Golang Image Processing: Learn How to Add Watermarks and Text
Golang Image Processing: Learn how to add watermarks and text
Introduction:
In the modern era of digitalization and social media, image processing has become an important skills. Whether for personal use or business operations, adding watermarks and text are common needs. In this article, we will explore how to use Golang for image processing and learn how to add watermarks and text.
Background:
Golang is an open source programming language known for its concise syntax, efficient performance and powerful concurrency capabilities. It has become one of the preferred languages for many developers. In Golang, there are some powerful third-party libraries that make image processing easy and efficient.
Add watermark:
Adding watermark is a common technique to protect the copyright of personal or commercial images. The following is an example that shows how to use Golang to add a watermark to an image:
package main import ( "image" "image/draw" "image/jpeg" "os" ) func main() { // 打开原始图片 file, err := os.Open("original.jpg") if err != nil { panic(err) } defer file.Close() // 解码图片 img, _, err := image.Decode(file) if err != nil { panic(err) } // 创建一个画布 bounds := img.Bounds() canvas := image.NewRGBA(bounds) // 绘制原始图片到画布上 draw.Draw(canvas, bounds, img, image.Point{}, draw.Src) // 添加水印 watermark := image.NewRGBA(image.Rect(0, 0, 100, 50)) draw.Draw(canvas, image.Rect(bounds.Dx()-100, bounds.Dy()-50, bounds.Dx(), bounds.Dy()), watermark, image.Point{}, draw.Src) // 保存处理后的图片 output, err := os.Create("output.jpg") if err != nil { panic(err) } defer output.Close() // 编码保存到文件 jpeg.Encode(output, canvas, nil) }
The above code first opens an image file named "original.jpg". It is then decoded into an image.Image
object, then a new RGBA canvas is created, and the original image is drawn onto the canvas. Finally, a 100x50 sized watermark was created and drawn to the lower right corner of the canvas. The final generated image with watermark is saved as "output.jpg".
Add text:
Adding text is another common image processing requirement. It can be used to add titles, descriptions or other instructions. Here is an example that shows how to add text on an image using Golang:
package main import ( "image" "image/draw" "image/jpeg" "os" "github.com/golang/freetype" "github.com/golang/freetype/truetype" "golang.org/x/image/font" ) func main() { // 打开原始图片 file, err := os.Open("original.jpg") if err != nil { panic(err) } defer file.Close() // 解码图片 img, _, err := image.Decode(file) if err != nil { panic(err) } // 创建一个画布 bounds := img.Bounds() canvas := image.NewRGBA(bounds) // 绘制原始图片到画布上 draw.Draw(canvas, bounds, img, image.Point{}, draw.Src) // 添加文字 fontBytes, err := os.ReadFile("font.ttf") if err != nil { panic(err) } font, err := freetype.ParseFont(fontBytes) if err != nil { panic(err) } context := freetype.NewContext() context.SetDPI(72) context.SetFont(font) context.SetFontSize(24) context.SetClip(bounds) context.SetDst(canvas) context.SetSrc(image.Black) pt := freetype.Pt(10, 10+int(context.PointToFixed(24)>>6)) context.DrawString("Hello, Golang!", pt) // 保存处理后的图片 output, err := os.Create("output.jpg") if err != nil { panic(err) } defer output.Close() // 编码保存到文件 jpeg.Encode(output, canvas, nil) }
The above code is similar to the example of adding a watermark, but we use the third-party library freetype to add text. First, an image file named "original.jpg" is opened and then decoded into an image.Image object. Then a new RGBA canvas is created and the original image is drawn onto the canvas. Then, a TrueType font file is loaded and parsed into a freetype.Font object. Created a freetype.Context object and set drawing parameters such as font and font size. Finally, the DrawString function is called to add text to the canvas. The final generated image is saved as "output.jpg".
Conclusion:
As a powerful programming language, Golang has a wealth of third-party libraries and tools, making image processing simple and efficient. This article introduces how to use Golang to add watermarks and text to images, and provides corresponding code examples. I hope this article can help readers learn how to use Golang for image processing and play a role in practical applications.
The above is the detailed content of Golang Image Processing: Learn How to Add Watermarks and Text. For more information, please follow other related articles on the PHP Chinese website!
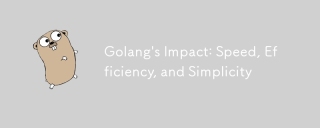
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
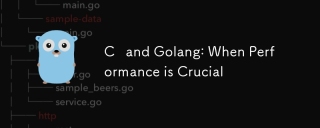
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
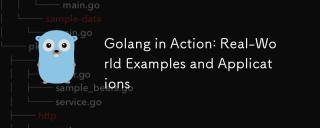
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
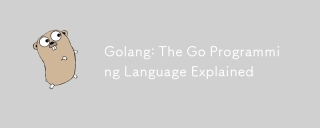
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
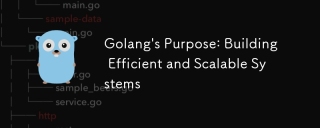
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
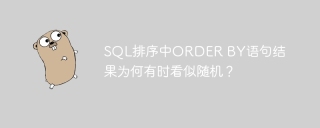
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
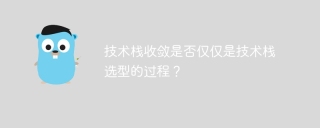
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
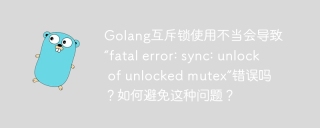
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),