3 Mac version
God-level code editing software (SublimeText3)
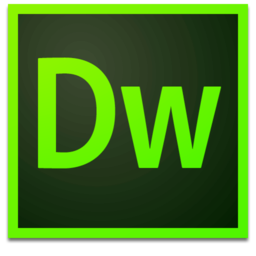
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use
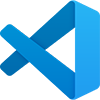
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version
