


Solutions to solve Java database operation exceptions (DatabaseOperationException)
Introduction:
When developing Java applications, database operations are one of the common tasks. However, when dealing with database operations, we often encounter some exceptions, one of which is DatabaseOperationException (database operation exception). This article will introduce common causes of DatabaseOperationException and provide solutions and code samples to help developers better handle these exceptions.
1. Common causes of DatabaseOperationException
- Connection errors: When establishing a connection with the database, errors such as connection timeout, insufficient permissions, or unavailable database server may occur. These errors will Causes abnormal database operation.
- SQL errors: When executing SQL statements, syntax errors, table non-existence or field mismatches may occur. These errors may also cause abnormal database operations.
- Database transaction error: When using database transactions, if the transaction commit and rollback operations are not managed correctly, database operation exceptions will occur.
2. Solution
- Solution to connection error
To solve the database operation exception caused by connection error, you can take the following measures:
(1) Confirm whether the database server is available: Make sure the database server is running normally and check whether the network connection is normal.
(2) Check the database connection parameters: Check whether the user name, password, URL and other connection parameters used when connecting to the database are correct.
(3) Set an appropriate connection timeout: If a connection timeout occurs when connecting to the database, it can be solved by setting an appropriate connection timeout.
(4) Use connection pool: Using connection pool can better manage database connections and improve the performance and stability of database operations.
- Solutions to SQL errors
To solve database operation exceptions caused by SQL errors, you can take the following measures:
(1) Check the syntax of the SQL statement: Carefully check whether there are syntax errors in the SQL statement. You can view specific error information by using database debugging tools or log output.
(2) Check whether the tables and fields exist: Confirm whether the tables and fields involved in the SQL statement exist. This can be verified by querying the metadata information of the database.
(3) Parameter binding and escaping: When using dynamic parameters, ensure that parameter binding and parameter escaping are performed correctly to prevent security issues such as SQL injection.
- Solutions to database transaction errors
To solve database operation exceptions caused by database transaction errors, you can take the following measures:
(1) Manage transactions correctly Commit and rollback operations: When performing database transaction operations, ensure that the transaction is committed after the operation is successful and rolled back when the operation fails to avoid the problem of abnormal situations that cause the transaction to fail to end correctly.
(2) Reasonably set the transaction isolation level: According to actual needs, set the transaction isolation level reasonably to ensure data consistency and concurrency.
(3) Use exception handling mechanism: When handling database operation exceptions, you can use try-catch blocks to capture exceptions and perform transaction rollback operations in the exception handling code.
3. Code Example
The following is a simple Java code example that demonstrates how to handle DatabaseOperationException and take appropriate solutions:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class DatabaseUtils { private static final String DB_URL = "jdbc:mysql://localhost:3306/mydb"; private static final String DB_USER = "root"; private static final String DB_PASSWORD = "password"; public static Connection getConnection() throws DatabaseOperationException { try { Class.forName("com.mysql.jdbc.Driver"); return DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD); } catch (ClassNotFoundException e) { throw new DatabaseOperationException("Failed to load database driver", e); } catch (SQLException e) { throw new DatabaseOperationException("Failed to establish database connection", e); } } public static void main(String[] args) { try { Connection connection = DatabaseUtils.getConnection(); // 执行数据库操作... } catch (DatabaseOperationException e) { e.printStackTrace(); // 处理数据库操作异常... } } }
In the above code example, we use the database The connection pool obtains the database connection and uses try-catch blocks to catch exceptions that may occur. In the getConnection()
method, by loading the database driver and establishing a database connection, if a ClassNotFoundException or SQLException occurs, a DatabaseOperationException will be thrown.
Conclusion:
Through the introduction of this article, we have learned about the common causes of DatabaseOperationException and provided solutions and code examples. Properly handling these exceptions can help improve the stability and reliability of Java applications and enable us to perform better database operations.
Reference materials:
- The Java Tutorials - Lesson: JDBC Basics (https://docs.oracle.com/javase/tutorial/jdbc/basics/index.html)
The above is the detailed content of Solutions to solve Java database operation exceptions (DatabaseOperationException). For more information, please follow other related articles on the PHP Chinese website!
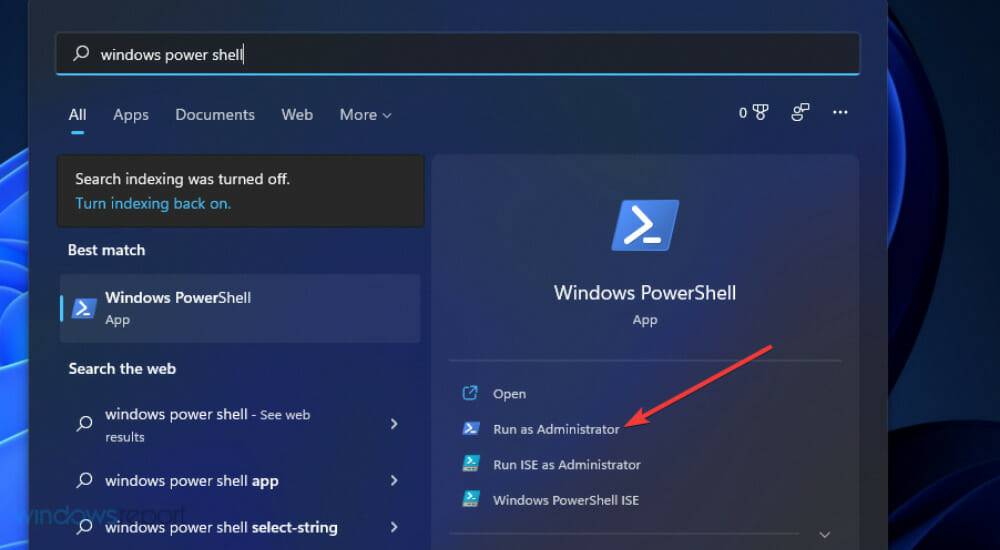
Sysprep问题可能出现在Windows11、10和8平台上。出现该问题时,Sysprep命令不会按预期运行和验证安装。如果您需要修复Sysprep问题,请查看下面的Windows11/10解决方案。Sysprep错误是如何在Windows中出现的?Sysprep无法验证您的Windows安装错误自Windows8以来一直存在。该问题通常是由于用户安装的UWP应用程序而出现的。许多用户已确认他们通过卸载从MSStore安装的某些UWP应用程序解决了此问题。如果缺少应该与Windows一起预安装
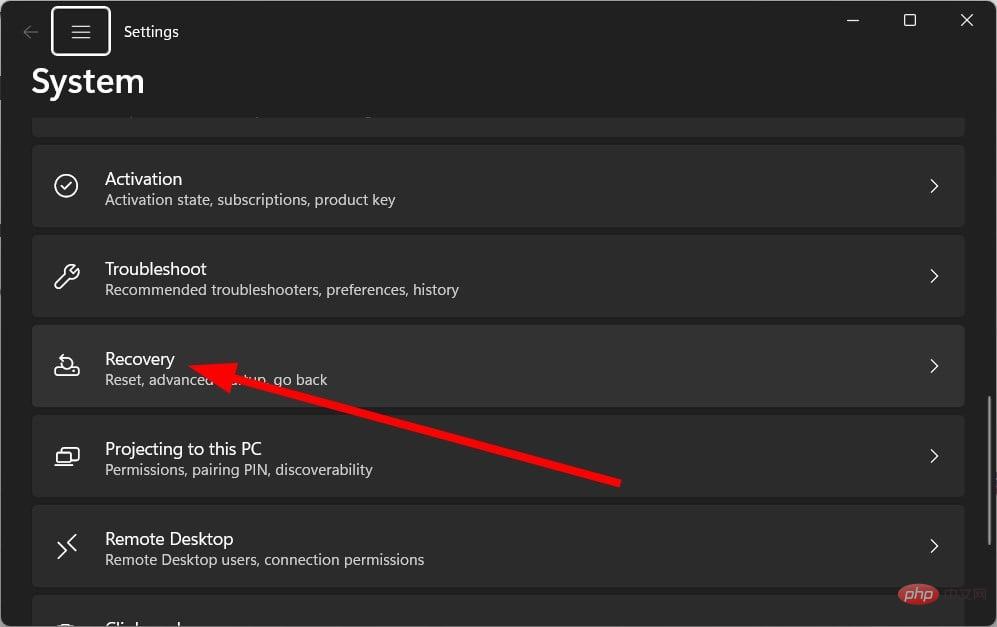
您将找到多个用户报告,确认NETHELPMSG2221错误代码。当您的帐户不再是管理员时,就会显示此信息。根据用户的说法,他们的帐户自动被撤销了管理员权限。如果您也遇到此问题,我们建议您应用指南中的解决方案并修复NETHELPMSG2221错误。您可以通过多种方式将管理员权限恢复到您的帐户。让我们直接进入它们。什么是NETHELPMSG2221错误?当您不是PC的管理员时,无法使用提升的程序。因此,例如,你将无法在电脑上运行命令提示符、WindowsPowerShell或任
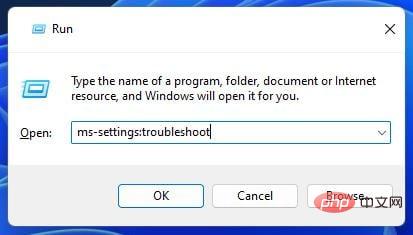
什么原因导致WindowsUpdate错误0x8024800c?导致WindowsUpdate错误的原因0x8024800c尚不完全清楚。但是,此问题可能与其他更新错误具有类似的原因。以下是一些潜在的0x8024800c错误原因:损坏的系统文件–某些系统文件需要修复。不同步的软件分发缓存–软件分发数据存储不同步,这意味着此错误是超时问题(它有一个WU_E_DS_LOCKTIMEOUTEXPIRED结果字符串)。损坏的WindowsUpdate组件-错误0x8024800c是由错误的Win
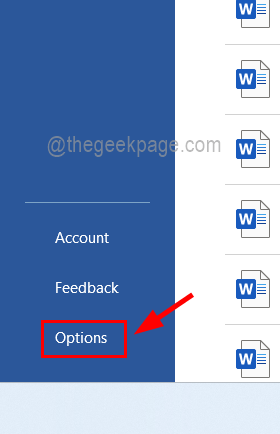
MSOffice产品是任何Windows系统上用于创建Word、Excel表格等文档的应用程序的绝佳选择。但是您需要从Microsoft购买Office产品的有效许可证,并且必须激活它才能使其有效工作.最近,许多Windows用户报告说,每当他们启动任何Office产品(如Word、Excel等)时,他们都会收到一条警告消息,上面写着“您的Office许可证存在问题,并要求用户获取正版Office许可证”。一些用户不假思索,就去微软购买了Office产品的许可证
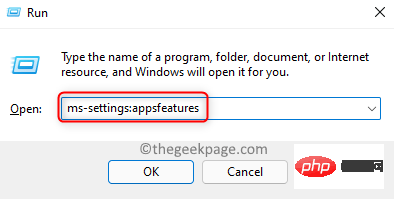
许多用户在系统变慢时报告任务管理器中存在WWAHost.exe进程。WWAHost.exe进程会占用大量系统资源,例如内存、CPU或磁盘,进而降低PC的速度。因此,每当您发现您的系统与以前相比变得缓慢时,请打开任务管理器,您会在那里找到这个WWAHost.exe进程。通常,已观察到启动任何应用程序(如Mail应用程序)会启动WWAHost.exe进程,或者它可能会自行开始执行,而无需在您的WindowsPC上进行任何外部输入。此进程是安全有效的Microsoft程序,是Wi
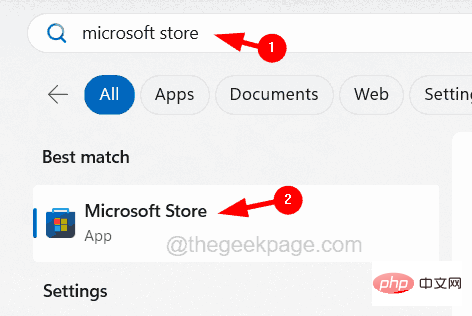
大多数人作为备份实践将他们的文件从iPhone传输到PC/Mac,以防由于某些明显的原因而丢失。为此,他们必须通过避雷线将iPhone连接到PC/Mac。许多iPhone用户在尝试将iPhone连接到计算机以在它们之间同步文件时遇到错误1667。此错误背后有相当潜在的原因,可能是计算机或iPhone中的内部故障,闪电电缆损坏或损坏,用于同步文件的过时的iTunes应用程序,防病毒软件产生问题,不更新计算机的操作系统等。在这篇文章中,我们将向您解释如何使用以下给定的解决方案轻松有效地解决此错误。初
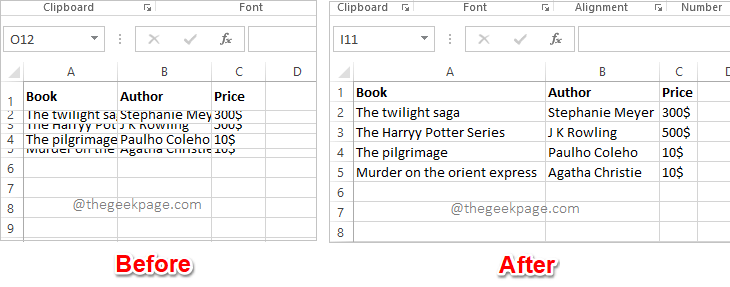
你有一个紧迫的截止日期,你即将提交你的工作,那时你注意到你的Excel工作表不整洁。行和列的高度和宽度不同,大部分数据是重叠的,无法完美查看数据。根据内容手动调整行和列的高度和宽度确实会花费大量时间,当然不建议这样做。顺便说一句,当你可以通过一些简单的点击或按键来自动化整个事情时,你为什么还要考虑手动做呢?在本文中,我们详细解释了如何通过以下3种不同的解决方案轻松地在Excel工作表中自动调整行高或列宽。从现在开始,您可以选择自己喜欢的解决方案并成为Excel任务的高手!解决方案1:通过
![修复:Windows 11 不关闭显示 [6 个简单的解决方案]](https://img.php.cn/upload/article/000/887/227/168171288789845.jpg)
Windows11可以选择在一段时间不活动后关闭显示器。当用户离开计算机并且不手动使其进入睡眠状态时,此功能可以节省电量。用户报告了即使在设置的持续时间之后他们的显示器也没有关闭的问题。幸运的是,有一些简单的解决方案可以解决这个问题。如果您的Windows11显示屏在设置时间后未关闭,则可能是由于应用程序或外部设备有问题。继续阅读本文以找到解决方案。如何调整睡眠和屏幕设置?单击开始并转到设置(或按Windows+I)。在系统下转到电源和电池。在屏幕和睡眠下,调整您希望显示器进入睡眠或关闭的时


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
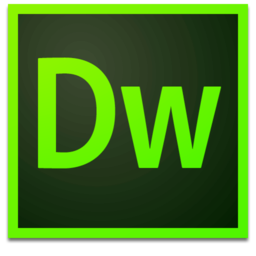
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
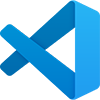
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
