How to solve Java data parsing error exception (DataParsingErrorException)
Introduction:
In Java programming, you often encounter the situation of parsing data. However, due to incorrect data format or data type conversion errors, data parsing error exceptions (DataParsingErrorException) can easily occur. This article explains how to resolve this common exception in Java and provides relevant code examples.
1. Analyze the cause of the exception
Before solving the data parsing error exception, we first need to analyze the cause of the exception. Common data parsing error exceptions include but are not limited to the following:
- Data format error: For example, a string cannot be converted to a numeric type or a date format is incorrect.
- Data type error: For example, trying to assign a string to an integer variable.
- Missing data: For example, trying to parse an empty string or an uninitialized object.
2. Exception handling methods
We can adopt different processing methods for different data parsing error exceptions. The following are several common processing methods and their related code examples:
- Data format error handling:
When we try to convert a string to a numeric type, if the string format is incorrect , a NumberFormatException will be thrown. We can use try-catch block to catch this exception and handle it accordingly.
try { String str = "abc"; int num = Integer.parseInt(str); // 执行转换后的逻辑 } catch (NumberFormatException e) { // 处理数据格式错误 System.out.println("数据格式错误:" + e.getMessage()); }
- Data type error handling:
When we try to assign data to the wrong data type, a ClassCastException exception will be thrown. We can catch this exception using try-catch block and handle it accordingly.
try { Object obj = "Hello"; Integer num = (Integer) obj; // 执行类型转换后的逻辑 } catch (ClassCastException e) { // 处理数据类型错误 System.out.println("数据类型错误:" + e.getMessage()); }
- Missing data handling:
When we try to parse an empty string or an uninitialized object, a NullPointerException will be thrown. We can catch this exception using try-catch block and handle it accordingly.
try { String str = null; int len = str.length(); // 执行数据处理逻辑 } catch (NullPointerException e) { // 处理缺失数据 System.out.println("缺失数据:" + e.getMessage()); }
3. Exception defense measures
In addition to adopting targeted exception handling methods, we can also take some defensive measures to avoid the occurrence of data parsing error exceptions during the process of writing code.
- Data validation:
Before parsing the data, we can validate the data to ensure that it meets the expected format and type.
String str = "123"; if (str.matches("\d+")) { int num = Integer.parseInt(str); // 执行数据处理逻辑 } else { // 处理数据格式错误 System.out.println("数据格式错误"); }
- Uniform encapsulation of exception handling:
For code blocks where data parsing errors may occur in multiple places, we can uniformly encapsulate the exception handling logic to improve the reusability and availability of the code. Maintainability.
public void parseData(String str) throws DataParsingErrorException { try { int num = Integer.parseInt(str); // 执行数据处理逻辑 } catch (NumberFormatException e) { throw new DataParsingErrorException("数据格式错误:" + e.getMessage()); } }
Conclusion:
Data parsing error exceptions are common problems in Java programming, but by analyzing the causes of exceptions, choosing appropriate exception handling methods, and taking preventive measures, we can effectively solve this problem Class exception. Using the code examples and suggestions provided in this article, I believe readers can better handle Java data parsing error exceptions and improve the robustness and stability of the program.
The above is the detailed content of How to solve Java data parsing error exception (DataParsingErrorExceotion). For more information, please follow other related articles on the PHP Chinese website!
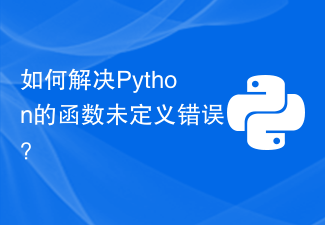
Python是一种面向对象的高级编程语言,具有简单、易读、易学等特点,因此被广泛应用于数据分析、人工智能、网站开发等领域。在Python编程过程中,我们常常会遇到函数未定义的错误,本文将介绍如何解决这个问题。定义函数首先,我们需要明确函数未定义错误的原因:通常是因为我们忘记或者未正确地定义某个函数。因此,我们需要检查代码中是否包含所有需要定义的函数,并确保它
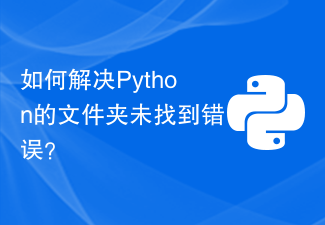
Python是一种流行的编程语言,但在使用中,经常会遇到一些错误。其中一个常见的错误是“文件夹未找到”。这个错误很容易让新手或者不熟悉Python的人感到困惑。在本文中,我们将讨论如何解决这个问题。1.确认文件夹路径是否正确在Python中,处理文件和文件夹的时候,需要指定文件和文件夹的路径。如果路径设置错误,那么就会导致程序无法找到文件夹。因此,我们需要先

<p>Xlive.dll是Microsoft的一个动态链接库(DLL),它是“WindowsLive游戏”的一部分。由Xlive.dll引起的错误可能是由于Xlive.dll文件的删除、放错位置、被恶意软件损坏或注册表项搞砸了。由于此错误而无法启动程序或游戏可能会令人沮丧。让我们看看解决这个问题的方法。此问题通常可以通过正确重新安装Xlive.dll文件来解决。</p><p><strong&
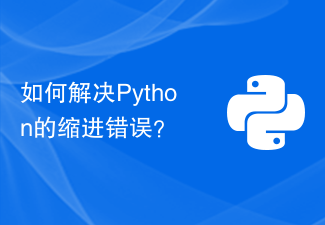
Python是一种非常流行的编程语言,由于其简洁明了的语法、易于学习以及丰富的生态系统得到了广泛的应用。然而,由于Python采用缩进作为代码块的标识,所以在编写Python程序的过程中,很容易遇到缩进错误的问题。缩进错误的原因可能是拼写错误、恰当使用缩进或可读性不好,这可能会导致代码运行失败或出现意想不到的结果。因此,在想要解决Python缩进错误的时候,
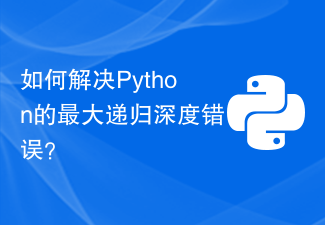
Python是一门易学易用的编程语言,然而在使用Python编写递归函数时,可能会遇到递归深度过大的错误,这时就需要解决这个问题。本文将为您介绍如何解决Python的最大递归深度错误。1.了解递归深度递归深度是指递归函数嵌套的层数。在Python默认情况下,递归深度的限制是1000,如果递归的层数超过这个限制,系统就会报错。这种报错通常称为“最大递归深度错误
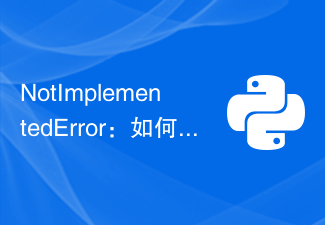
在Python编程中,当我们想要调用一个尚未实现的方法时,会出现NotImplementedError的错误提示。这个错误可以让我们感到困惑,因为它并没有明确告诉我们如何解决它。在本文中,我们将探讨NotImplementedError的原因,并提供一些解决方法,帮助您克服此错误。什么是NotImplementedError?NotImplementedEr
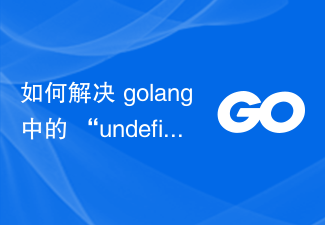
Go语言中的时间相关函数是非常常用的一部分,而time.Now()函数则是最常用的获取当前时间的方式。然而有时候我们在代码中调用这个函数却会出现"undefined:time.Now"的错误,那么我们该怎么解决这个问题呢?首先,我们需要了解一下这个错误的原因。Go语言的std库是根据当前Go版本编译生成的。当你的Go程序引入一个std
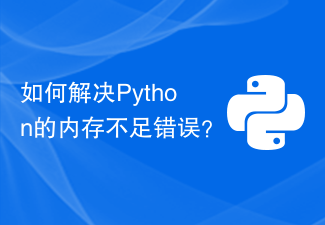
Python作为一种高级编程语言,在数据处理、科学计算、人工智能等领域广泛应用。不过,在这些应用场景中,Python的内存占用较高,甚至可能出现内存不足的情况。本文将介绍如何解决Python的内存不足错误。减少内存使用量Python语言本身并不是一个占用内存很大的语言。通常情况下,Python的内存使用量是由程序设计、数据结构、算法等因素共同决定的。因此,我


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
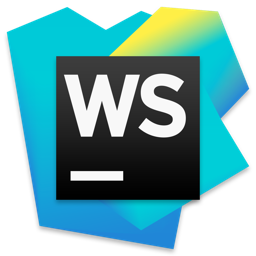
WebStorm Mac version
Useful JavaScript development tools
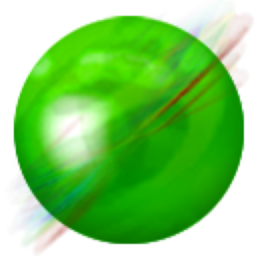
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
