


How to solve golang error: cannot refer to unexported name 'x' in package 'y', solution strategy
How to solve golang error: cannot refer to unexported name 'x' in package 'y'
In the process of using golang development, we may encounter this Error: "cannot refer to unexported name 'x' in package 'y'". This error is mainly caused when we access unexported variables or functions.
In golang, the case of the first letter of variable and function names determines their visibility. An identifier is exportable if its first letter is an uppercase letter; if its first letter is a lowercase letter, it is not exportable. Non-exportable variables or functions can only be accessed within the same package and cannot be directly accessed by other packages. When we try to access unexported variables or functions in other packages, the above error will occur.
So, how to solve this problem? The following are several solution strategies:
- Change the variable or function to export: If we need to access a variable or function in other packages, then we can change its first letter to a capital letter , so it can be exported. For example, if we have a variable named "x" that is not accessible outside the package, we can change it to "X".
Code example:
package y var x string // 不可导出的变量 func foo() { // 不可导出的函数 }
Modified code:
package y var X string // 可导出的变量 func Foo() { // 可导出的函数 }
- Access within the same package: If we cannot change the variable or function If it is exported but needs to be used in other packages, you can indirectly access this variable or function by providing a function inside the same package. In this way, other packages can get the required results by calling this function.
Code example:
package y var x string // 不可导出的变量 func getX() string { return x }
Called in other packages:
package main import ( "fmt" "package/y" ) func main() { x := y.getX() fmt.Println(x) }
- Use interfaces to hide implementation details: If there are some private types in a package ( The first letter is lowercase), but we hope that other packages can only call public methods when using them. We can encapsulate these private types as interfaces and expose this interface in a public way. In this way, other packages can only access these types through the interface, but cannot directly access the private methods or properties of their implementation objects.
Code example:
package y type privateType struct { data int } func (p *privateType) privateMethod() { fmt.Println(p.data) } type PublicInterface interface { PublicMethod() } func CreatePrivateType() PublicInterface { return &privateType{} } func (p *privateType) PublicMethod() { p.privateMethod() }
Used in other packages:
package main import ( "package/y" ) func main() { p := y.CreatePrivateType() p.PublicMethod() // 可以调用 p.privateMethod() // 无法调用,报错 }
Through the above solutions, we can solve the golang error: "cannot refer to unexported name 'x' in package 'y'". Choosing the appropriate strategy according to the specific situation can make our code more readable, maintainable, and improve code reusability.
The above is the detailed content of How to solve golang error: cannot refer to unexported name 'x' in package 'y', solution strategy. For more information, please follow other related articles on the PHP Chinese website!
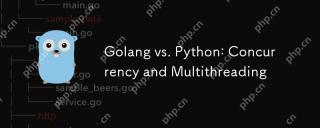
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
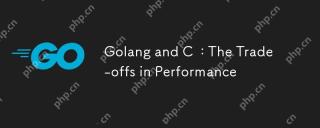
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
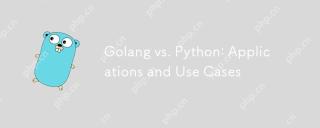
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
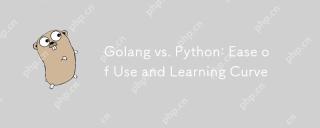
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
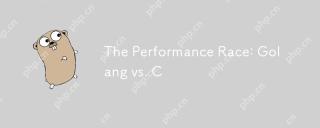
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
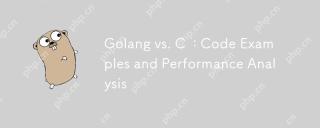
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
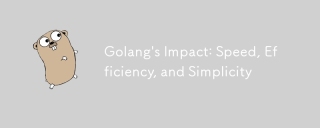
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor
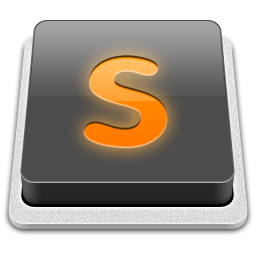
SublimeText3 Mac version
God-level code editing software (SublimeText3)
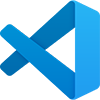
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft