How to use PHP to develop online question and answer function
How to use PHP to develop online Q&A function
Introduction:
With the development of the Internet, online Q&A platforms have gradually become the main way for people to obtain knowledge and information. one. In this process, PHP, as a powerful back-end programming language, is widely used in the development of various websites and applications. This article will introduce how to use PHP to develop a simple online question and answer function, including user registration, login, question, answer and other functions, and provide corresponding code examples.
1. Implementation of user registration and login functions
- Create database table
First, we need to create a user table to store user registration information. You can use PHP's MySQLi or PDO extension to connect to the database and execute SQL statements to create user tables. The sample code is as follows:
$servername = "localhost"; $username = "username"; $password = "password"; $dbname = "database"; $conn = new mysqli($servername, $username, $password, $dbname); $sql = "CREATE TABLE users ( id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY, username VARCHAR(30) NOT NULL, password VARCHAR(30) NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP )"; if ($conn->query($sql) === TRUE) { echo "Table users created successfully"; } else { echo "Error creating table: " . $conn->error; } $conn->close();
- Implementation of registration function
The user registration function requires a registration page and a registration handler. The registration page contains input boxes for username and password, as well as a submit button. The registration handler accepts form data, validates and processes it, and inserts user information into the database. The sample code is as follows:
Registration page (register.php):
<form action="register_process.php" method="POST"> <input type="text" name="username" placeholder="用户名" required><br> <input type="password" name="password" placeholder="密码" required><br> <button type="submit">注册</button> </form>
Registration handler (register_process.php):
$servername = "localhost"; $username = "username"; $password = "password"; $dbname = "database"; $conn = new mysqli($servername, $username, $password, $dbname); $username = $_POST['username']; $password = $_POST['password']; $sql = "INSERT INTO users (username, password) VALUES ('$username', '$password')"; if ($conn->query($sql) === TRUE) { echo "注册成功"; } else { echo "Error: " . $sql . "<br>" . $conn->error; } $conn->close();
- Login function Implementing the
user login function requires a login page and a login handler. The login page contains input boxes for username and password, as well as a submit button. The login handler accepts form data, authenticates against user information in the database, and creates a login session. The sample code is as follows:
Login page (login.php):
<form action="login_process.php" method="POST"> <input type="text" name="username" placeholder="用户名" required><br> <input type="password" name="password" placeholder="密码" required><br> <button type="submit">登录</button> </form>
Login handler (login_process.php):
session_start(); $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "database"; $conn = new mysqli($servername, $username, $password, $dbname); $username = $_POST['username']; $password = $_POST['password']; $sql = "SELECT * FROM users WHERE username='$username' AND password='$password'"; $result = $conn->query($sql); if ($result->num_rows == 1) { $_SESSION['username'] = $username; echo "登录成功"; } else { echo "登录失败"; } $conn->close();
2. Question and answer function Implementation
- Creating question and answer tables
In order to implement the question and answer function, we need to create a question table and an answer table. Data tables can be created using the same method. The sample code is as follows:
$servername = "localhost"; $username = "username"; $password = "password"; $dbname = "database"; $conn = new mysqli($servername, $username, $password, $dbname); $sql = "CREATE TABLE questions ( id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY, user_id INT(6) UNSIGNED, title VARCHAR(100) NOT NULL, content TEXT NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP )"; if ($conn->query($sql) === TRUE) { echo "Table questions created successfully"; } else { echo "Error creating table: " . $conn->error; } $sql = "CREATE TABLE answers ( id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY, question_id INT(6) UNSIGNED, user_id INT(6) UNSIGNED, content TEXT NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP )"; if ($conn->query($sql) === TRUE) { echo "Table answers created successfully"; } else { echo "Error creating table: " . $conn->error; } $conn->close();
- Implementation of the question function
The question function requires a question page and a question processing program. The question page contains input boxes for the question title and content, as well as a submit button. The question handler accepts form data, inserts it into the database, and associates the question with the user. The sample code is as follows:
Question page (ask.php):
<form action="ask_process.php" method="POST"> <input type="text" name="title" placeholder="问题标题" required><br> <textarea name="content" placeholder="问题内容" required></textarea><br> <button type="submit">提问</button> </form>
Question handler (ask_process.php):
session_start(); $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "database"; $conn = new mysqli($servername, $username, $password, $dbname); $title = $_POST['title']; $content = $_POST['content']; $user_id = $_SESSION['user_id']; $sql = "INSERT INTO questions (user_id, title, content) VALUES ('$user_id', '$title', '$content')"; if ($conn->query($sql) === TRUE) { echo "提问成功"; } else { echo "Error: " . $sql . "<br>" . $conn->error; } $conn->close();
- Answer function Implementing the
answer function requires an answer page and an answer handler. The answer page contains an input box for the answer content and a submit button. The answer handler accepts the form data, inserts it into the database, and associates the answer with the question and user. The sample code is as follows:
Answer page (answer.php):
<form action="answer_process.php" method="POST"> <textarea name="content" placeholder="回答内容" required></textarea><br> <button type="submit">回答</button> </form>
Answer handler (answer_process.php):
session_start(); $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "database"; $conn = new mysqli($servername, $username, $password, $dbname); $content = $_POST['content']; $question_id = $_POST['question_id']; $user_id = $_SESSION['user_id']; $sql = "INSERT INTO answers (question_id, user_id, content) VALUES ('$question_id', '$user_id', '$content')"; if ($conn->query($sql) === TRUE) { echo "回答成功"; } else { echo "Error: " . $sql . "<br>" . $conn->error; } $conn->close();
Conclusion:
Passed In the above steps, we have completed the development of a basic online question and answer function. Users can register, log in, ask and answer questions. Of course, this is just a simple example, and more functions and security measures may need to be added in actual applications. I hope this article can be helpful to developing online Q&A functions using PHP. Thank you for reading.
The above is the detailed content of How to use PHP to develop online question and answer function. For more information, please follow other related articles on the PHP Chinese website!
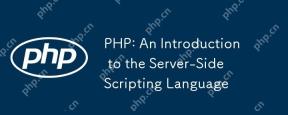
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
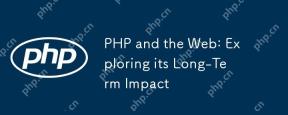
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
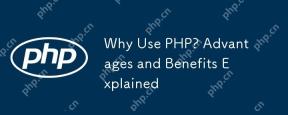
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
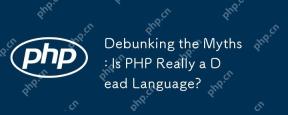
PHP is not dead. 1) The PHP community actively solves performance and security issues, and PHP7.x improves performance. 2) PHP is suitable for modern web development and is widely used in large websites. 3) PHP is easy to learn and the server performs well, but the type system is not as strict as static languages. 4) PHP is still important in the fields of content management and e-commerce, and the ecosystem continues to evolve. 5) Optimize performance through OPcache and APC, and use OOP and design patterns to improve code quality.
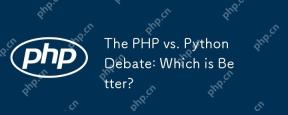
PHP and Python have their own advantages and disadvantages, and the choice depends on the project requirements. 1) PHP is suitable for web development, easy to learn, rich community resources, but the syntax is not modern enough, and performance and security need to be paid attention to. 2) Python is suitable for data science and machine learning, with concise syntax and easy to learn, but there are bottlenecks in execution speed and memory management.
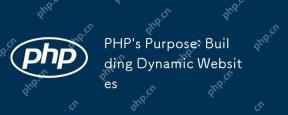
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
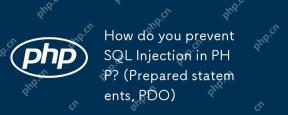
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
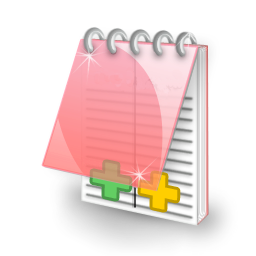
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
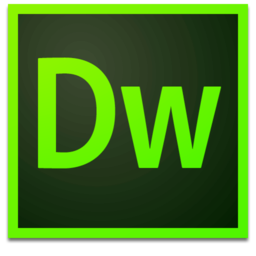
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor