How to solve the code modularization problem in C++ development
How to solve the code modularization problem in C development
For C developers, code modularization is a common problem. As projects increase in size and complexity, code modularization becomes even more important to improve code maintainability, reusability, and testability. This article will introduce some methods and techniques to help C developers solve code modularization problems.
- Using namespaces
Namespaces are a way in C to organize related code together. By using namespaces, you can separate different functions or modules, avoid naming conflicts, and improve code readability. For example, you can place code related to file input and output in a namespace called "io".
namespace io { // 文件输入输出相关的代码 // ... }
- Dividing classes and functions
Putting related code into classes and functions is another way to modularize your code. By organizing code with similar functionality into classes, you can improve code reusability and maintainability. For example, you can create a class called "Math" and put math-related functions in it.
class Math { public: static int add(int a, int b) { return a + b; } // 其他数学相关的函数 // ... };
- Using header files
Header files are commonly used tools in C development, which can put public function, class and data structure declarations together. By using header files, you can easily reference modular code and reduce repeated code writing. In the header file, you can define the interfaces of related classes and functions and reference them in other files through the #include statement.
// Math.h #ifndef MATH_H #define MATH_H class Math { public: static int add(int a, int b); // 其他数学相关的函数的声明 // ... }; #endif
// Math.cpp #include "Math.h" int Math::add(int a, int b) { return a + b; }
- Use modular development tools
In addition to the above methods, you can also use modular development tools to solve code modularization problems. For example, you can use CMake to manage the modularity of your project. Through the CMake configuration file, different code files, library files and dependencies can be combined together to generate executable files or library files.
# CMakeLists.txt cmake_minimum_required(VERSION 3.10) project(MyProject) set(SOURCES main.cpp io/FileIO.cpp math/Math.cpp ) add_executable(MyProject ${SOURCES})
Summary
By using namespaces, dividing classes and functions, using header files, and using modular development tools, C developers can effectively solve code modularization problems. These methods and techniques can not only improve the maintainability, reusability and testability of the code, but also improve development efficiency and team collaboration. When developing C, we should always pay attention to code modularization to avoid code bloat and confusion, and improve development quality and efficiency.
The above is the detailed content of How to solve the code modularization problem in C++ development. For more information, please follow other related articles on the PHP Chinese website!
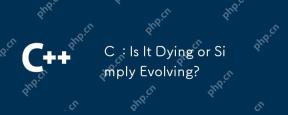
C isnotdying;it'sevolving.1)C remainsrelevantduetoitsversatilityandefficiencyinperformance-criticalapplications.2)Thelanguageiscontinuouslyupdated,withC 20introducingfeatureslikemodulesandcoroutinestoimproveusabilityandperformance.3)Despitechallen
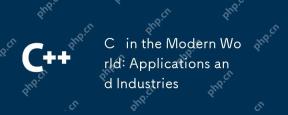
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
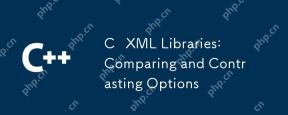
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
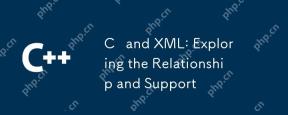
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
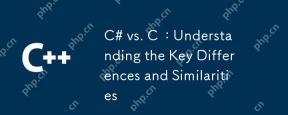
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
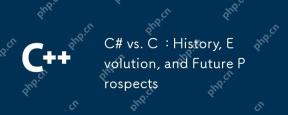
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
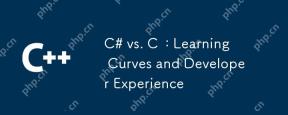
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
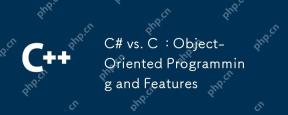
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
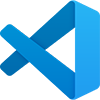
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
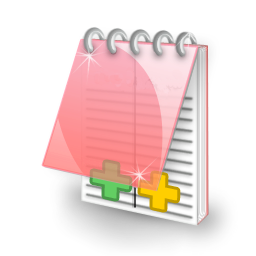
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
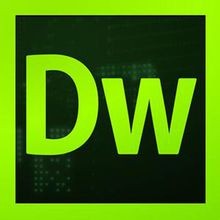
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
