How to solve Java input and output stream exception (IOStreamException)
How to solve Java input and output stream exception (IOStreamException)
Overview:
In Java programming, input and output stream exception (IOStreamException) is a common mistake. It usually occurs while processing files or network connections and can result in data loss or operation failure. In order to solve this problem, we need to correctly handle input and output stream exceptions. This article will explain how to resolve IOStreamException in Java and provide some sample code to help you understand better.
Solution:
The following are some common solutions to avoid or handle IOStreamException.
- Use try-catch statement:
When processing input and output streams, always use try-catch statement to catch possible exceptions. In this way, when an exception occurs, it can be handled in time to avoid program crash.
Sample code:
try { // 打开文件或建立网络连接 InputStream inputStream = new FileInputStream("test.txt"); // 读取数据 int data = inputStream.read(); // 处理数据 // ... // 关闭流 inputStream.close(); } catch (IOException e) { // 处理异常 e.printStackTrace(); }
- Close the stream:
After using the input and output streams, it is very important to close the stream in time. Because if the stream is not closed, it may cause problems such as waste of resources and file locking. Therefore, it is a good practice to close the stream in a finally block.
Sample code:
InputStream inputStream = null; try { inputStream = new FileInputStream("test.txt"); // 读取数据 int data = inputStream.read(); // 处理数据 // ... } catch (IOException e) { // 处理异常 e.printStackTrace(); } finally { // 关闭流 if (inputStream != null) { try { inputStream.close(); } catch (IOException e) { e.printStackTrace(); } } }
- Exception passing:
Sometimes, we cannot handle IOStreamException in the current method, but we can pass the exception to the previous layer call method to process. This keeps the code clear and maintainable.
Sample code:
public void readData() throws IOException { InputStream inputStream = null; try { inputStream = new FileInputStream("test.txt"); // 读取数据 int data = inputStream.read(); // 处理数据 // ... } finally { // 关闭流 if (inputStream != null) { inputStream.close(); } } } public void processFile() { try { readData(); } catch (IOException e) { // 处理异常 e.printStackTrace(); } }
- Using BufferedStream:
Java provides the BufferedInputStream and BufferedOutputStream classes, which can improve IO performance and reduce the occurrence of IOStreamException. Using these classes can avoid IO operations being triggered for each read and write, and instead perform batch reads and writes through the buffer.
Sample code:
try { InputStream inputStream = new BufferedInputStream(new FileInputStream("test.txt")); // 读取数据 int data = inputStream.read(); // 处理数据 // ... inputStream.close(); } catch (IOException e) { // 处理异常 e.printStackTrace(); }
Summary:
In Java programming, handling input and output stream exceptions is an important issue. To avoid data loss and program errors, we need to handle IOStreamException correctly. This article introduces the use of try-catch statements, closing streams, exception delivery, and using BufferedStream to solve this problem, and provides relevant sample code. Through in-depth study and practice, you will better understand and handle IOStreamException, thereby improving the stability and performance of your program.
The above is the detailed content of How to solve Java input and output stream exception (IOStreamException). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
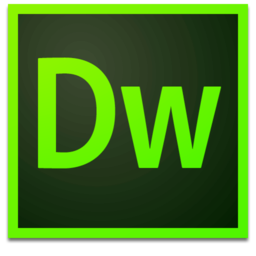
Dreamweaver Mac version
Visual web development tools
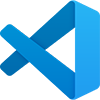
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft