How to use Python to perform noise filtering on pictures
Introduction:
Noise is a common problem in image processing, they can be due to damage to the image sensor or other equipment , Useless information caused by signal interference or transmission errors. Noise can seriously affect image quality and visualization. Noise filtering is a common image processing technique that can reduce or remove noise in images. In this article, we will use Python to demonstrate how to use common noise filtering algorithms to process images.
1. Import the necessary libraries
Before we begin, we need to import some necessary Python libraries in order to perform image processing operations. In this example, we will use the OpenCV library and the NumPy library.
import cv2 import numpy as np
2. Read the image
Next, we need to read the image to be processed. You can use OpenCV's imread
function to read an image file and store it in a variable.
image = cv2.imread('image.jpg')
3. Add noise
In order to demonstrate the noise filtering algorithm, we need to add some noise to the image first. In this example we will use Gaussian noise to add to the image. We can use OpenCV’s randn
function to generate random values from a Gaussian distribution and add them to the pixel values of the image.
# 添加高斯噪声 noise = np.random.randn(*image.shape) * 50 noisy_image = image + noise.astype(np.uint8)
4. Display the original image and the noisy image
Before performing noise filtering, let us first display the original image and the noisy image for comparison.
# 显示原始图像和带噪声的图像 cv2.imshow("Original Image", image) cv2.imshow("Noisy Image", noisy_image) cv2.waitKey(0) cv2.destroyAllWindows()
5. Use noise filtering algorithm
Next, we will use two common noise filtering algorithms: mean filtering and median filtering. These filtering algorithms can remove Gaussian noise from images.
- Mean filter
Mean filter is a simple filtering algorithm that replaces the value of each pixel with the average value of surrounding pixels. In OpenCV, we can use theblur
function to implement mean filtering.
# 均值滤波 kernel_size = 5 blur_image = cv2.blur(noisy_image, (kernel_size, kernel_size))
- Median filtering
Median filtering is a nonlinear filtering algorithm that replaces the value of each pixel with the median value of surrounding pixels. Median filtering usually works better with salt and pepper noise. In OpenCV, we can use themedianBlur
function to implement median filtering.
# 中值滤波 kernel_size = 5 median_image = cv2.medianBlur(noisy_image, kernel_size)
6. Display the filtered image
After noise filtering the image, let us display the filtered image for comparison.
# 显示滤波后的图像 cv2.imshow("Blur Image", blur_image) cv2.imshow("Median Image", median_image) cv2.waitKey(0) cv2.destroyAllWindows()
7. Conclusion
By using Python and the OpenCV library, we can easily perform noise filtering on images. In this article, we demonstrate how to use mean filtering and median filtering, two common noise filtering algorithms, to reduce or remove noise in images. According to actual application requirements, we can adjust the size and parameters of the filter to obtain better filtering effects.
Code example:
import cv2 import numpy as np # 读取图像 image = cv2.imread('image.jpg') # 添加高斯噪声 noise = np.random.randn(*image.shape) * 50 noisy_image = image + noise.astype(np.uint8) # 显示原始图像和带噪声的图像 cv2.imshow("Original Image", image) cv2.imshow("Noisy Image", noisy_image) cv2.waitKey(0) cv2.destroyAllWindows() # 均值滤波 kernel_size = 5 blur_image = cv2.blur(noisy_image, (kernel_size, kernel_size)) # 中值滤波 median_image = cv2.medianBlur(noisy_image, kernel_size) # 显示滤波后的图像 cv2.imshow("Blur Image", blur_image) cv2.imshow("Median Image", median_image) cv2.waitKey(0) cv2.destroyAllWindows()
The above are the steps and code examples for using Python to perform noise filtering on images. I hope this article can help you understand and use noise filtering algorithms to improve image processing results.
The above is the detailed content of How to use Python to perform noise filtering on images. For more information, please follow other related articles on the PHP Chinese website!
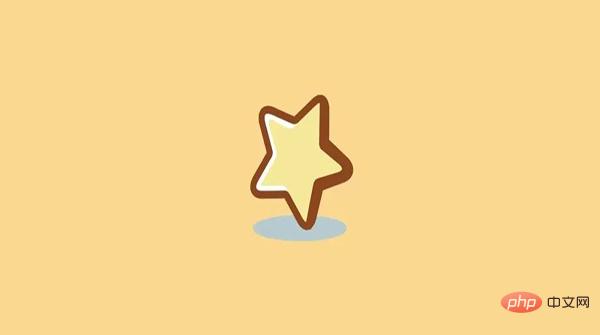
网上下载的 pdf 学习资料有一些会带有水印,非常影响阅读。比如下面的图片就是在 pdf 文件上截取出来的,今天我们就来用Python解决这个问题。安装模块PIL:Python Imaging Library 是 python 上非常强大的图像处理标准库,但是只能支持 python 2.7,于是就有志愿者在 PIL 的基础上创建了支持 python 3的 pillow,并加入了一些新的特性。pip install pillow pymupdf 可以用 python 访问扩展名为*.pdf、
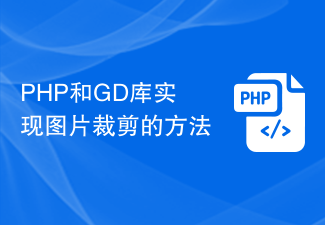
PHP和GD库实现图片裁剪的方法概述:图片裁剪是网页开发中常见的需求之一,它可以用于调整图片的尺寸,剪裁不需要的部分,以适应不同的页面布局和展示需求。在PHP开发中,我们可以借助GD库来实现图片裁剪的功能。GD库是一个强大的图形库,可提供一系列函数来处理和操控图像。代码示例:下面我们将详细介绍如何使用PHP和GD库来实现图片裁剪。首先,确保你的PHP环境已经
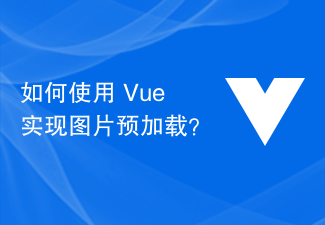
在网页开发中,图片预载是一种常见的技术,可以提升用户的体验感。当用户浏览网页时,图片可以提前下载并加载,减少图片加载时的等待时间。在Vue框架中,我们可以通过一些简单的方法来实现图片预载。本文将介绍Vue中的图片预载技术,包括预载的原理、实现的方法和使用注意事项。一、预载的原理首先,我们来了解一下图片预载的原理。传统的图片加载方式是等到图片全部下载完成才显示
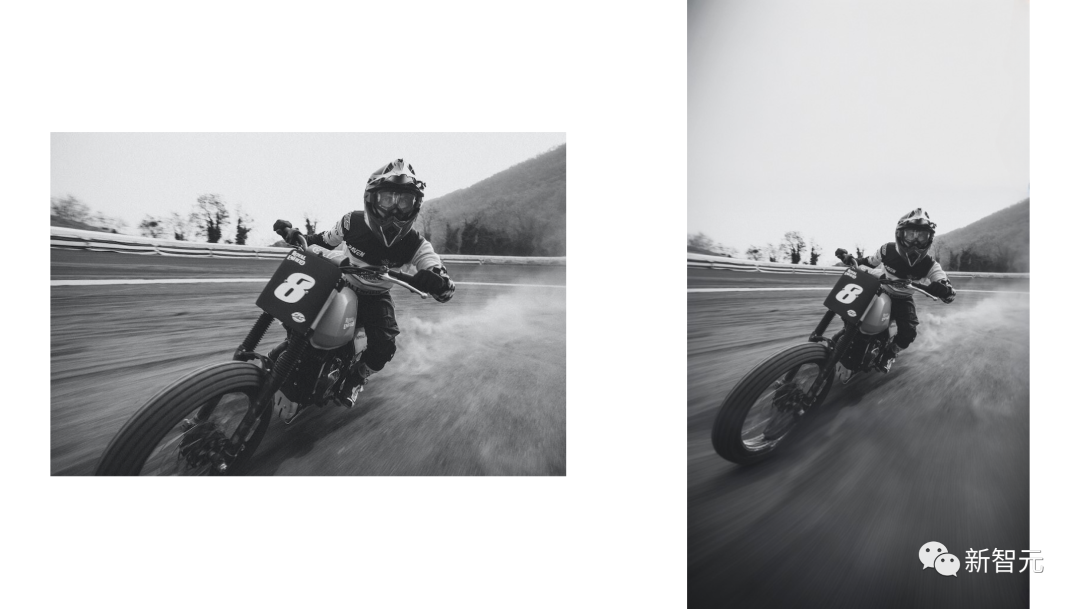
此前,PS的重建图像功能就让人无比振奋,让无数人惊呼今天,StabilityAI又放大招了。它联合Clipdrop推出了UncropClipdrop——一个终极图像比例编辑器。从Uncrop这个名字上,我们就能看出它的用途。它是一个AI生成的「外画」工具,通过创建扩展背景,这个工具可以补充任何现有照片或图像,来更改任何图像的比例。敲黑板:通过Clipdrop网站,就可以免费试用这个工具了,无需登录!比例任意调,满意为止Uncrop基于StabilityAI的文本到图像模型StableDiffus
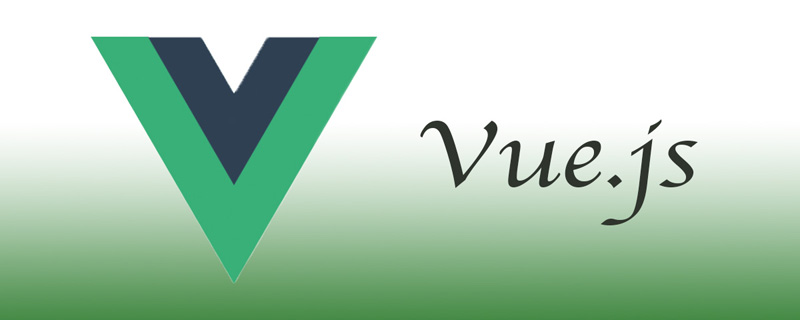
vue报错找不到图片的解决办法:1、修改配置文件,将绝对路径改为相对路径;2、将图片作为模块加载进去,并将图片放到static目录下;3、将imageUrls引入响应的vue文件中,解析引用即可。
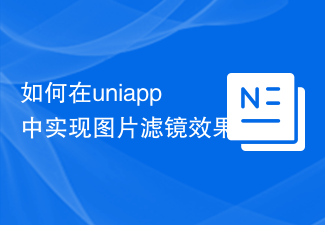
如何在uniapp中实现图片滤镜效果在移动应用开发中,图片滤镜效果是一种常见且受用户喜爱的功能之一。而在uniapp中,实现图片滤镜效果也并不复杂。本文将为大家介绍如何通过uniapp实现图片滤镜效果,并附上相关代码示例。导入图片首先,我们需要在uniapp项目中导入一张图片,以供后续滤镜效果的处理。可以在项目的资源文件夹中放置一张命名为“filter.jp
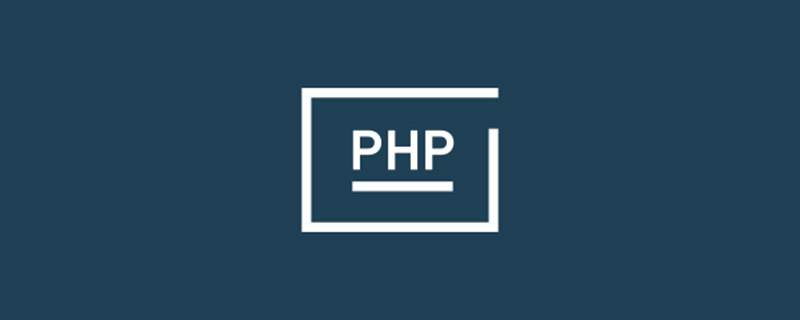
php写图片不显示不出来的解决办法:1、找到并打开php.ini文件;2、找到“extension=php_gd2.dll”,并将前面的分号去掉;3、重新启动服务器;4、在绘图前清一下缓存即可。
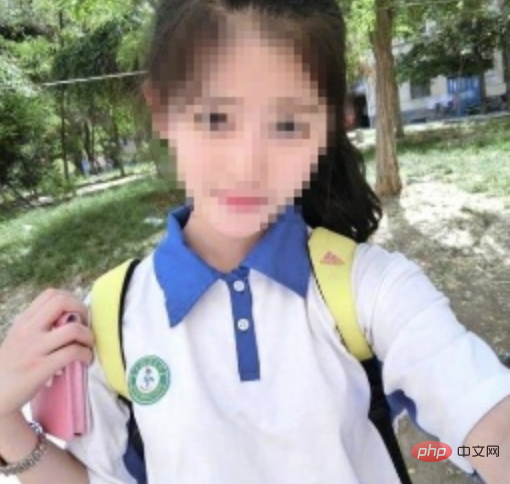
哈喽,大家好。你有没有想过用 AI 技术去除马赛克?仔细想想这个问题还挺难的,因为我们之前使用的 AI 技术,不管是人脸识别还是OCR识别,起码人工能识别出来。但如果给你一张打上马赛克的图片,你能把它复原吗?显然是很难的。如果人都无法复原,又怎能教会计算机去复原呢?还记得前几天我写的一篇《用AI生成头像》文章吗。在那篇文章中,我们训练了一个DCGAN模型,它可以从任意随机数生成一个图像。随机数作为像素生成的噪声图模型从随机数生成正常头像DCGAN包含生成器模型和判别器模型两个模型组成,生成


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
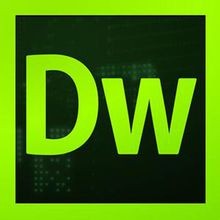
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment
