Introduction to stacks and queues in C
Stacks and queues are commonly used data structures in C, and they are widely used in programs. This article will introduce the concepts, usage and application scenarios of stacks and queues in detail.
1. The concept of stack
Stack is a linear data structure, which has the characteristics of "first in, last out". In the stack, the data pushed into the stack is closer to the bottom of the stack; the data pushed into the stack later is closer to the top of the stack.
The main operations of the stack are push and pop. Pushing is to add data to the stack, and popping is to delete data from the stack. The stack also has two important special operations: viewing the top element of the stack (top) and determining whether the stack is empty (empty).
The application scenarios of the stack are very wide. For example, the use of the stack is involved when calling functions. When a function is called, its parameters, local variables and other information will be pushed onto the stack. When the function execution ends, this information will be popped from the stack and restored to the state before the function call.
2. The concept of queue
Queue (Queue) is also a linear data structure, which has the characteristics of "first in, first out". In the queue, the data that is queued earlier is closer to the head of the queue; the data that is queued later is closer to the end of the queue.
The main operations of the queue are enqueue and dequeue. Entering the queue means adding data to the end of the queue, while dequeuing means deleting data from the head of the queue. The queue also has two important special operations: viewing the head element (front) and determining whether the queue is empty (empty).
Queues are also widely used. For example, in process scheduling in the operating system, queues can be used to save processes waiting to be executed. When system resources are free, a process is taken out from the head of the queue and executed until all tasks are completed.
3. Application examples of stacks and queues
- Bracket matching
In programming, it is often necessary to determine whether the brackets in a string match. For example, when writing a Python program, if you need to check whether the code block is indented correctly, you can use the stack to achieve this.
The specific implementation method is to traverse every character in the string and push it onto the stack when encountering a left bracket. When a right parenthesis is encountered, the top element of the stack is popped for matching. If the match is successful, continue traversing; otherwise, an error message is returned.
- Process Scheduling
In the operating system, it is necessary to achieve unified scheduling and coordination of processes. At this time, you can use a queue to store processes waiting for execution, and the operating system determines the priority and execution order.
The specific implementation method is to abstract each process into a data structure, including process number, priority and other information. Put these processes into a queue, and then execute the processes in the queue in sequence. When a process completes its task, it will be popped out of the queue until the queue is empty.
4. Implementation of stacks and queues in C
In C, you can use the container classes provided by the standard library to implement stacks and queues.
- Implementation of stack
The stack can be implemented using the container class std::stack.
std::stack is a template class that requires specifying the element type and underlying container type. When the underlying container type is not specified, std::deque is used as the underlying container by default.
The following is a simple stack implementation example:
#include <iostream> #include <stack> int main() { std::stack<int> s; s.push(1); s.push(2); s.push(3); std::cout << s.top() << std::endl; // 输出3 s.pop(); std::cout << s.top() << std::endl; // 输出2 while (!s.empty()) { s.pop(); } return 0; }
- Queue implementation
The queue can be implemented using the container class std::queue.
std::queue is also a template class and needs to specify the element type and underlying container type. When the underlying container type is not specified, std::deque is used as the underlying container by default.
The following is a simple queue implementation example:
#include <iostream> #include <queue> int main() { std::queue<int> q; q.push(1); q.push(2); q.push(3); std::cout << q.front() << std::endl; // 输出1 q.pop(); std::cout << q.front() << std::endl; // 输出2 while (!q.empty()) { q.pop(); } return 0; }
Summary
As can be seen from the above introduction, stacks and queues are very practical data structures that can help We solve a lot of real problems. In programming, mastering the usage and implementation principles of these two data structures can improve the efficiency and reliability of the program.
The above is the detailed content of Stack and Queue in C++. For more information, please follow other related articles on the PHP Chinese website!
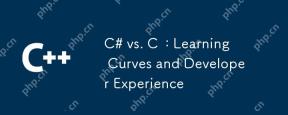
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
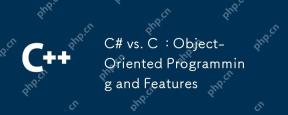
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
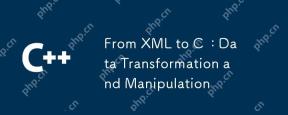
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
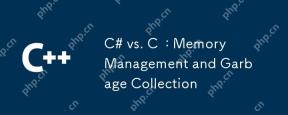
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
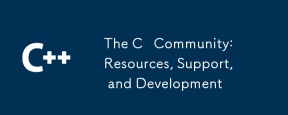
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
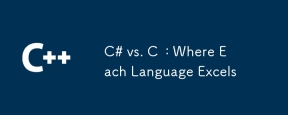
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
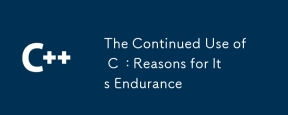
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
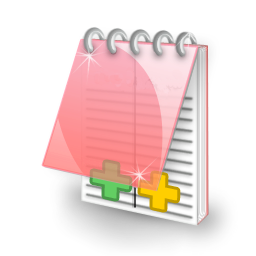
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
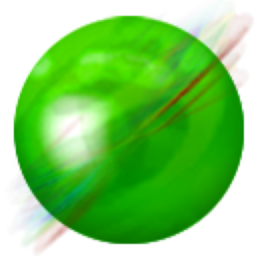
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment