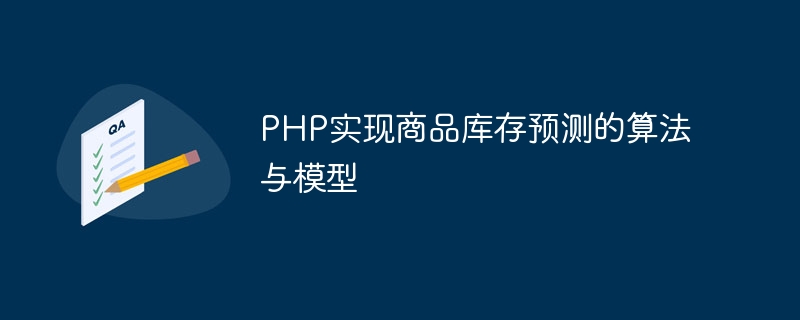
Algorithms and models for PHP to implement commodity inventory forecasting
- Introduction
Commodity inventory forecasting refers to predicting the sales and sales of commodities through algorithms and models. Inventory levels allow supply chain managers to make appropriate decisions, such as purchasing plans and inventory adjustments. In actual business, accurate forecasting of commodity inventory is of great significance to ensure the efficient operation of the supply chain and save costs. This article will introduce how to use PHP to implement commodity inventory forecasting algorithms and models based on historical sales data.
- Data preparation
First, you need to prepare historical sales data as a training set for the model. The data includes the sales quantity of each product and the corresponding date. Data can be obtained from a database or imported from a CSV file. In this article, we will import data from a CSV file.
- Data preprocessing
Before data prediction, the data needs to be preprocessed. First, the date needs to be converted into a timestamp to facilitate subsequent calculations. Secondly, the sales quantity needs to be normalized so that the sales quantity of different commodities can be compared and analyzed. You can use the following code to preprocess the data:
// 读取CSV文件
$data = array_map('str_getcsv', file('sales_data.csv'));
// 定义数组来存储预处理后的数据
$normalizedData = array();
// 对数据进行预处理
foreach ($data as $row) {
$date = strtotime($row[0]);
$quantity = $row[1];
// 归一化处理
$normalizedQuantity = ($quantity - $min) / ($max - $min);
$normalizedData[] = array($date, $normalizedQuantity);
}
- Model training
After the data preprocessing is completed, you need to use historical data to train the model. This article uses a simple linear regression model as an example. The goal of the linear regression model is to predict the target value through known feature values. In this article, the feature value refers to the date and the target value refers to the sales quantity. You can use the following code to train a linear regression model:
// 分离特征值和目标值
$dates = array_column($normalizedData, 0);
$quantities = array_column($normalizedData, 1);
// 使用线性回归模型
$model = new LinearRegression();
$model->train($dates, $quantities);
- Inventory prediction
After the model training is completed, you can use the model to predict future sales to obtain the inventory of the product need. You can use the following code to predict sales in the future:
// 设置预测的时间范围
$startDate = strtotime('2022-01-01');
$endDate = strtotime('2022-12-31');
// 预测销售数量
$predictedQuantities = array();
// 对每个日期进行预测
for ($date = $startDate; $date <= $endDate; $date += 86400) {
$predictedQuantity = $model->predict($date);
// 还原归一化处理
$quantity = $predictedQuantity * ($max - $min) + $min;
$predictedQuantities[] = array(date('Y-m-d', $date), $quantity);
}
- Result display and analysis
Finally, the predicted sales quantity can be displayed and analyzed for supply Chain managers make decisions. The predicted sales quantity can be plotted into a curve chart, or indicators such as total sales volume per month can be calculated. You can use the following code to display the prediction results:
// 绘制曲线图或者计算销售总量等指标
foreach ($predictedQuantities as $row) {
echo $row[0] . ":" . $row[1] . "</br>";
}
Through the above steps, we can use PHP to implement the commodity inventory prediction algorithm and model based on historical sales data. In this way, the inventory demand of goods can be more accurately predicted, so that procurement plans and inventory adjustments can be reasonably arranged, supply chain management efficiency can be improved, and costs can be saved. Of course, in order to better predict inventory demand, more complex models and algorithms can also be used, or combined with other factors, such as promotional activities, weather factors, etc., for predictive analysis.
The above is the detailed content of Algorithms and models for commodity inventory forecasting using PHP. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn