How to fix: Java Layout Error: Components arranged incorrectly
Summary:
In graphical user interface (GUI) development in Java, correct layout is essential for creating Apps with a good user experience are crucial. However, sometimes we may encounter layout errors that cause components to be arranged incorrectly. This article will introduce some common Java layout errors and their solutions, and provide code examples.
- Using the wrong layout manager:
In Java, there are many layout managers to choose from, such as FlowLayout, BorderLayout, GridLayout, etc. Choosing a layout manager that doesn't suit your current needs can result in components being arranged incorrectly. The solution is to choose an appropriate layout manager based on actual needs.
Example:
import java.awt.*; import javax.swing.*; public class LayoutExample extends JFrame { public LayoutExample() { setTitle("布局示例"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // 使用错误的布局管理器 setLayout(new FlowLayout()); JButton button1 = new JButton("按钮1"); JButton button2 = new JButton("按钮2"); add(button1); add(button2); pack(); setVisible(true); } public static void main(String[] args) { SwingUtilities.invokeLater(() -> new LayoutExample()); } }
In the above code, the wrong layout manager FlowLayout is used, which will cause button 1 and button 2 to be arranged in the same row. To solve this problem, you can modify the layout manager to the correct layout manager, such as GridLayout.
- Wrong component constraint parameters:
For some layout managers, such as GridBagLayout and GridBagConstraints, the position and size of the component can be precisely controlled by setting the component's constraint parameters. However, if the wrong constraint parameters are set, the components will not be arranged correctly.
Example:
import java.awt.*; import javax.swing.*; public class ConstraintExample extends JFrame { public ConstraintExample() { setTitle("约束示例"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setLayout(new GridBagLayout()); JButton button1 = new JButton("按钮1"); JButton button2 = new JButton("按钮2"); // 使用错误的约束参数 GridBagConstraints constraints = new GridBagConstraints(); constraints.gridx = 0; constraints.gridy = 0; constraints.weightx = 1; constraints.weighty = 1; constraints.fill = GridBagConstraints.BOTH; add(button1, constraints); constraints.gridx = 1; constraints.gridy = 1; add(button2, constraints); pack(); setVisible(true); } public static void main(String[] args) { SwingUtilities.invokeLater(() -> new ConstraintExample()); } }
In the above code, wrong constraint parameters are used, causing button 1 and button 2 to be located at (0, 0). To solve this problem, you need to set the component's constraint parameters correctly to ensure that they are placed correctly where you want them to be.
Conclusion:
In GUI development in Java, correct layout is crucial to creating applications with a good user experience. This article introduces some common Java layout errors and provides corresponding solutions and code examples. By correctly choosing the layout manager and setting the correct component constraint parameters, we can solve the problem of incorrect component arrangement and achieve a beautiful and intuitive user interface.
The above is the detailed content of How to fix: Java Layout Error: Components arranged incorrectly. For more information, please follow other related articles on the PHP Chinese website!
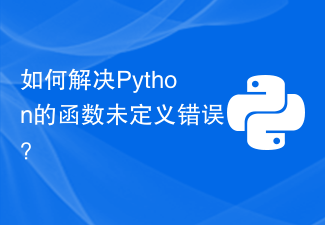
Python是一种面向对象的高级编程语言,具有简单、易读、易学等特点,因此被广泛应用于数据分析、人工智能、网站开发等领域。在Python编程过程中,我们常常会遇到函数未定义的错误,本文将介绍如何解决这个问题。定义函数首先,我们需要明确函数未定义错误的原因:通常是因为我们忘记或者未正确地定义某个函数。因此,我们需要检查代码中是否包含所有需要定义的函数,并确保它
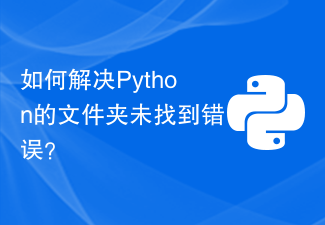
Python是一种流行的编程语言,但在使用中,经常会遇到一些错误。其中一个常见的错误是“文件夹未找到”。这个错误很容易让新手或者不熟悉Python的人感到困惑。在本文中,我们将讨论如何解决这个问题。1.确认文件夹路径是否正确在Python中,处理文件和文件夹的时候,需要指定文件和文件夹的路径。如果路径设置错误,那么就会导致程序无法找到文件夹。因此,我们需要先

<p>Xlive.dll是Microsoft的一个动态链接库(DLL),它是“WindowsLive游戏”的一部分。由Xlive.dll引起的错误可能是由于Xlive.dll文件的删除、放错位置、被恶意软件损坏或注册表项搞砸了。由于此错误而无法启动程序或游戏可能会令人沮丧。让我们看看解决这个问题的方法。此问题通常可以通过正确重新安装Xlive.dll文件来解决。</p><p><strong&
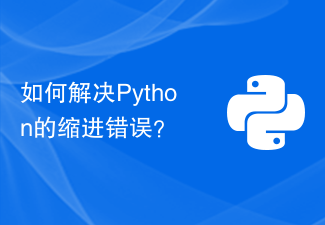
Python是一种非常流行的编程语言,由于其简洁明了的语法、易于学习以及丰富的生态系统得到了广泛的应用。然而,由于Python采用缩进作为代码块的标识,所以在编写Python程序的过程中,很容易遇到缩进错误的问题。缩进错误的原因可能是拼写错误、恰当使用缩进或可读性不好,这可能会导致代码运行失败或出现意想不到的结果。因此,在想要解决Python缩进错误的时候,
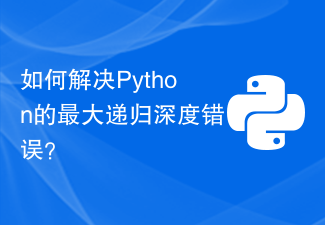
Python是一门易学易用的编程语言,然而在使用Python编写递归函数时,可能会遇到递归深度过大的错误,这时就需要解决这个问题。本文将为您介绍如何解决Python的最大递归深度错误。1.了解递归深度递归深度是指递归函数嵌套的层数。在Python默认情况下,递归深度的限制是1000,如果递归的层数超过这个限制,系统就会报错。这种报错通常称为“最大递归深度错误
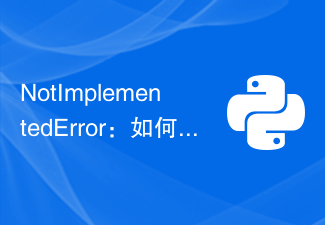
在Python编程中,当我们想要调用一个尚未实现的方法时,会出现NotImplementedError的错误提示。这个错误可以让我们感到困惑,因为它并没有明确告诉我们如何解决它。在本文中,我们将探讨NotImplementedError的原因,并提供一些解决方法,帮助您克服此错误。什么是NotImplementedError?NotImplementedEr
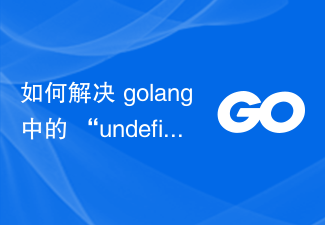
Go语言中的时间相关函数是非常常用的一部分,而time.Now()函数则是最常用的获取当前时间的方式。然而有时候我们在代码中调用这个函数却会出现"undefined:time.Now"的错误,那么我们该怎么解决这个问题呢?首先,我们需要了解一下这个错误的原因。Go语言的std库是根据当前Go版本编译生成的。当你的Go程序引入一个std
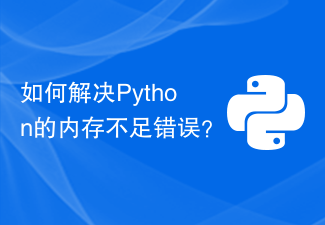
Python作为一种高级编程语言,在数据处理、科学计算、人工智能等领域广泛应用。不过,在这些应用场景中,Python的内存占用较高,甚至可能出现内存不足的情况。本文将介绍如何解决Python的内存不足错误。减少内存使用量Python语言本身并不是一个占用内存很大的语言。通常情况下,Python的内存使用量是由程序设计、数据结构、算法等因素共同决定的。因此,我


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
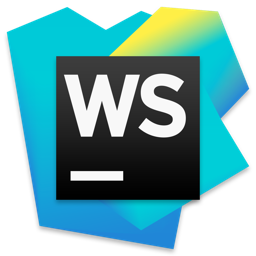
WebStorm Mac version
Useful JavaScript development tools
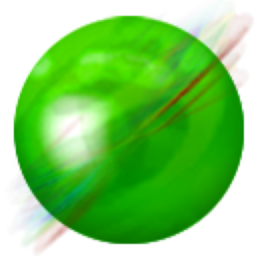
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
