ThinkPHP6 FAQ: What should I do if I encounter a problem?
Introduction:
ThinkPHP6 is a widely used PHP framework with powerful functions and flexible development methods. However, although the framework has been rigorously tested and optimized, you may still encounter some problems during use. This article will briefly introduce some common problems you may encounter when using ThinkPHP6 in the form of Q&A, and provide corresponding solutions.
1. Question: How to deal with routing problems?
Answer: In ThinkPHP6, URL routing can be handled by configuring routing rules in the routing file. By default, the route configuration file is located at route/route.php
. You can configure routing rules according to the following example:
use thinkacadeRoute; // 完全匹配路由规则 Route::get('index', 'Index/index'); // 带参数的路由规则 Route::get('map/:id/[:city]', 'Map/index'); // 使用闭包处理路由请求 Route::get('test', function () { return 'Hello, ThinkPHP6!'; });
2. Question: How to use database operations?
Answer: ThinkPHP6 has built-in powerful database operation classes, which can easily perform database addition, deletion, modification and query operations. First, configure the database connection information in the configuration file database.php
, and then you can use the code in the following example to perform database operations:
use thinkacadeDb; // 查询操作 $result = Db::table('user')->where('id', 1)->find(); // 添加操作 $data = ['name' => 'John', 'age' => 20]; Db::table('user')->insert($data); // 更新操作 Db::table('user')->where('id', 1)->update(['name' => 'Bob']); // 删除操作 Db::table('user')->where('id', 1)->delete();
3. Question: How to handle form validation?
Answer: In ThinkPHP6, you can use the built-in validator class for form validation. First, you need to introduce the Validator class in the controller. When processing form submission, you can use the code in the following example for form verification:
use thinkacadeValidate; // 表单验证规则 $rule = [ 'name' => 'require|max:25', 'email' => 'email', 'age' => 'number|between:1,100', ]; // 错误信息提示 $message = [ 'name.require' => '名称必须', 'name.max' => '名称最多不能超过25个字符', 'email' => '邮箱格式错误', 'age.number' => '年龄必须是数字', 'age.between' => '年龄只能在1-100之间', ]; // 数据验证 $validate = Validate::rule($rule)->message($message); if (!$validate->check($data)) { // 输出错误信息 dump($validate->getError()); }
4. Question: How to use the model for data operations?
Answer: The model is an important component in ThinkPHP6 used to handle database operations, and can easily read and write data. First, you need to create a model class, in which you can define some database operation methods. The code in the following example demonstrates the basic usage of the model:
namespace appmodel; use thinkModel; class User extends Model { // 模型对应的数据表名 protected $table = 'user'; // 查询用户信息 public function getUserInfo($id) { return $this->where('id', $id)->find(); } // 添加用户 public function addUser($data) { return $this->insert($data); } // 更新用户信息 public function updateUserInfo($id, $data) { return $this->where('id', $id)->update($data); } // 删除用户 public function deleteUser($id) { return $this->where('id', $id)->delete(); } }
Sample code for using the model for data operations:
use appmodelUser; // 查询用户信息 $userModel = new User(); $userInfo = $userModel->getUserInfo(1); // 添加用户 $data = ['name' => 'John', 'age' => 20]; $userModel->addUser($data); // 更新用户信息 $userModel->updateUserInfo(1, ['name' => 'Bob']); // 删除用户 $userModel->deleteUser(1);
Conclusion:
This article introduces some issues that may arise when using ThinkPHP6 Common problems encountered and corresponding solutions provided. By reading this article, readers can better master the use of ThinkPHP6 and improve development efficiency and quality. However, it is important to note that this article only provides brief answers to some questions and does not cover all issues. In actual development, if you encounter more complex problems, it is recommended to consult the official documentation or seek professional help.
The above is the detailed content of ThinkPHP6 FAQ: What should I do if I encounter a problem?. For more information, please follow other related articles on the PHP Chinese website!
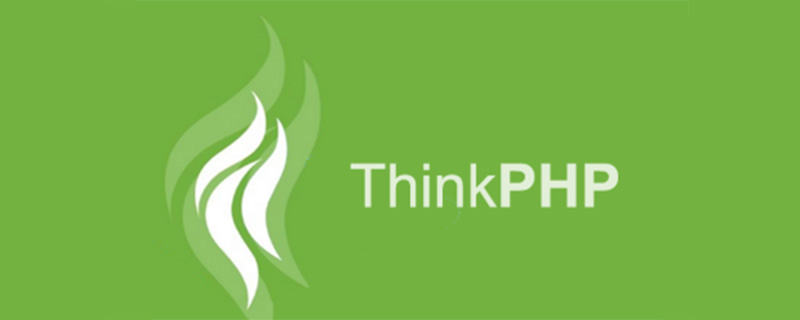
thinkphp是国产框架。ThinkPHP是一个快速、兼容而且简单的轻量级国产PHP开发框架,是为了简化企业级应用开发和敏捷WEB应用开发而诞生的。ThinkPHP从诞生以来一直秉承简洁实用的设计原则,在保持出色的性能和至简的代码的同时,也注重易用性。
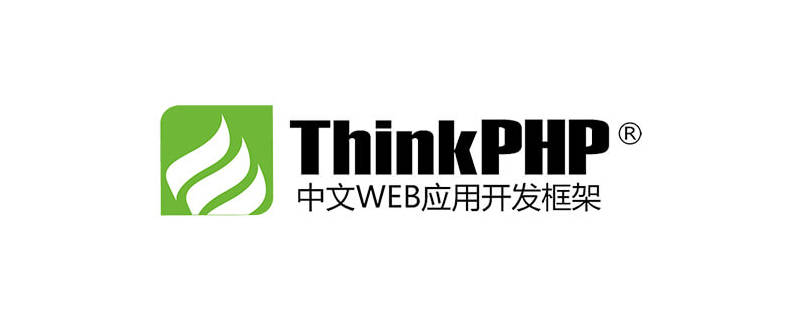
本篇文章给大家带来了关于thinkphp的相关知识,其中主要介绍了关于使用think-queue来实现普通队列和延迟队列的相关内容,think-queue是thinkphp官方提供的一个消息队列服务,下面一起来看一下,希望对大家有帮助。
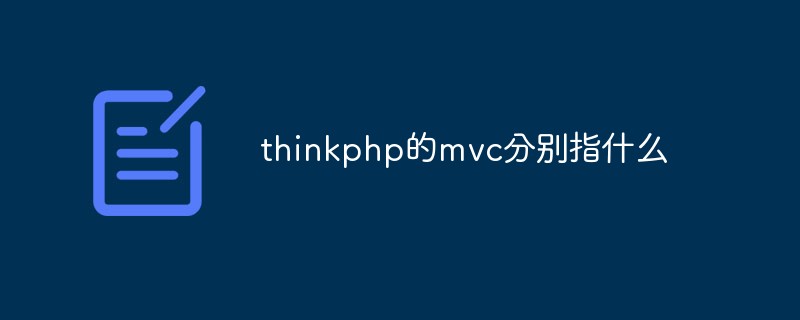
thinkphp基于的mvc分别是指:1、m是model的缩写,表示模型,用于数据处理;2、v是view的缩写,表示视图,由View类和模板文件组成;3、c是controller的缩写,表示控制器,用于逻辑处理。mvc设计模式是一种编程思想,是一种将应用程序的逻辑层和表现层进行分离的方法。
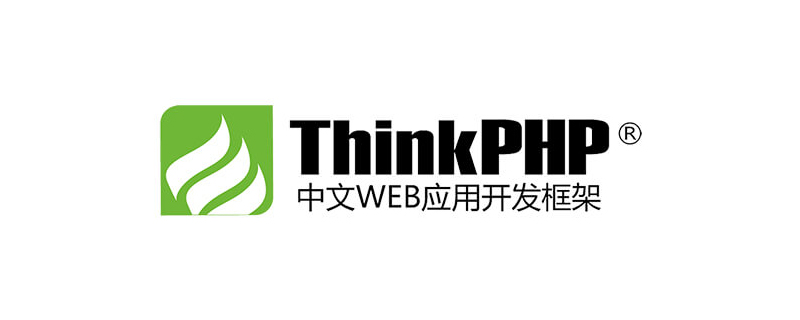
本篇文章给大家带来了关于thinkphp的相关知识,其中主要介绍了使用jwt认证的问题,下面一起来看一下,希望对大家有帮助。
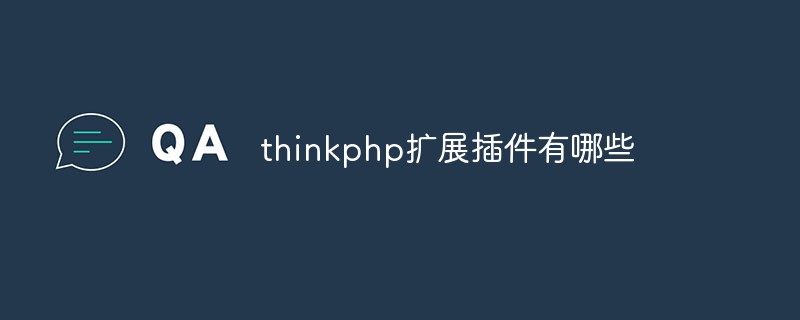
thinkphp扩展有:1、think-migration,是一种数据库迁移工具;2、think-orm,是一种ORM类库扩展;3、think-oracle,是一种Oracle驱动扩展;4、think-mongo,一种MongoDb扩展;5、think-soar,一种SQL语句优化扩展;6、porter,一种数据库管理工具;7、tp-jwt-auth,一个jwt身份验证扩展包。
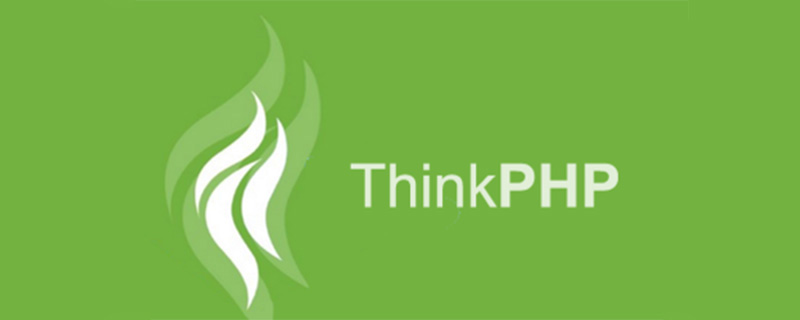
本篇文章给大家带来了关于ThinkPHP的相关知识,其中主要整理了使用think-queue实现redis消息队列的相关问题,下面一起来看一下,希望对大家有帮助。
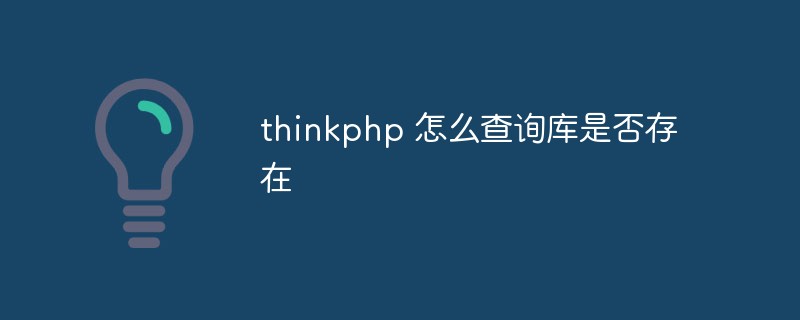
thinkphp查询库是否存在的方法:1、打开相应的tp文件;2、通过“ $isTable=db()->query('SHOW TABLES LIKE '."'".$data['table_name']."'");if($isTable){...}else{...}”方式验证表是否存在即可。
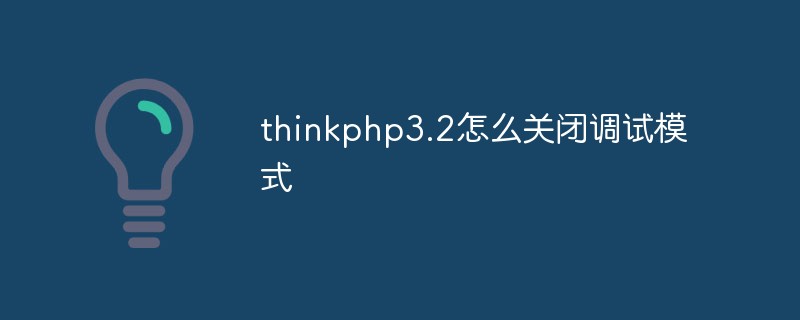
在thinkphp3.2中,可以利用define关闭调试模式,该标签用于变量和常量的定义,将入口文件中定义调试模式设为FALSE即可,语法为“define('APP_DEBUG', false);”;开启调试模式将参数值设置为true即可。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
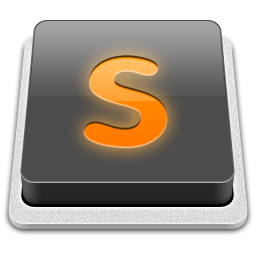
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.