Recursive algorithm is a very important concept in programming. The implementation of this algorithm is often relatively simple and also has strong practicality. Various recursive algorithms can be easily implemented using C. This article will introduce how to use C to implement recursive algorithms.
What is a recursive algorithm?
Recursive algorithm refers to an algorithm that calls itself in a function. It is usually suitable for situations where multiple operations need to be performed on the same problem, but the data required for each operation is small. Recursive algorithms can make programs more concise and clear, while also reducing the amount of code and improving code readability.
Steps to implement recursive algorithm
- Define recursive function
First you need to define a recursive function, which will call itself to complete the recursive calculation. When defining a recursive function, you need to ensure that the parameter type, return value type, and function name of the function are correct.
- Determine the recursion end condition
In the implementation process of the recursive function, it is necessary to add a statement to determine whether to end the recursion. This needs to be considered based on the actual situation, and the recursion needs to be stopped when certain conditions are reached. Usually, the recursion end condition needs to meet two conditions: first, the problem cannot be further dismantled; second, the final solution has been obtained.
- Write recursive code
Write recursive code based on the definition of the recursive function and the recursive end condition. Inside the recursive function, the parameters need to be processed, and the parameters need to be passed to call the recursive function.
Example 1: Calculating factorial
Calculating factorial is a good example of recursion. We can use C to implement this algorithm.
#include <iostream> using namespace std; int factorial(int n) { if (n == 0) { return 1; } else { return n * factorial(n - 1); } } int main() { int n = 5; cout << n << "的阶乘是:" << factorial(n) << endl; return 0; }
In the above code, we first define a factorial()
function to calculate the factorial, and then call the function in the main()
function. factorial()
In the function, if the passed parameter n
is equal to 0, then 1 is returned; otherwise, the result of n * factorial(n - 1)
is returned.
Example 2: Fibonacci Sequence
The Fibonacci Sequence is also a classic recursive example. We can use C to implement the recursive algorithm of the Fibonacci Sequence.
#include <iostream> using namespace std; int fibonacci(int n) { if (n == 0) { return 0; } else if (n == 1) { return 1; } else { return fibonacci(n - 1) + fibonacci(n - 2); } } int main() { int n = 10; cout << "斐波那契数列前" << n << "项如下:" << endl; for (int i = 0; i < n; i++) { cout << fibonacci(i) << " "; } cout << endl; return 0; }
In the above code, we first define a fibonacci()
function to calculate the Fibonacci sequence, and then calculate it sequentially in the main()
function Fibonacci sequence from 0 to 9 and output the result. fibonacci()
In the function, if the passed parameter n
is equal to 0, then 0 is returned; if the passed parameter n
is equal to 1, then 1 is returned; Otherwise, return the result of fibonacci(n - 1) fibonacci(n - 2)
.
Advantages and Disadvantages of Recursive Algorithms
Using recursive algorithms has its advantages and disadvantages.
Advantages:
- The coding is simple and clear, easy to understand and implement.
- Suitable for scenarios where the problem size is small and the structure is simple.
- Algorithms that can be implemented using recursion are usually easier to implement.
Disadvantages:
- Recursion requires more system overhead, including function calls, parameter transfers, stack operations, etc., so the operating efficiency is low.
- The depth of recursion and the number of recursions are usually limited. Exceeding a certain level will cause stack overflow problems.
- It is necessary to determine the conditions for the end of recursion during the implementation process, which may increase the complexity of the code and the difficulty of debugging.
Summary
Recursive algorithm is an important concept in programming. Using recursion can make the code more concise and readable. When using recursive algorithms, care must be taken to avoid infinite recursion and the efficiency of the algorithm must be considered. The C language provides powerful tools to support the implementation of recursive algorithms, and can quickly and efficiently implement various recursive algorithms.
The above is the detailed content of Implementing recursive algorithms using C++. For more information, please follow other related articles on the PHP Chinese website!
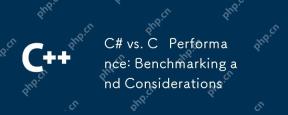
The performance differences between C# and C are mainly reflected in execution speed and resource management: 1) C usually performs better in numerical calculations and string operations because it is closer to hardware and has no additional overhead such as garbage collection; 2) C# is more concise in multi-threaded programming, but its performance is slightly inferior to C; 3) Which language to choose should be determined based on project requirements and team technology stack.
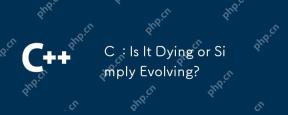
C isnotdying;it'sevolving.1)C remainsrelevantduetoitsversatilityandefficiencyinperformance-criticalapplications.2)Thelanguageiscontinuouslyupdated,withC 20introducingfeatureslikemodulesandcoroutinestoimproveusabilityandperformance.3)Despitechallen
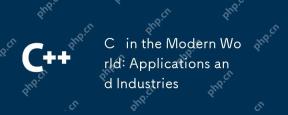
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
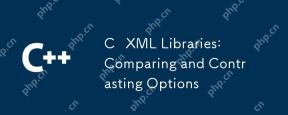
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
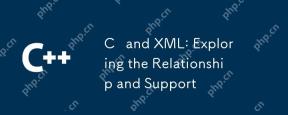
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
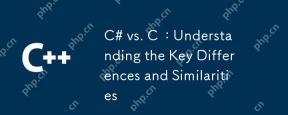
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
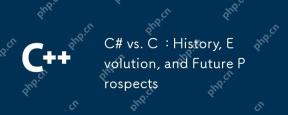
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
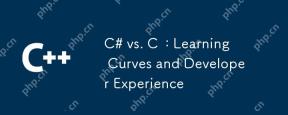
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
