How to use Python to track shapes in pictures
Introduction:
Image processing is an important part of the field of computer vision, and tracking specific shapes in images Tracking is one of the common tasks. This article will introduce how to use Python and the OpenCV library to track shapes in pictures, and provide corresponding code examples.
1. Preparation:
Before starting to write code, we need to install the Python and OpenCV libraries, and prepare a picture containing the target shape as input. First, make sure you have Python installed. You can download and install the version suitable for your operating system from the official Python website. Next, we need to install the OpenCV library using the pip command. Open the terminal (or command line) and enter the following command to install OpenCV:
pip install opencv-python
2. Import the library and read the image:
Before formally writing the code, we First you need to import the corresponding library. Python provides the import statement to import the libraries you need to use. In this task, we need to import the cv2 library (which is the Python interface to the OpenCV library). In addition, we also need to import the numpy library to support array operations. Here is the code to import the required libraries:
import cv2
import numpy as np
Next, we need to read the image and convert it to a grayscale image. Grayscale images are easier to process and can reduce the amount of calculations. Considering that future work may modify the original image, we can use a copy to save the grayscale image. Here is the code to read the image and convert it to grayscale:
image = cv2.imread("input.jpg")
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
3. Shape detection and tracking:
Before performing shape tracking, we need to perform shape detection first. OpenCV provides a function cv2.findContours() for finding contours in images. This function accepts a binarized image as input and returns a list of all contours in the image. Here is a code example for shape detection:
ret, thresh = cv2.threshold(gray, 127, 255, 0)
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2. CHAIN_APPROX_SIMPLE)
Next, we can use the cv2.approxPolyDP() function to approximate the contour and make it smoother. The degree of approximation can be changed by adjusting the value of epsilon, the second parameter of this function. Small epsilon values will produce more accurate approximations, while large epsilon values will produce rougher approximations. Here is a code example for shape approximation:
epsilon = 0.04 * cv2.arcLength(contour, True)
approx = cv2.approxPolyDP(contour, epsilon, True)
Finally, We can use the cv2.drawContours() function to draw the detected shapes onto the original image. The following is a code example for drawing contours:
cv2.drawContours(image, [approx], 0, (0, 255, 0), 2)
Code explanation:
Above code , ret is a Boolean value used to indicate whether the thresholding operation was successful. thresh is a thresholded image used for contour detection. contours is a list containing all detected contours. hierarchy is the hierarchical information of the outline. epsilon is a parameter used to approximate the contour. approx is the approximated contour. Finally, the cv2.drawContours() function is used to draw contours.
4. Display and results:
After completing the shape tracking, we can display the result image and save it as a new file. The following is a code example for displaying and saving results:
cv2.imshow("Result", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
cv2 .imwrite("output.jpg", image)
Code explanation:
In the above code, the cv2.imshow() function is used to display the result image. cv2.waitKey(0) is used to wait for the user to press any key before closing the image window. The cv2.destroyAllWindows() function is used to close all open windows. Finally, the cv2.imwrite() function is used to save the resulting image.
Summary:
This article introduces how to use Python and the OpenCV library to track shapes in pictures. We first learned about the preparation, then imported the required libraries and read the images. Next, we performed shape detection and tracking to track the target shape by approximating contours and drawing contours. Finally, we display the resulting image and save the tracking results.
Here is the complete code example:
import cv2 import numpy as np image = cv2.imread("input.jpg") gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) ret, thresh = cv2.threshold(gray, 127, 255, 0) contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) for contour in contours: epsilon = 0.04 * cv2.arcLength(contour, True) approx = cv2.approxPolyDP(contour, epsilon, True) cv2.drawContours(image, [approx], 0, (0, 255, 0), 2) cv2.imshow("Result", image) cv2.waitKey(0) cv2.destroyAllWindows() cv2.imwrite("output.jpg", image)
I hope this article can help readers understand how to use Python and the OpenCV library to track shapes in pictures. By learning and applying the code examples provided in this article, readers can easily perform shape tracking tasks and play a role in image processing applications.
The above is the detailed content of How to use Python to trace shapes on pictures. For more information, please follow other related articles on the PHP Chinese website!
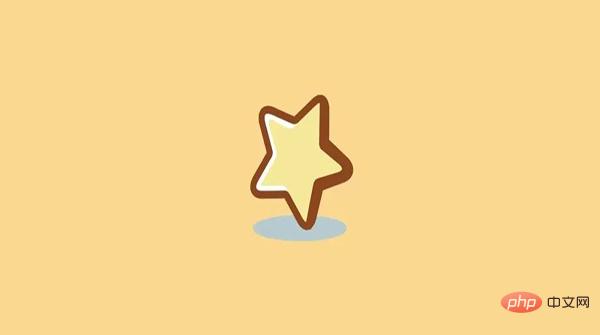
网上下载的 pdf 学习资料有一些会带有水印,非常影响阅读。比如下面的图片就是在 pdf 文件上截取出来的,今天我们就来用Python解决这个问题。安装模块PIL:Python Imaging Library 是 python 上非常强大的图像处理标准库,但是只能支持 python 2.7,于是就有志愿者在 PIL 的基础上创建了支持 python 3的 pillow,并加入了一些新的特性。pip install pillow pymupdf 可以用 python 访问扩展名为*.pdf、
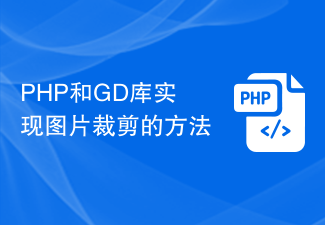
PHP和GD库实现图片裁剪的方法概述:图片裁剪是网页开发中常见的需求之一,它可以用于调整图片的尺寸,剪裁不需要的部分,以适应不同的页面布局和展示需求。在PHP开发中,我们可以借助GD库来实现图片裁剪的功能。GD库是一个强大的图形库,可提供一系列函数来处理和操控图像。代码示例:下面我们将详细介绍如何使用PHP和GD库来实现图片裁剪。首先,确保你的PHP环境已经
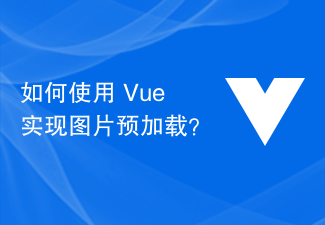
在网页开发中,图片预载是一种常见的技术,可以提升用户的体验感。当用户浏览网页时,图片可以提前下载并加载,减少图片加载时的等待时间。在Vue框架中,我们可以通过一些简单的方法来实现图片预载。本文将介绍Vue中的图片预载技术,包括预载的原理、实现的方法和使用注意事项。一、预载的原理首先,我们来了解一下图片预载的原理。传统的图片加载方式是等到图片全部下载完成才显示
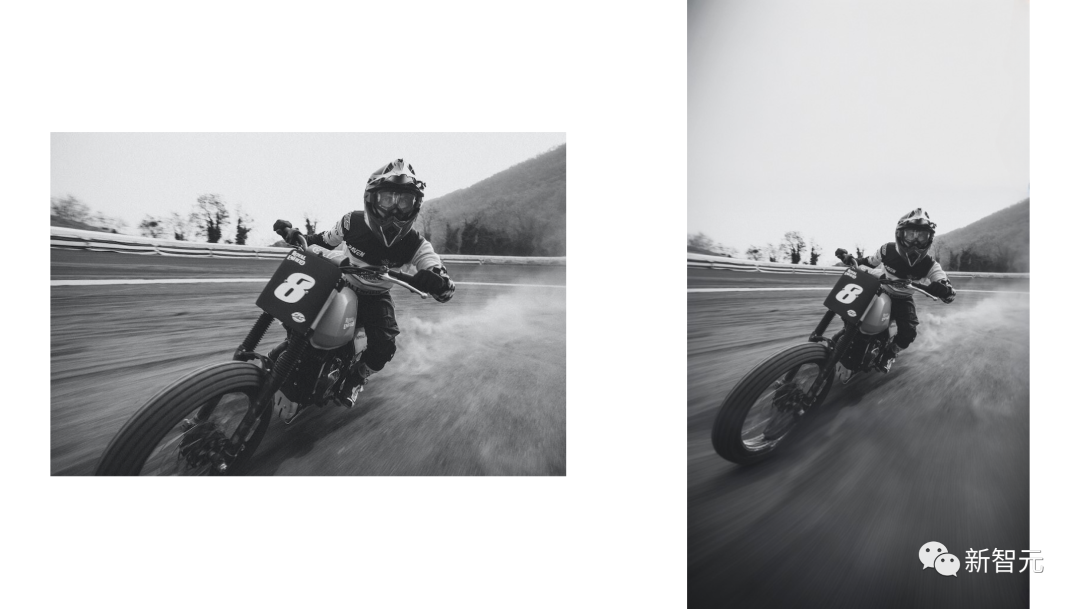
此前,PS的重建图像功能就让人无比振奋,让无数人惊呼今天,StabilityAI又放大招了。它联合Clipdrop推出了UncropClipdrop——一个终极图像比例编辑器。从Uncrop这个名字上,我们就能看出它的用途。它是一个AI生成的「外画」工具,通过创建扩展背景,这个工具可以补充任何现有照片或图像,来更改任何图像的比例。敲黑板:通过Clipdrop网站,就可以免费试用这个工具了,无需登录!比例任意调,满意为止Uncrop基于StabilityAI的文本到图像模型StableDiffus
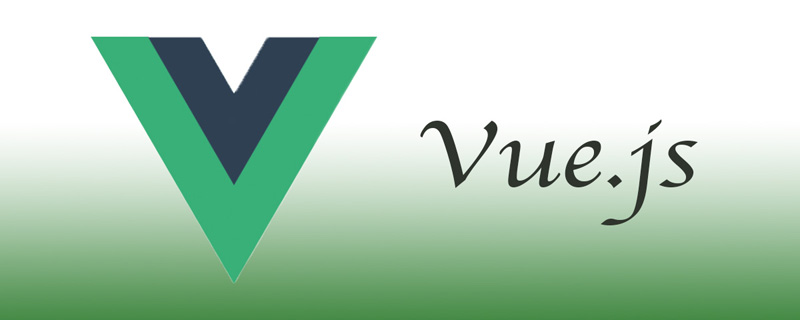
vue报错找不到图片的解决办法:1、修改配置文件,将绝对路径改为相对路径;2、将图片作为模块加载进去,并将图片放到static目录下;3、将imageUrls引入响应的vue文件中,解析引用即可。
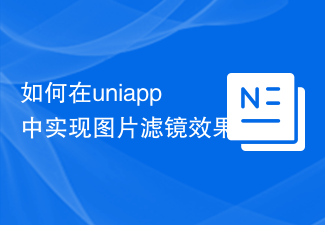
如何在uniapp中实现图片滤镜效果在移动应用开发中,图片滤镜效果是一种常见且受用户喜爱的功能之一。而在uniapp中,实现图片滤镜效果也并不复杂。本文将为大家介绍如何通过uniapp实现图片滤镜效果,并附上相关代码示例。导入图片首先,我们需要在uniapp项目中导入一张图片,以供后续滤镜效果的处理。可以在项目的资源文件夹中放置一张命名为“filter.jp
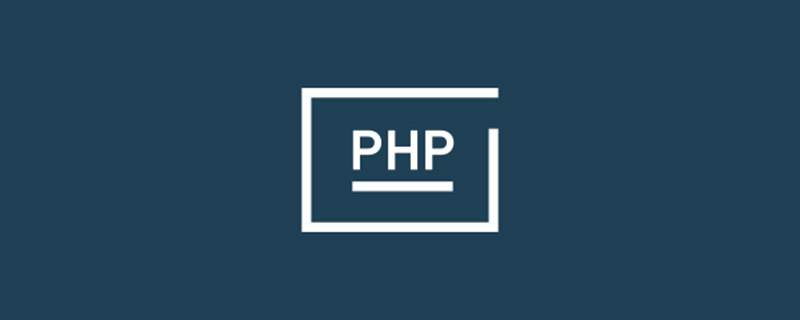
php写图片不显示不出来的解决办法:1、找到并打开php.ini文件;2、找到“extension=php_gd2.dll”,并将前面的分号去掉;3、重新启动服务器;4、在绘图前清一下缓存即可。
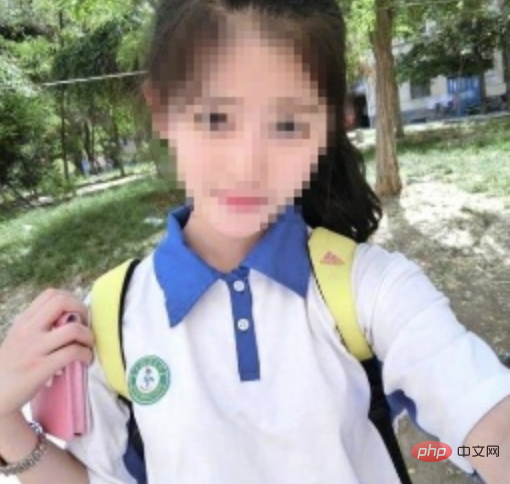
哈喽,大家好。你有没有想过用 AI 技术去除马赛克?仔细想想这个问题还挺难的,因为我们之前使用的 AI 技术,不管是人脸识别还是OCR识别,起码人工能识别出来。但如果给你一张打上马赛克的图片,你能把它复原吗?显然是很难的。如果人都无法复原,又怎能教会计算机去复原呢?还记得前几天我写的一篇《用AI生成头像》文章吗。在那篇文章中,我们训练了一个DCGAN模型,它可以从任意随机数生成一个图像。随机数作为像素生成的噪声图模型从随机数生成正常头像DCGAN包含生成器模型和判别器模型两个模型组成,生成


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
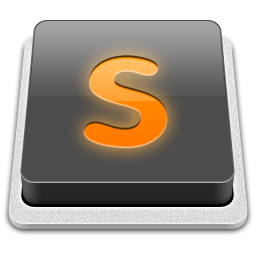
SublimeText3 Mac version
God-level code editing software (SublimeText3)
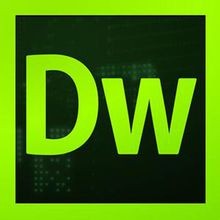
Dreamweaver CS6
Visual web development tools
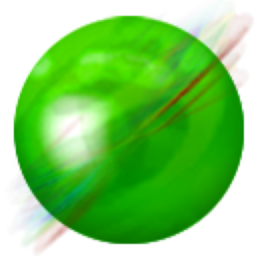
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
