


Explore the aggregation relationship in PHP object-oriented programming
In object-oriented programming, the aggregation relationship is a way to represent the relationship between objects. In PHP, using aggregation relationships can effectively organize and manage dependencies between objects, improving code reusability and maintainability. This article will explore aggregation relationships in PHP object-oriented programming and provide relevant code examples.
Aggregation relationship is a strong association relationship, which means that one object contains other objects, and these objects can exist independently. In an aggregation relationship, the contained objects are called aggregate objects, and the objects that contain other objects are called aggregate objects. Aggregation relationships can be used to represent the relationship between the whole and parts, such as the relationship between a class and multiple students, the relationship between an order and multiple products, etc.
In PHP, a common way to implement an aggregation relationship is by using one object as a property of another object. For example, we can have a class class (Classroom) that contains multiple student objects (Student). The following is a simple code example:
class Student { private $name; public function __construct($name) { $this->name = $name; } public function getName() { return $this->name; } } class Classroom { private $students = array(); public function addStudent(Student $student) { $this->students[] = $student; } public function getStudents() { return $this->students; } } // 创建学生对象 $student1 = new Student('张三'); $student2 = new Student('李四'); $student3 = new Student('王五'); // 创建班级对象 $classroom = new Classroom(); // 添加学生到班级中 $classroom->addStudent($student1); $classroom->addStudent($student2); $classroom->addStudent($student3); // 获取班级中的学生列表 $students = $classroom->getStudents(); // 输出学生列表 foreach ($students as $student) { echo $student->getName() . PHP_EOL; }
In the above example, we first define a student class (Student), which has a private property $name and a public method getName() for obtaining students name. Then we defined a class class (Classroom), which has a private property $students for storing an array of student objects, a public method addStudent() for adding student objects to the class, and a public method getStudents() for Returns the list of students in the class. We create several student objects and add them to the class object. Finally, we get the student list by calling the getStudents() method and output the students' names in sequence.
Through aggregation relationships, we can easily manage dependencies between objects. For example, in the above example, a class object can contain multiple student objects, and these student objects can exist independently. Such a design can improve the flexibility and scalability of the code, and aggregate objects can be easily added, deleted, or manipulated in subsequent operations.
In summary, the aggregation relationship in PHP object-oriented programming is a way to represent the relationship between objects, by treating one object as a property of another object. Through aggregation relationships, we can easily organize and manage dependencies between objects and improve code reusability and maintainability. In practical applications, we can design and use aggregation relationships according to specific needs, making the code clearer, readable, and easier to maintain.
The above is the detailed content of Explore Aggregation Relationships in PHP Object-Oriented Programming. For more information, please follow other related articles on the PHP Chinese website!
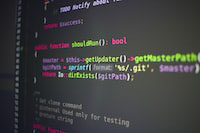
什么是面向对象编程?面向对象编程(OOP)是一种编程范式,它将现实世界中的实体抽象为类,并使用对象来表示这些实体。类定义了对象的属性和行为,而对象则实例化了类。OOP的主要优点在于它可以使代码更易于理解、维护和重用。OOP的基本概念OOP的主要概念包括类、对象、属性和方法。类是对象的蓝图,它定义了对象的属性和行为。对象是类的实例,它具有类的所有属性和行为。属性是对象的特征,它可以存储数据。方法是对象的函数,它可以对对象的数据进行操作。OOP的优点OOP的主要优点包括:可重用性:OOP可以使代码更
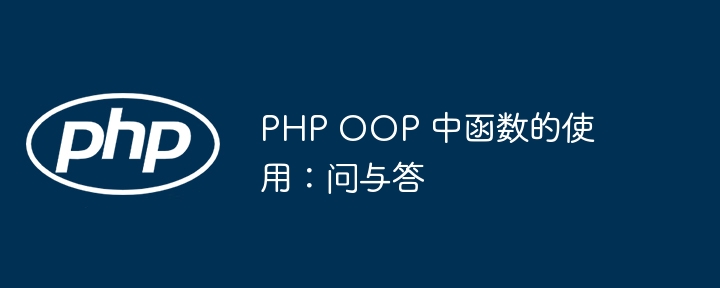
PHPOOP中函数有两种类型:类方法和静态方法。类方法属于特定类,由该类实例调用;静态方法不属于任何类,通过类名调用。类方法使用publicfunction声明,静态方法使用publicstaticfunction声明。类方法通过对象实例调用($object->myMethod()),静态方法直接通过类名调用(MyClass::myStaticMethod())。
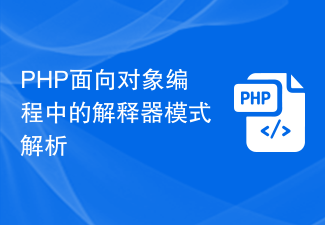
PHP面向对象编程中的解释器模式解析导语:在面向对象编程中,解释器模式是一种行为设计模式。该模式用于将一种语言的语法表示为一个解释器,并提供了一种解释该语法的方式。在PHP中,解释器模式可以帮助我们根据特定规则进行解析和处理字符串或文本。介绍:解释器模式是行为型设计模式的一种,它通过创建一个解释器来解释特定的语法规则。这种模式通常用于处理一些特定的语言或表达
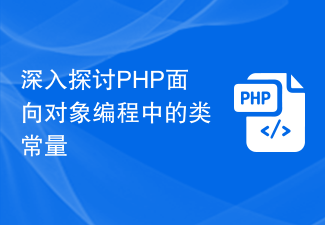
PHP是一种常用的编程语言,广泛用于web应用程序的开发。在PHP的面向对象编程中,类常量是一种重要的概念。本文将深入探讨PHP面向对象编程中的类常量,并提供一些代码示例来帮助读者更好地理解和应用。一、类常量的定义和特点类常量是在类定义中声明的不可变的值。与普通的类属性不同,类常量在整个类的生命周期中保持不变,可以通过类名直接访问。定义类常量时使用关键字co
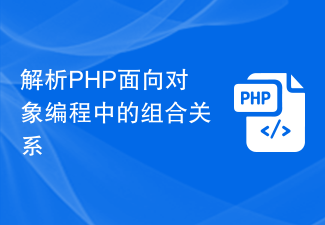
解析PHP面向对象编程中的组合关系组合关系是面向对象编程中常用的一种关系模式,它描述的是一个对象包含其他对象的情况。在PHP中,通过使用类的属性来实现组合关系。在这篇文章中,我们将讨论PHP中的组合关系的概念,并通过代码示例来说明如何实现和使用组合关系。组合关系的概念是指一个类的实例对象包含另一个类的实例对象的情况。这种关系是一种强依赖关系,包含的对象一般是
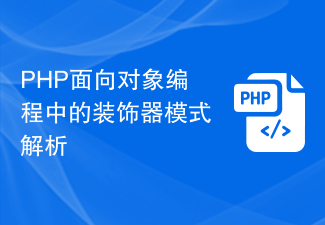
PHP面向对象编程中的装饰器模式解析引言:在面向对象编程的世界中,装饰器模式是一种非常有用的设计模式。它可以在不改变已有对象的结构和功能的前提下,动态地给对象添加一些额外的功能。在本文中,我们将深入探讨装饰器模式在PHP中的应用,并通过实际的代码示例来更好地理解其实现原理。一、什么是装饰器模式?装饰器模式是一种结构型设计模式,它允许我们通过包装已有对象,来动
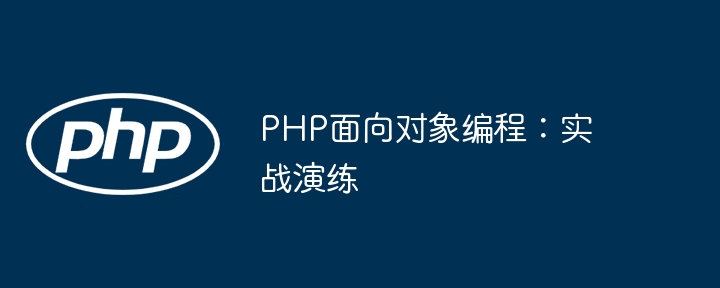
PHP面向对象编程(OOP)是模拟真实实体的编程范例。OOP的核心概念包括:类和对象:类定义对象的蓝图,对象是类的实例。封装性:对象属性和方法与其他代码隔离开来。继承:子类可以继承父类的属性和方法。多态性:同名方法在运行时根据对象的类型表现出不同的行为。
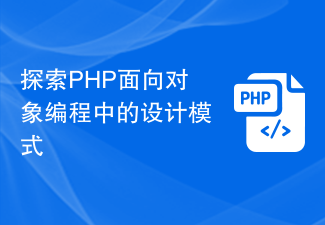
探索PHP面向对象编程中的设计模式设计模式是软件开发中经过实践验证的解决问题的模板。在PHP面向对象编程中,设计模式能够帮助我们更好地组织和管理代码,提高代码的可维护性和可扩展性。本文将讨论几种常见的设计模式,并给出相应的PHP示例。单例模式(SingletonPattern)单例模式用于创建只允许存在一个实例的类。在PHP中,我们可以通过使用静态成员和私


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
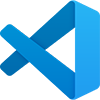
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
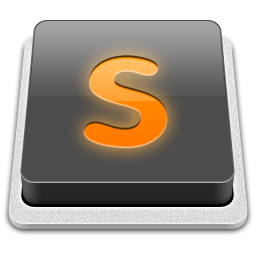
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
