In Python, dictionaries are like maps in C and Java. Like a Map dictionary, it consists of two parts: keys and values. Dictionaries are dynamic, you can add more keys and values after creating the dictionary, and you can also delete keys and values from the dictionary. You can add another dictionary to the currently created dictionary. You can also add lists to dictionaries and dictionaries to lists.
In a dictionary, you can access elements by their respective keys.
Dictionary = { 1: "Apple", 2: "Ball", 3: "Caterpillar", 4: "Doctor", 5: "Elephant" }
Here, dictionary 1, 2, 3... represent keys, and "Apple", "Ball", "Caterpillar"... represent values.
Dictionary = { Key: "Value", Key : "Value", . . . . . Key : "Value" }
Access elements
print(dictionary[1]) #print element whose key value is 1 i.e. “Apple” print(dictionary[4]) # print element whose key value is 4 “Doctor”
Insert and update elements in the dictionary
Dictionary[6] = "Flesh" # inserting element with key value 6 at last in dictionary Dictionary[3] = "Cat" # element with key value 3 is update with value “Cat”
Delete elements in the dictionary
Dictionary.pop(3) # Delete element with key value 3 del Dictionary[4] # delete element with key value 4 Dictionary.popitem() # delete last inserted element in dictionary del Dictionary # this will delete whole dictionary
Built-in functions of dictionary in Python
-
Dictionary_2 = Dictionary.copy()
This copy function will copy all values of the dictionary to Dictionary_2
-
Dictionary.clear()
clear() function will clear the entire dictionary.
-
Dictionary.get(2)
The get() function will return the value of key 2.
-
Dictionary.values()
This function will return all values of the dictionary.
-
Dictionary.update({5:”Ears”})
This function will update the value of the given key
The above is the detailed content of How is a dictionary implemented in Python?. For more information, please follow other related articles on the PHP Chinese website!
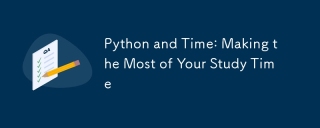
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
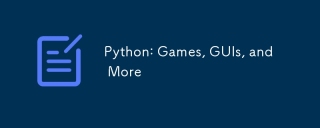
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
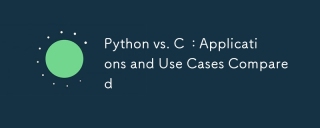
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
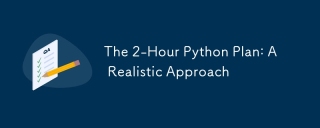
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
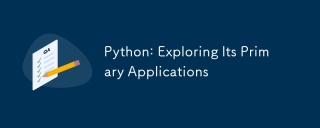
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
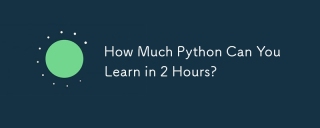
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
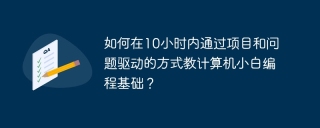
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
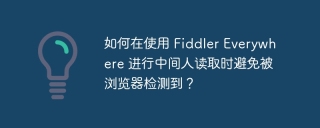
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
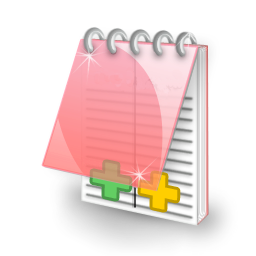
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
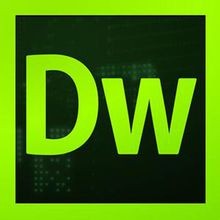
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.