How to use Python to draw geometric shapes on pictures
Introduction: Python, as a powerful programming language, can not only perform advanced technologies such as data processing and machine learning, but also Image processing and graphics drawing are also possible. In image processing, it is often necessary to draw various geometric shapes on pictures. This article will introduce how to use Python to draw geometric shapes on pictures.
1. Environment preparation and library installation
Before starting, we first need to install several necessary libraries for Python, mainly including the OpenCV library and the Matplotlib library. You can install it by using the pip command, as shown below:
pip install opencv-python
pip install matplotlib
After the installation is complete, we can start using Python to perform image geometry The shape is drawn.
2. Drawing a Rectangle
Drawing a rectangle is one of the simplest geometric shape drawings and can be achieved through the rectangle function in the OpenCV library. The following is a simple sample code:
import cv2
Read the image
img = cv2.imread('image.jpg')
Draw Rectangle
cv2.rectangle(img, (100, 100), (300, 300), (0, 255, 0), 3)
Display image
cv2 .imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
In the above code, we first read an image through the cv2.imread function , and save it to the img variable. Then we call the cv2.rectangle function to draw a rectangle, where the first parameter is the image variable, the second parameter is the coordinates of the upper left corner of the rectangle, the third parameter is the coordinates of the lower right corner of the rectangle, and the fourth parameter is the color. The fifth parameter is line width. Finally, we display the image through the cv2.imshow function.
3. Draw a circle
Drawing a circle can also be achieved through the OpenCV library, and you can use the circle function. The following is a sample code:
import cv2
Read the image
img = cv2.imread('image.jpg')
Draw a circle
cv2.circle(img, (200, 200), 100, (0, 0, 255), -1)
Show picture
cv2.imshow(' image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
In the above code, we first read an image through the cv2.imread function and Save it to the img variable. Then we call the cv2.circle function to draw a circle, where the first parameter is the image variable, the second parameter is the center coordinate, the third parameter is the radius, the fourth parameter is the color, and the fifth parameter is the line Width, if set to -1, fills the circle. Finally, we display the image through the cv2.imshow function.
4. Drawing lines
Drawing lines is also a common requirement for drawing geometric shapes, which can be achieved using the line function in the OpenCV library. The following is a sample code:
import cv2
Read the image
img = cv2.imread('image.jpg')
Draw the line
cv2.line(img, (100, 100), (300, 300), (255, 0, 0), 5)
Show picture
cv2.imshow ('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
In the above code, we also first read an image through the cv2.imread function, and save it into img variable. Then we call the cv2.line function to draw a straight line, where the first parameter is the picture variable, the second parameter is the starting point coordinates of the line, the third parameter is the end point coordinates of the line, and the fourth parameter is the color. The fifth parameter is line width. Finally, we display the image through the cv2.imshow function.
5. Draw polygons
You can also use the line function in the OpenCV library to draw polygons. You only need to pass in the coordinates of multiple points. The following is a sample code:
import cv2
Read the image
img = cv2.imread('image.jpg')
Define the polygon Several vertices
pts = np.array([[200, 50], [300, 100], [300, 200], [100, 200], [100, 100]], np.int32 )
Draw polygon
cv2.polylines(img, [pts], True, (255, 0, 255), 3)
Display image
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
In the above code, we also first read through the cv2.imread function An image and save it to the img variable. Then we define an array pts, which contains the coordinates of several vertices of the polygon. Finally, we call the cv2.polylines function to draw polygons, where the first parameter is the image variable, the second parameter is the array of vertices, the third parameter indicates whether to close the polygon, the fourth parameter is the color, and the fifth parameter is the line width. Finally, we display the image through the cv2.imshow function.
Conclusion: This article briefly introduces how to use Python to draw geometric shapes on pictures, and provides sample code for rectangles, circles, lines and polygons. By learning these methods, we can better apply Python for image processing and graphics drawing. Hope this article is helpful to everyone.
The above is the detailed content of How to draw geometric shapes on a picture using Python. For more information, please follow other related articles on the PHP Chinese website!
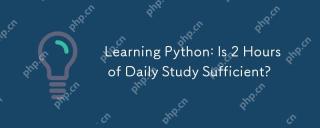
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
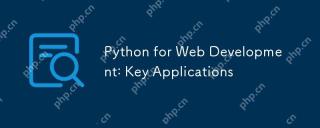
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
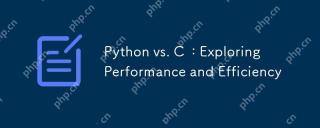
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
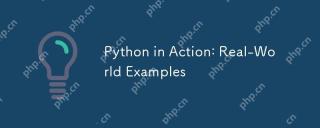
Python's real-world applications include data analytics, web development, artificial intelligence and automation. 1) In data analysis, Python uses Pandas and Matplotlib to process and visualize data. 2) In web development, Django and Flask frameworks simplify the creation of web applications. 3) In the field of artificial intelligence, TensorFlow and PyTorch are used to build and train models. 4) In terms of automation, Python scripts can be used for tasks such as copying files.
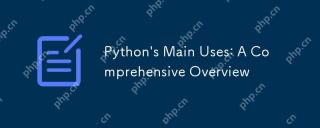
Python is widely used in data science, web development and automation scripting fields. 1) In data science, Python simplifies data processing and analysis through libraries such as NumPy and Pandas. 2) In web development, the Django and Flask frameworks enable developers to quickly build applications. 3) In automated scripts, Python's simplicity and standard library make it ideal.
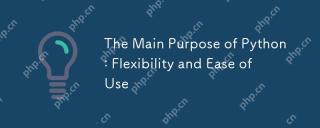
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
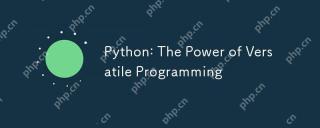
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
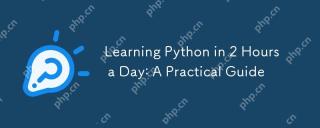
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
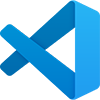
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
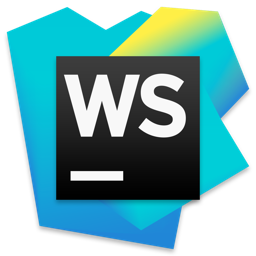
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version