golang error: missing return at end of function, solution
When using Golang to write code, we sometimes encounter a compilation error, the error message is " missing return at end of function". This error means that a return statement is missing from a function. This article describes common causes of this error and provides workarounds and code examples.
There are many reasons for this error. Here are some common situations:
- The return type is not specified when the function is declared.
This is the most common reason for this error. one of the reasons. In Golang, the return type needs to be explicitly specified when a function is declared. If you do not specify a return type when declaring a function, the compiler will not be able to determine the return type of the function and will report an error. The solution is to specify the return type explicitly when the function is declared.
func myFunction() int { // function body }
- All return statements in the function are surrounded by conditional control structures
In some cases, we may place all return statements of the function in conditional control structures ( such as if statements or for loops). Doing so will cause the compiler to be unable to determine that there will be a return statement on all possible paths, causing an error. The solution is to ensure that there are return statements on all possible paths, or to move all return statements out of the conditional control structure.
func myFunction() int { if condition { return 0 } else { return 1 } }
- Unreachable code appears in a function
When some code in a function can never be reached, the compiler will think that the function is missing a return statement. For example, in an infinite or infinite loop, the compiler will not be able to reach the end of the function. The solution is to add a return statement somewhere where the end of the function can be reached, or to refactor the code to avoid unreachable code.
func myFunction() int { for { // infinite loop } // unreachable code }
- Other reasons
In addition to the above situations, there are some other reasons that may cause this error to occur. For example, there are multiple return statements in the function, but their return types are inconsistent; or the return statements of the function are placed in incorrect locations. The solution is to check the return statements in the function to make sure they comply with the syntax rules and are reachable.
To summarize, when we encounter the "missing return at end of function" error when writing code in Golang, we must first confirm whether the return type is specified when the function is declared; secondly, check the function All return statements to ensure they are reachable and have consistent return types; finally, check whether there is unreachable code in the code.
Sample code:
package main import "fmt" func divide(x, y int) (int, error) { if y == 0 { return 0, fmt.Errorf("divide by zero") } return x / y, nil } func main() { result, err := divide(6, 2) if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Result:", result) } }
In the above sample code, we define a function named "divide" to calculate the quotient of two integers. If the divisor is 0, the function returns 0 and an error. In the main function, we call the divide function and print different information based on the return result.
I hope that through the introduction of this article, you can have a clearer understanding of the "missing return at end of function" error and be able to correctly handle this error when writing Golang code. Remember, good coding habits and care are key to avoiding these types of mistakes.
The above is the detailed content of golang error: missing return at end of function, solution. For more information, please follow other related articles on the PHP Chinese website!
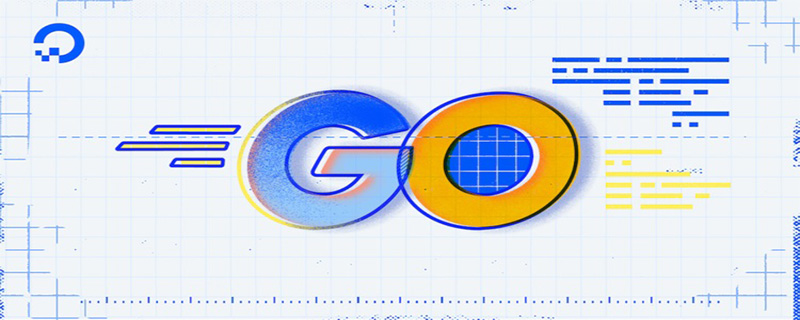
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
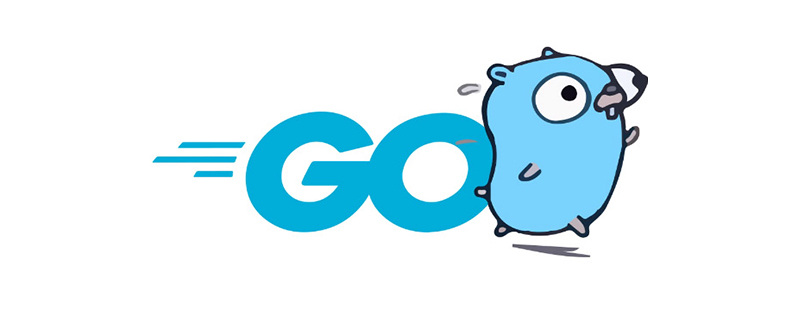
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
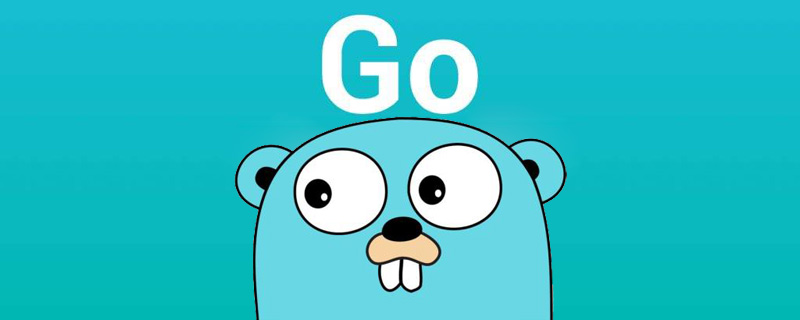
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
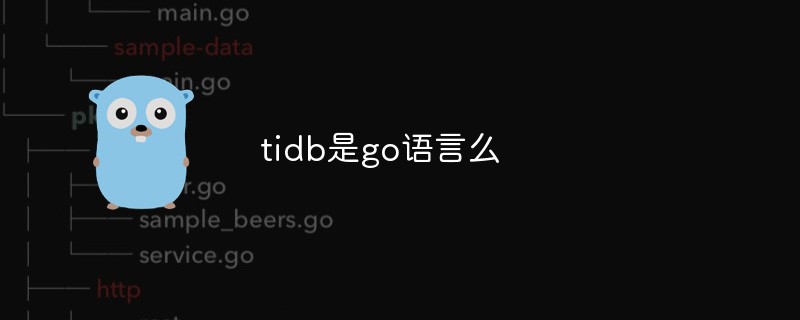
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
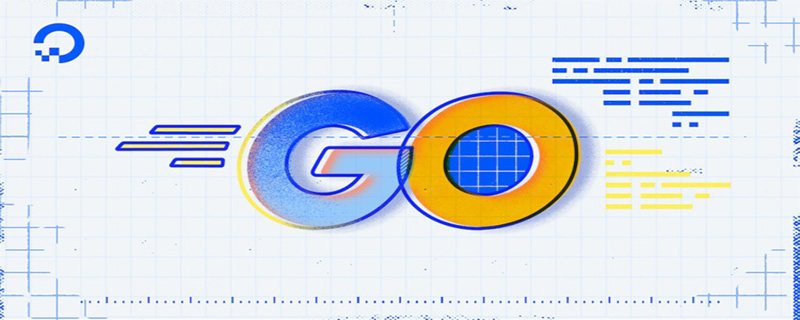
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
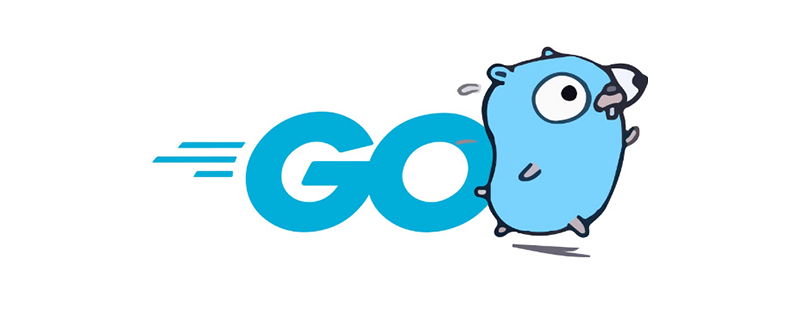
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
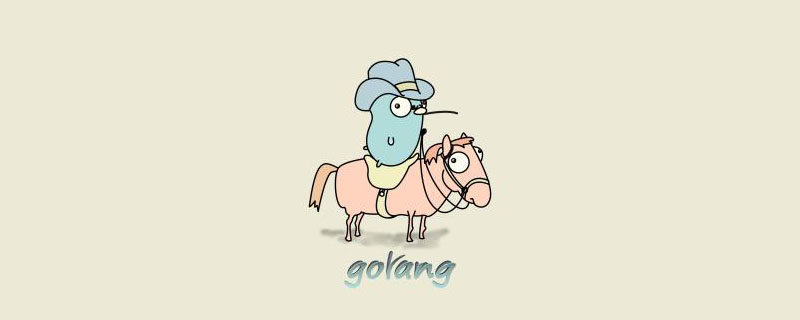
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
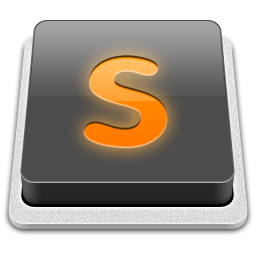
SublimeText3 Mac version
God-level code editing software (SublimeText3)
