In this article, we will learn how to use Python to perform matrix and linear algebra calculations, such as matrix multiplication, finding determinants, solving linear equations, etc.
You can use a matrix object from the NumPy library to achieve this. When doing calculations, matrices are relatively comparable to array objects.
Linear algebra is a vast subject and beyond the scope of this article.
However, if you need to manipulate matrices and vectors, NumPy is a good starting point.
usage instructions
Find the transpose of a matrix using Numpy
Find the inverse of a matrix using Numpy
Matrix and vector multiplication
Use the numpy.linalg subpackage to obtain the determinant of the matrix
Use numpy.linalg to find eigenvalues
Use numpy.linalg to solve equations
Method 1: Find the transpose of a matrix using Numpy
numpy.matrix.T Properties − Returns the transpose of the given matrix.
The Chinese translation ofExample
is:Example
The following program uses the numpy.matrix.T property to return the transpose of the matrix −
# importing NumPy module import numpy as np # input matrix inputMatrix = np.matrix([[6, 1, 5], [2, 0, 8], [1, 4, 3]]) # printing the input matrix print("Input Matrix:\n", inputMatrix) # printing the transpose of an input matrix # by applying the .T attribute of the NumPy matrix of the numpy Module print("Transpose of an input matrix\n", inputMatrix.T)
Output
When executed, the above program will generate the following output -
Input Matrix: [[6 1 5] [2 0 8] [1 4 3]] Transpose of an input matrix [[6 2 1] [1 0 4] [5 8 3]]
Method 2: Find the inverse of a matrix using Numpy
numpy.matrix.I Properties - Returns the inverse of the given matrix.
The Chinese translation ofExample
is:Example
The following program uses the numpy.matrix.I property to return the inverse of the matrix −
# importing NumPy module import numpy as np # input matrix inputMatrix = np.matrix([[6, 1, 5],[2, 0, 8],[1, 4, 3]]) # printing the input matrix print("Input Matrix:\n", inputMatrix) # printing the inverse of an input matrix # by applying the .I attribute of the NumPy matrix of the numpy Module print("Inverse of an input matrix:\n", inputMatrix.I)
Output
When executed, the above program will generate the following output -
Input Matrix: [[6 1 5] [2 0 8] [1 4 3]] Inverse of an input matrix: [[ 0.21333333 -0.11333333 -0.05333333] [-0.01333333 -0.08666667 0.25333333] [-0.05333333 0.15333333 0.01333333]]
Method 3: Multiplying matrices and vectors
The Chinese translation ofExample
is:Example
The following program uses the * operator to return the product of the input matrix and vector -
# importing numpy module import numpy as np # input matrix inputMatrix = np.matrix([[6, 1, 5],[2, 0, 8],[1, 4, 3]]) # printing the input matrix print("Input Matrix:\n", inputMatrix) # creating a vector using numpy.matrix() function inputVector = np.matrix([[1],[3],[5]]) # printing the multiplication of the input matrix and vector print("Multiplication of input matrix and vector:\n", inputMatrix*inputVector)
Output
When executed, the above program will generate the following output -
Input Matrix: [[6 1 5] [2 0 8] [1 4 3]] Multiplication of input matrix and vector: [[34] [42] [28]]
Method 4: Use the numpy.linalg subpackage to obtain the determinant of the matrix
numpy.linalg.det() Function − Calculate the determinant of a square matrix.
The Chinese translation ofExample
is:Example
The following program uses the numpy.linalg.det() function to return the determinant of the matrix −
# importing numpy module import numpy as np # input matrix inputMatrix = np.matrix([[6, 1, 5],[2, 0, 8],[1, 4, 3]]) # printing the input matrix print("Input Matrix:\n", inputMatrix) # getting the determinant of an input matrix outputDet = np.linalg.det(inputMatrix) # printing the determinant of an input matrix print("Determinant of an input matrix:\n", outputDet)
Output
When executed, the above program will generate the following output -
Input Matrix: [[6 1 5] [2 0 8] [1 4 3]] Determinant of an input matrix: -149.99999999999997
The fifth way to find eigenvalues using numpy.linalg
numpy.linalg.eigvals() function − Calculate the eigenvalues and right eigenvectors of the specified square matrix/matrix.
The Chinese translation ofExample
is:Example
The following program returns the Eigenvalues of an input matrix using the numpy.linalg.eigvals() function −
# importing NumPy module import numpy as np # input matrix inputMatrix = np.matrix([[6, 1, 5],[2, 0, 8],[1, 4, 3]]) # printing the input matrix print("Input Matrix:\n", inputMatrix) # getting Eigenvalues of an input matrix eigenValues = np.linalg.eigvals(inputMatrix) # printing Eigenvalues of an input matrix print("Eigenvalues of an input matrix:\n", eigenValues)
Output
When executed, the above program will generate the following output -
Input Matrix: [[6 1 5] [2 0 8] [1 4 3]] Eigenvalues of an input matrix: [ 9.55480959 3.69447805 -4.24928765]
Method 6: Use numpy.linalg to solve equations
We can solve a problem similar to finding the value of X for A*X = B,
Where A is a matrix and B is a vector.
The Chinese translation ofExample
is:Example
The following is a program that uses the solve() function to return the x value-
# importing NumPy module import numpy as np # input matrix inputMatrix = np.matrix([[6, 1, 5],[2, 0, 8],[1, 4, 3]]) # printing the input matrix print("Input Matrix:\n", inputMatrix) # creating a vector using np.matrix() function inputVector = np.matrix([[1],[3],[5]]) # getting the value of x in an equation inputMatrix * x = inputVector x_value = np.linalg.solve(inputMatrix, inputVector) # printing x value print("x value:\n", x_value) # multiplying input matrix with x values print("Multiplication of input matrix with x values:\n", inputMatrix * x_value)
Output
When executed, the above program will generate the following output -
Input Matrix: [[6 1 5] [2 0 8] [1 4 3]] x value: [[-0.39333333] [ 0.99333333] [ 0.47333333]] Multiplication of input matrix with x values: [[1.] [3.] [5.]]
in conclusion
In this article, we learned how to perform matrix and linear algebra operations using the NumPy module in Python. We learned how to calculate the transpose, inverse, and determinant of a matrix. We also learned how to do some calculations in linear algebra, such as solving equations and determining eigenvalues.
The above is the detailed content of Matrix and linear algebra calculations in Python. For more information, please follow other related articles on the PHP Chinese website!
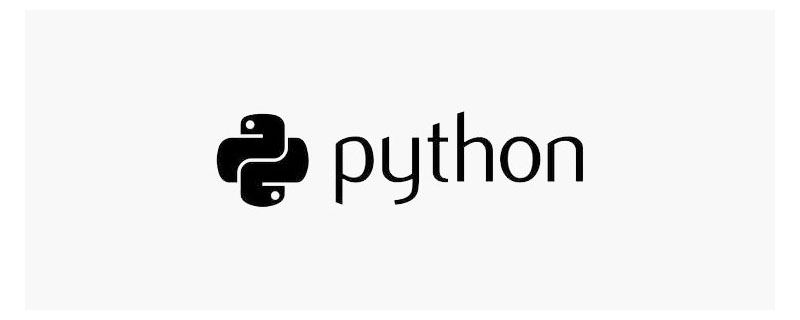
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
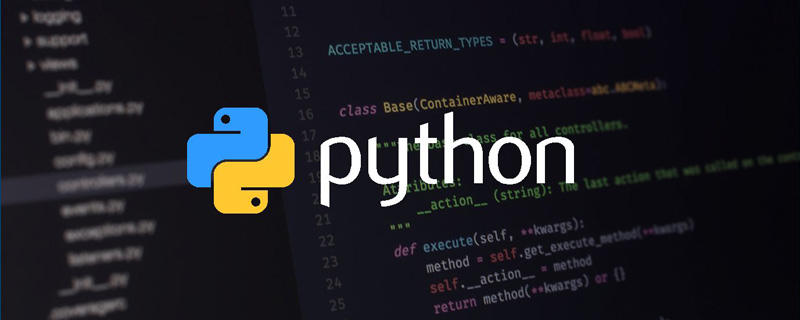
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
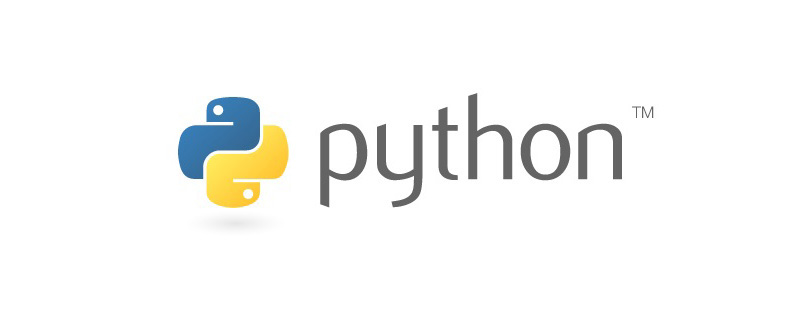
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
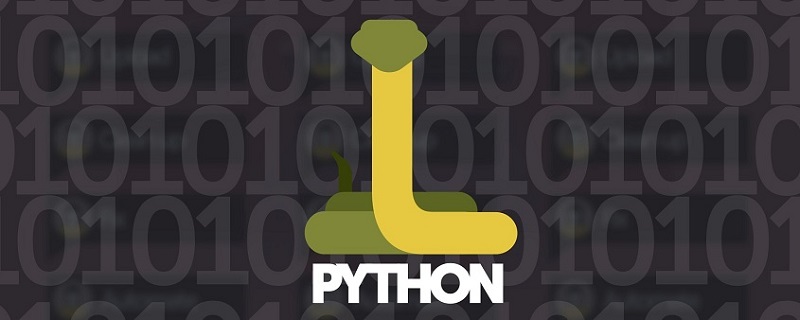
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
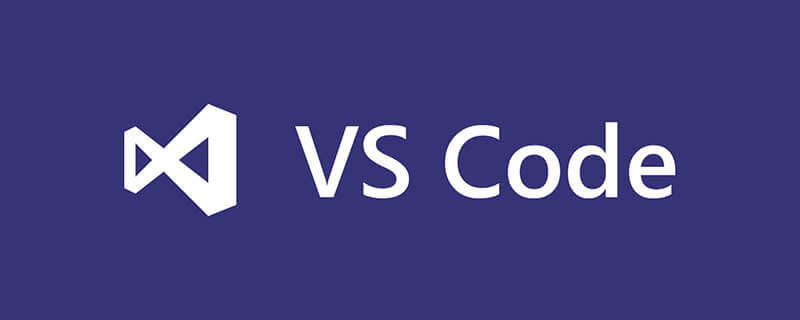
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
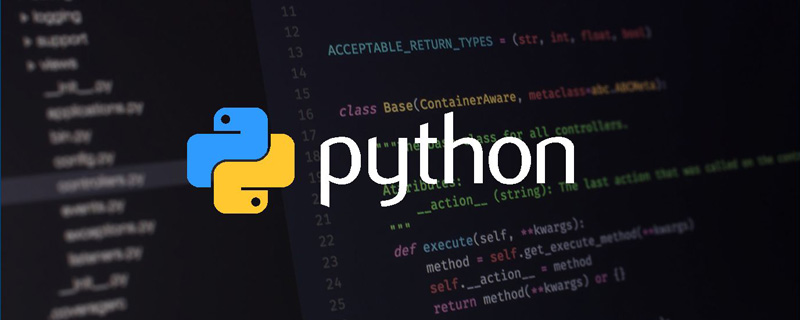
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
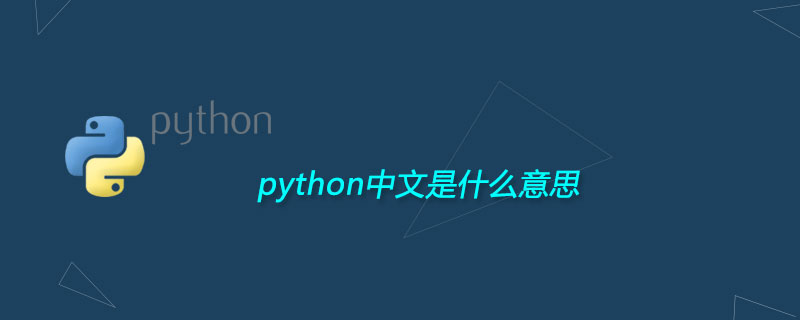
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
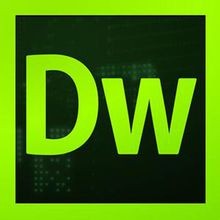
Dreamweaver CS6
Visual web development tools
