


C++ error: Class members must be initialized in the initialization list, how to deal with it?
In C programming, if there is a member variable in the class definition that is not initialized in the initialization list, the compiler will report an error "Class members must be initialized in the initialization list." This means that when an object is created, the constructor of this member variable will not be called, and may cause instability in the program.
So, in C, when creating objects of a class, you must use the initialization list as much as possible. This article will introduce what an initialization list is and its usage, and how to solve the error that class members are not initialized in the initialization list.
What is an initialization list?
In C, members of a class can be basic data types, arrays, structures, classes, etc. When defining member variables in a class, you can choose to initialize them in the constructor body or use an initialization list (Constructor Initialization List).
The initialization list is a function header of the constructor. You can use the initialization list to initialize members before the constructor. It is a series of initialization operations separated by commas after the colon (:) before the constructor body. In the initialization list, you can initialize multiple member variables at the same time, or you can use the default constructor for initialization, thereby avoiding errors caused by class members not being initialized in the initialization list.
The syntax is as follows:
class SomeClass { public: SomeClass(int a, int b, int c) : var1(a), var2(b), var3(c) { //构造函数体中的其它操作 } private: int var1; int var2; int var3; };
In this example, SomeClass has three member variables: var1, var2, var3. They will be initialized before the constructor, and other operations in the constructor body It will be executed after initializing the list.
Usage of initialization list
- Initialization of object members
If there are other objects in the class as class members, you can also use the initialization list to initialize these member. As shown below:
class Person { public: Person(int _age, int _id) : age(_age), id(_id), car("BMW", "blue") { } private: int age; int id; Car car; //Car 是一个类类型成员 };
In this example, we use the initialization list to initialize the member variables age, id and car. Among them, car is a member variable of the Person class. It is an object of Car type and is declared with "Car car". When we create a Person object, we also need to initialize the car member. When using an initialization list, you need to use the constructor of the Car class to initialize the object.
- Initialization of type conversion
When you need to convert one type to another type, you can use the initialization list:
class A { public: A(int val) : a(val), b(val*1.2), c(val*1.5) {} private: int a; double b; float c; };
In In this example, an int type value is used for initialization, and type conversion can also be performed in the initialization list.
- Initialization of read-only members
Sometimes, member variables in a class need to be read-only and can only be initialized when the object is created, not during construction Modifications within the function body. This can be achieved using an initialization list. As shown below:
class B { public: B() : a(5), b(2), c(a*b) {} private: const int a; const int b; const int c; };
In this example, variables a, b and c are all read-only, and they all need to be initialized when the object is created. Since c is the product of a and b, and both a and b are read-only, they can only be operated on in the initialization list, and the expression assigned to it cannot be modified in the constructor body.
How to deal with the error "Class members must be initialized in the initialization list"?
If the class members are not initialized in the initialization list when creating an object of the class, the compiler will report an error "Class members must be initialized in the initialization list." In order to avoid this error, we can follow the following steps:
- Determine uninitialized member variables.
In the compiler, the error message will indicate which member variables are not initialized in the initialization list. We can follow the prompts to find the corresponding member variables in the source code, such as:
class Test { public: Test() { int a = 0; std::string b; } };
In the Test class, there are two member variables a and b. In the constructor of the class, we did not initialize them in the initialization list, which is why the error was reported.
- Add member variables to the initialization list.
Add uninitialized member variables to the initialization list of the constructor as needed. Modify the code of the constructor to:
class Test { public: Test() : a(0), b("") {} private: int a; std::string b; };
In this example, we use the initialization list to initialize the member variables a and b, thereby avoiding the error "class members must be initialized in the initialization list" .
Notes
In C, using the initialization list can not only avoid errors when class members are not initialized in the initialization list, but also improve the efficiency of the program. If initialization is required in the constructor body, it may cause the constructor of the class member to be called multiple times at the end of the constructor, but using an initialization list will only call it once, thus improving the efficiency of the program.
In addition, when the member variable is of const type, if it is not initialized in the initialization list, the compiler will report an error. When using the initialization list, you should pay attention to the type of member variables and their initialization method.
The above is the detailed content of C++ error: Class members must be initialized in the initialization list, how to deal with it?. For more information, please follow other related articles on the PHP Chinese website!
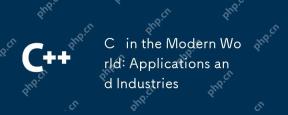
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
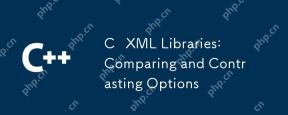
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
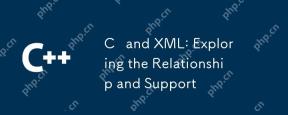
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
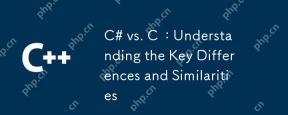
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
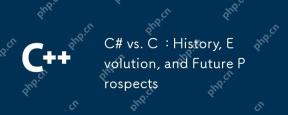
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
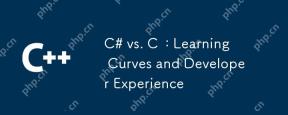
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
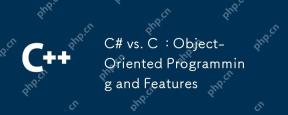
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
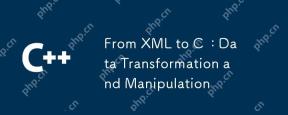
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
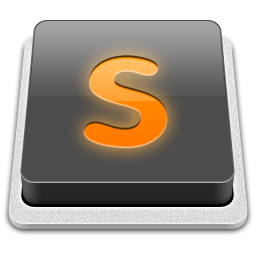
SublimeText3 Mac version
God-level code editing software (SublimeText3)