C++ error: dereferencing a null pointer, how to solve it?
In the process of using C, you often encounter some error messages. Among them, dereference of null pointer is a common error. This kind of error will cause the program to crash, seriously affecting the stability and reliability of the program. Therefore, we need to understand what a null pointer is, why the problem of dereferencing a null pointer occurs, and how to avoid and solve this problem.
1. What is a null pointer
In C, a pointer is a variable that stores a memory address. It can point to any type of data, and the data can be accessed through pointers when the program is running. Pointers have a special value, the null pointer (nullptr). A null pointer represents a pointer that does not point to any valid memory address.
In C, a pointer can be initialized to a null pointer. For example:
int* ptr = nullptr; // 初始化为指向整数的空指针
In addition, if a pointer is assigned a value of 0, then it is also a null pointer. For example:
int* ptr = 0; // 初始化为指向整数的空指针
2. Why does the problem of dereferencing a null pointer occur?
Dereferencing a null pointer means using a null pointer to access a memory address in a program. Doing so will cause the program to crash because the null pointer does not point to any valid memory address. The following is a simple sample program:
#include <iostream> int main() { int* ptr = nullptr; std::cout << *ptr << std::endl; return 0; }
A segmentation fault will occur when running this program. This is because the program is trying to read data from the address of a null pointer, which has no corresponding memory address.
The reason for dereferencing a null pointer may be that the pointer of the variable is not properly initialized or the object pointed to by the pointer has been released. When using pointers, you need to ensure that the object pointed to by the pointer is valid and that the pointer itself is not a null pointer.
3. How to avoid the problem of dereferencing a null pointer
In order to avoid the problem of dereferencing a null pointer, you can take the following measures:
- Initialize the pointer
When using a pointer, the pointer should be initialized first. If you are not sure what object the pointer points to, you can initialize the pointer to nullptr or 0. This avoids problems caused by uninitialized pointers.
- Check if the pointer is null
Before operating with the pointer, you should first check if the pointer is nullptr. If the pointer is nullptr, no operation should be performed.
- Avoid releasing memory that has been released
If an object has been released, the pointer to the object is no longer valid. If you continue to use this pointer, there will be a problem of dereferencing the null pointer.
- Using smart pointers
A smart pointer is a pointer that can automatically manage the memory life cycle. It can automatically manage dynamically allocated memory and avoid memory leaks and dereferencing null pointers. There are three types of smart pointers provided in the C 11 standard: unique_ptr, shared_ptr, and weak_ptr.
4. How to solve the problem of dereferencing a null pointer
If the problem of dereferencing a null pointer occurs in the program, you can take the following measures to solve it:
- Check Whether the pointer is null
When the program is running, you can check whether the pointer is nullptr by asserting. If the pointer is null, you can output an error message or take other measures in the program to prevent the program from crashing.
- Error handling mechanism
The exception handling mechanism can be used in the program to deal with the problem of dereferencing null pointers. If the pointer is null, you can throw an exception (Exception) and let the program enter the exception handling stage to avoid program crash.
In short, during the process of program design and implementation, special attention needs to be paid to the use of pointers to avoid problems with null pointers or dereferenced null pointers. Through good program design and the use of smart pointers and other methods, the stability and reliability of the program can be effectively guaranteed.
The above is the detailed content of C++ error: dereferencing a null pointer, how to solve it?. For more information, please follow other related articles on the PHP Chinese website!
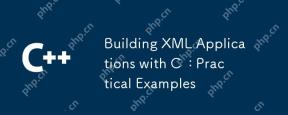
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
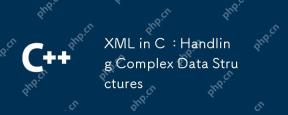
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
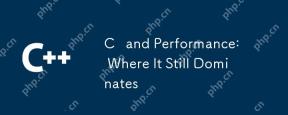
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
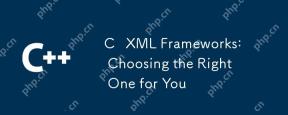
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
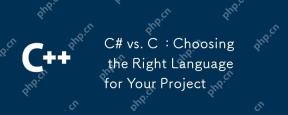
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
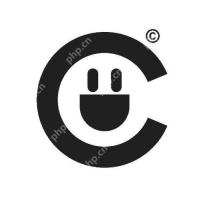
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
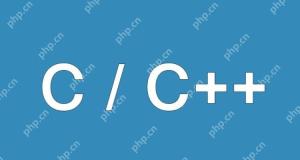
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
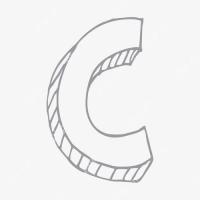
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
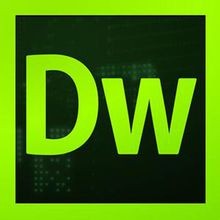
Dreamweaver CS6
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
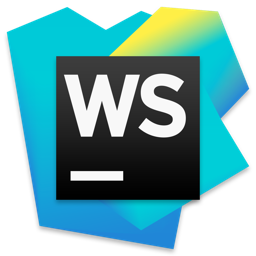
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
