


Caching and performance optimization in Laravel: speed up application response and processing
Caching and performance optimization in Laravel: Accelerating application response and processing
When developing web applications, performance optimization is a very important task. A high-performance application provides a better user experience and is more scalable. In the Laravel framework, caching and performance optimization are two very important topics. This article will introduce how to use Laravel's caching system to speed up application response and processing.
- Introduction to Laravel Caching System
Laravel provides a powerful caching system for caching various data of the application, such as database query results, view templates, etc. The caching system can store this data in memory, reducing the number of database queries and calculations, thereby significantly improving application performance and response speed. Here is a simple code example that demonstrates how to use Laravel's caching system to cache database query results:
// 使用缓存系统缓存数据库查询结果 $users = Cache::remember('users', 60, function () { return DB::table('users')->get(); }); // 当缓存未命中时,会执行回调函数来获取新的数据并缓存
In the above example, the Cache::remember
method accepts three parameters: Cache key name, cache time (unit: minutes), callback function. If the corresponding key already exists in the cache, the data in the cache is returned directly. If the cache does not exist, execute the callback function to obtain new data and store it in the cache.
- Cache Driver
Laravel's cache system supports a variety of cache drivers, including file cache, database cache, Redis cache, etc. Different cache drivers are suitable for different application scenarios, and developers can choose the appropriate driver according to their own needs. By default, Laravel uses the file cache drive, but the default drive can be changed through a configuration file.
The following is a sample code using the Redis cache driver:
// 在配置文件中指定Redis作为缓存驱动器 'cache' => [ 'default' => env('CACHE_DRIVER', 'redis'), 'stores' => [ 'redis' => [ 'driver' => 'redis', 'connection' => 'default', ], ], ], // 使用Redis缓存驱动器缓存数据库查询结果 $users = Cache::store('redis')->remember('users', 60, function () { return DB::table('users')->get(); });
In the above code example, we specify Redis as the cache driver through the configuration file. Then, specify the use of the Redis cache driver for caching operations through the Cache::store('redis')
method.
- Data caching and view caching
In Laravel, different types of data can be cached, including database query results, API response data, view templates, etc. For caching of database query results, we can use the Cache::remember
method introduced earlier. For view caching, we can use the @cache
directive to achieve it. Here is a simple view caching example code:
{{-- 使用@cache指令来缓存部分视图 --}} @cache('sidebar', 60) <div class="sidebar"> {{-- 渲染侧边栏内容 --}} </div> @endcache
In the above code example, we use the @cache('sidebar', 60)
directive to cache the <div the content in class="sidebar"> tag is cached for 60 minutes. When the cache expires or misses, the sidebar content is re-rendered and stored in the cache. <ol start="4"><li>Cache Clearing and Invalidation</li></ol>
<p>While the application is running, there may be situations where you need to manually clear or invalidate the cache. In Laravel, we can use the methods provided by the <code>Cache
facade class to implement cache clearing and invalidation. Here is some sample code:
// 清除指定缓存键的缓存 Cache::forget('users'); // 清除所有缓存 Cache::flush(); // 使指定缓存键的缓存失效 Cache::put('users', $users, 60);
In the above code example, the Cache::forget
method is used to clear the cache for the specified cache key, Cache::flush
Method is used to clear all caches. In addition, the Cache::put
method is used to set the cache of the specified cache key and specify the expiration time.
Conclusion
Caching and performance optimization are one of the key elements in developing high-performance web applications. Laravel provides a powerful caching system that can help us cache various data and provide faster response and processing speed. By using appropriate cache drivers and sound caching strategies, we can maximize the performance and responsiveness of our applications. However, it should be noted that caching is not a mindless use. For frequently changing data or data that needs to be updated in real time, caching strategies should be chosen carefully. In actual development, developers need to use the cache system appropriately based on application scenarios and performance requirements to obtain the best performance and user experience.
Through the introduction and sample code of this article, I believe readers can better understand and apply the caching system in Laravel, and further optimize the performance and response speed of their own applications. I hope this article is helpful to readers, thank you for reading!
The above is the detailed content of Caching and performance optimization in Laravel: speed up application response and processing. For more information, please follow other related articles on the PHP Chinese website!

Laravel optimizes the web development process including: 1. Use the routing system to manage the URL structure; 2. Use the Blade template engine to simplify view development; 3. Handle time-consuming tasks through queues; 4. Use EloquentORM to simplify database operations; 5. Follow best practices to improve code quality and maintainability.

Laravel is a modern PHP framework that provides a powerful tool set, simplifies development processes and improves maintainability and scalability of code. 1) EloquentORM simplifies database operations; 2) Blade template engine makes front-end development intuitive; 3) Artisan command line tools improve development efficiency; 4) Performance optimization includes using EagerLoading, caching mechanism, following MVC architecture, queue processing and writing test cases.

Laravel's MVC architecture improves the structure and maintainability of the code through models, views, and controllers for separation of data logic, presentation and business processing. 1) The model processes data, 2) The view is responsible for display, 3) The controller processes user input and business logic. This architecture allows developers to focus on business logic and avoid falling into the quagmire of code.

Laravel is a PHP framework based on MVC architecture, with concise syntax, powerful command line tools, convenient data operation and flexible template engine. 1. Elegant syntax and easy-to-use API make development quick and easy to use. 2. Artisan command line tool simplifies code generation and database management. 3.EloquentORM makes data operation intuitive and simple. 4. The Blade template engine supports advanced view logic.

Laravel is suitable for building backend services because it provides elegant syntax, rich functionality and strong community support. 1) Laravel is based on the MVC architecture, simplifying the development process. 2) It contains EloquentORM, optimizes database operations. 3) Laravel's ecosystem provides tools such as Artisan, Blade and routing systems to improve development efficiency.
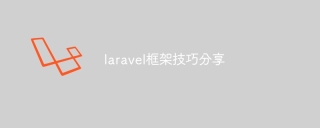
In this era of continuous technological advancement, mastering advanced frameworks is crucial for modern programmers. This article will help you improve your development skills by sharing little-known techniques in the Laravel framework. Known for its elegant syntax and a wide range of features, this article will dig into its powerful features and provide practical tips and tricks to help you create efficient and maintainable web applications.
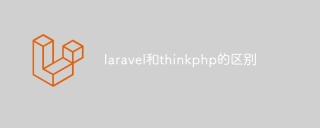
Laravel and ThinkPHP are both popular PHP frameworks and have their own advantages and disadvantages in development. This article will compare the two in depth, highlighting their architecture, features, and performance differences to help developers make informed choices based on their specific project needs.
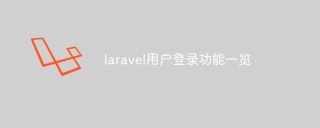
Building user login capabilities in Laravel is a crucial task and this article will provide a comprehensive overview covering every critical step from user registration to login verification. We will dive into the power of Laravel’s built-in verification capabilities and guide you through customizing and extending the login process to suit specific needs. By following these step-by-step instructions, you can create a secure and reliable login system that provides a seamless access experience for users of your Laravel application.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
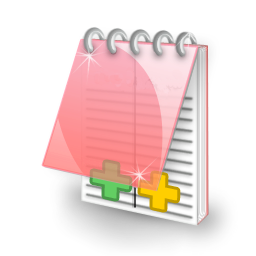
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool