


C++ syntax error: A constructor with only a single parameter must be declared explicit. How to solve it?
In C programming, you may encounter the following error message: A constructor with only a single parameter must be declared explicit. This error message may confuse beginners. Next, let’s take a look at what explicit is in C, the reasons why this error message appears, and how to solve this problem.
The role of explicit
In C, if we define a constructor that only receives one parameter, then we need to explicitly declare the constructor using the keyword explicit. Using explicit can avoid implicit conversion problems and prevent objects of one type from being implicitly converted to objects of another type.
For example, if we have a constructor that receives a string parameter, if it is not declared using the explicit keyword, then in some cases, the constructor will be called implicitly, which may leading to some potential problems. The following is an example that demonstrates this problem:
#include <iostream> #include <string> class Person { public: Person(const std::string& name) : name_(name) { } std::string GetName() const { return name_; } private: std::string name_; }; void DisplayPerson(const Person& p) { std::cout << "Person's name is " << p.GetName() << std::endl; } int main() { // 隐式调用 Person 的构造函数 DisplayPerson("Tom"); return 0; }
The above code will report an error: a constructor with only a single parameter must be declared explicit. This is because in the DisplayPerson function, we use a string constant as a parameter to pass to the Person type, resulting in implicit conversion.
In order to solve this problem, we can add the explicit keyword before the constructor, as follows:
explicit Person(const std::string& name) : name_(name) { }
In this way, in the DisplayPerson function, we cannot directly pass in a string constant Yes, you must explicitly create a Person object and then pass it into the function.
int main() { // 显式调用 Person 的构造函数 Person p("Tom"); DisplayPerson(p); return 0; }
Causes and solutions
Only single-parameter constructors must be declared explicit. This error message usually occurs when a single-parameter constructor is used in a program. We should not rely on implicit type conversions when using these constructors. Implicit type conversions introduce type mismatches and erratic behavior, and can lead to hard-to-find bugs.
If this error message appears in your program, you can use the following two methods to solve it:
Method 1: Use the explicit keyword
If you define a receive-only For a one-parameter constructor, you need to explicitly declare the constructor using the explicit keyword. This will prevent implicit type conversions and make the code safer and easier to understand.
explicit ClassName(Type parameter) {};
Where explicit specifies that the single-parameter constructor cannot be called implicitly. ClassName is the name of the class you want to instantiate, Type is the type of the parameter, and parameter is the name of the parameter.
The following is a sample program:
#include <iostream> using namespace std; class Student { public: explicit Student(int id) { m_id = id; } int getId() { return m_id; } private: int m_id; }; int main(int argc, char** argv) { Student std1(1); // 正确 Student std2 = 2; // 错误,必须显式声明,不能进行隐式转换 return 0; }
Method 2: Use type conversion function
The second method is to use type conversion function. If you don't want to use explicit, you can define a conversion function that instantiates the class to the desired type. This is the method commonly used when converting one class to another.
The following is a sample program:
#include <iostream> using namespace std; class Student { public: Student(int id) { m_id = id; } int getId() { return m_id; } operator int() { return m_id; } private: int m_id; }; void display(int id) { cout << "ID: " << id << endl; } int main(int argc, char** argv) { Student std(1); display(std); // 您可以将 Student 对象转换为所需的类型(int) return 0; }
In this example, we use the operator int function to convert the Student class to an integer type. Using this approach, the Student object can be implicitly converted to an integer type and passed to the display() function.
Summary
C’s explicit keyword enables constructors to be created explicitly, thereby avoiding problems caused by potential implicit conversions. During programming, if you encounter the error message "Constructors with only a single parameter must be declared explicit", you can use the above two methods to solve this problem. Remember, explicit code is safer, simpler, and easier to understand and maintain.
The above is the detailed content of C++ syntax error: A constructor with only a single parameter must be declared explicit. How to solve it?. For more information, please follow other related articles on the PHP Chinese website!

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
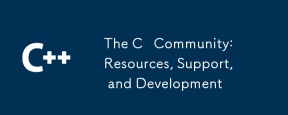
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
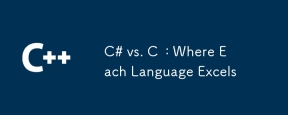
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
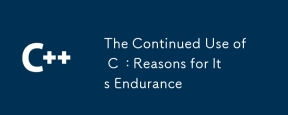
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
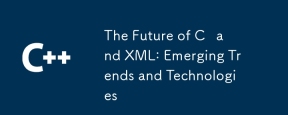
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
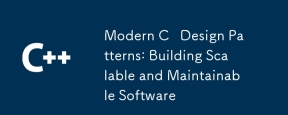
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
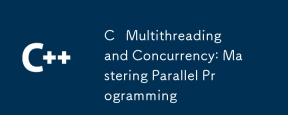
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
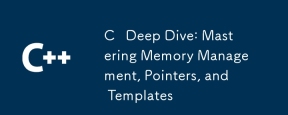
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
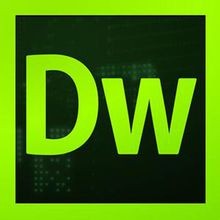
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.