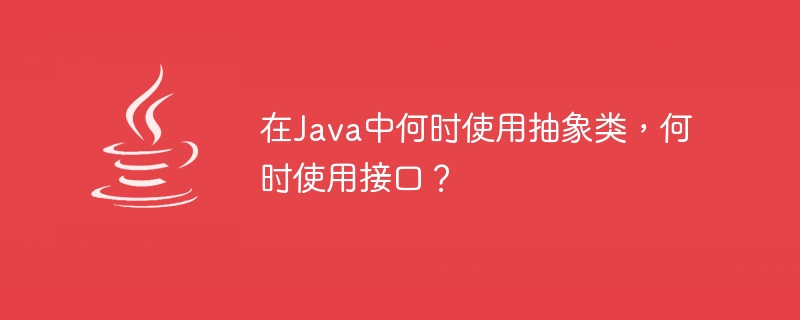
Interfaces can be used to define behavioral contracts and can also be used as contracts for interaction between two systems, while abstract classes are mainly used to define default behaviors for subclasses, which means All subclasses should perform the same functionality.
When to use abstract classes
- If we use the concept of inheritance, abstract classes are a good choice because it provides a common base class implementation for derived classes.
- If we want to declare non-public members, abstract classes are also a good choice. In an interface, all methods must be public.
- If we want to add new methods in the future, then abstract classes are a better choice. Because if we add a new method to an interface, all classes that already implement the interface must be modified to implement the new method.
- If we want to create multiple versions of our component, we can create an abstract class. Abstract classes provide an easy-to-use way to version components. By updating the base class, all inherited classes are automatically updated. Once the interface is created, it cannot be changed. If a new version of an interface is required, a completely new interface must be created.
- Abstract classes have better forward compatibility. Once a client uses an interface, we can't change it; if they use an abstract class, we can still add behavior without breaking existing code.
- If we want to provide common implemented functionality to all implementations of our component, we can use abstract classes. Abstract classes allow us to partially implement the class, whereas interfaces do not contain the implementation of any members.
Example
abstract class Car {
public void accelerate() {
System.out.println("Do something to accelerate");
}
public void applyBrakes() {
System.out.println("Do something to apply brakes");
}
public abstract void changeGears();
}
Now, any car you want to instantiate must implement the changeGears() method.
class Alto extends Car {
public void changeGears() {
System.out.println("Implement changeGears() method for Alto Car");
}
}
class Santro extends Car {
public void changeGears() {
System.out.println("Implement changeGears() method for Santro Car");
}
}
When to use interfaces
- If the functionality we are creating will be used in widely different objects, use interfaces. Abstract classes are primarily used for closely related objects, while interfaces are best suited for providing common functionality to unrelated classes.
- If we think that the API will not change for a period of time, interfaces are a good choice.
- If we want functionality similar to multiple inheritance, interfaces are also a good choice because we can implement multiple interfaces.
- If we design a small and concise functional module, use interfaces. If we are designing a large functional unit, use abstract classes.
Example
public interface Actor {
void perform();
}
public interface Producer {
void invest();
}
Nowadays, most actors are wealthy enough to make their own films. If we use interfaces instead of abstract classes, we can implement both Actor and Producer. In addition, we can also define a new ActorProducer interface, which inherits both.
public interface ActorProducer extends Actor, Producer{
// some statements
}
The above is the detailed content of When to use abstract class and when to use interface in Java?. For more information, please follow other related articles on the PHP Chinese website!