Microservice task queue scheduler implemented in Go language
With the popularity of microservice architecture, task queue scheduler plays an important role in various application scenarios Role. As a programming language known for its high concurrency and high efficiency, Go language is very suitable for implementing task queue schedulers. This article will introduce how to use Go language to implement a simple microservice task queue scheduler and provide corresponding code examples.
1. The basic principle of the task queue scheduler
The task queue scheduler is a technology widely used in microservice architecture. It schedules various types of tasks according to certain Strategies are assigned to different worker nodes for execution. It usually consists of the following main components:
- Task queue: used to store tasks to be executed.
- Scheduler: Select tasks from the task queue according to a certain strategy and assign them to available worker nodes.
- Working node: The computing node that actually executes the task.
The main function of the scheduler is to select tasks from the task queue and distribute them to available worker nodes. For the case of multiple working nodes, the scheduler usually adopts a load balancing strategy to ensure that each working node executes tasks in a balanced manner. At the same time, the scheduler also needs to handle abnormal situations in the task queue, such as task execution failure or timeout, etc.
2. Use the Go language to implement the core code of the task queue scheduler
The following is an example of the core code using the Go language to implement the task queue scheduler:
package main import ( "fmt" "sync" ) type TaskQueue struct { queue []string mutex sync.Mutex } func (tq *TaskQueue) Push(task string) { tq.mutex.Lock() defer tq.mutex.Unlock() tq.queue = append(tq.queue, task) } func (tq *TaskQueue) Pop() string { tq.mutex.Lock() defer tq.mutex.Unlock() if len(tq.queue) == 0 { return "" } task := tq.queue[0] tq.queue = tq.queue[1:] return task } type Worker struct { id int queue *TaskQueue finish chan bool } func (w *Worker) start() { for { task := w.queue.Pop() if task == "" { break } fmt.Printf("Worker %d is processing task: %s ", w.id, task) // 执行任务的逻辑 } w.finish <- true } func main() { queue := &TaskQueue{} workers := make([]*Worker, 5) finish := make(chan bool) for i := range workers { workers[i] = &Worker{ id: i, queue: queue, finish: finish, } go workers[i].start() } tasks := []string{"task1", "task2", "task3", "task4", "task5"} for _, task := range tasks { queue.Push(task) } // 等待所有任务执行完成 for range workers { <-finish } fmt.Println("All tasks have been processed") }
In the above code , we defined the TaskQueue
structure to store the task queue, and used the mutex lock sync.Mutex
to ensure concurrency safety. TaskQueue
The structure contains the queue
field for saving tasks in the task queue, and provides the Push
and Pop
methods for populating the queue. Add tasks and remove tasks.
Then, we defined the Worker
structure to represent the work node. Each worker node holds a TaskQueue
object and notifies the scheduler that task execution is completed through the finish
channel. Worker
The start
method of the structure is used to execute the logic of the task.
In the main
function, we create a TaskQueue
instance and multiple Worker
instances, and add tasks to the task queue. Subsequently, we use the go
keyword to start multiple worker nodes and wait for all task execution to be completed through the finish
channel.
3. Summary
This article introduces how to use Go language to implement a simple microservice task queue scheduler, and gives corresponding code examples. Through this example, we can see that writing the code of the task queue scheduler using Go language is very simple and intuitive. With the powerful concurrency capabilities of the Go language, we can easily implement an efficient and scalable task queue scheduler, thereby improving system performance and reliability under the microservice architecture.
I hope this article can help readers better understand the basic principles of the task queue scheduler and how to implement it using the Go language.
The above is the detailed content of Microservice task queue scheduler implemented in Go language. For more information, please follow other related articles on the PHP Chinese website!
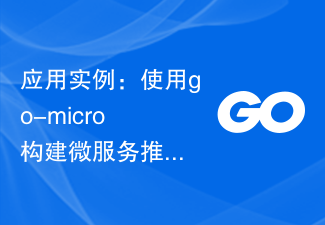
随着互联网应用的普及,微服务架构已成为目前比较流行的一种架构方式。其中,微服务架构的关键就是将应用拆分为不同的服务,通过RPC方式进行通信,实现松散耦合的服务架构。在本文中,我们将结合实际案例,介绍如何使用go-micro构建一款微服务推荐系统。一、什么是微服务推荐系统微服务推荐系统是一种基于微服务架构的推荐系统,它将推荐系统中的不同模块(如特征工程、分类
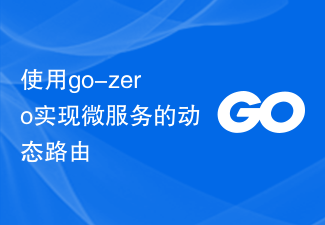
随着云计算和容器化技术的普及,微服务架构已成为现代化软件开发中的主流方案。而动态路由技术则是微服务架构中必不可少的一环。本文将介绍如何使用go-zero框架实现微服务的动态路由。一、什么是动态路由在微服务架构中,服务的数量和种类可能非常多,如何管理和发现这些服务是一项非常棘手的任务。传统的静态路由并不适用于微服务架构,因为服务数量以及运行时的状态都是动态变化
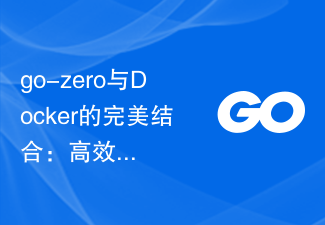
随着互联网的快速发展,微服务架构渐渐成为了业界的热门话题,而Docker作为容器化的利器,更是被广泛应用于微服务架构中的部署和运维。而今天我要介绍的是另一款非常优秀的微服务框架——go-zero,以及它与Docker的完美结合。一、什么是go-zerogo-zero是一款由饿了么点评公司开源的,基于Go语言构建的微服务框架。它的特点是高性能、易于使用和功能全
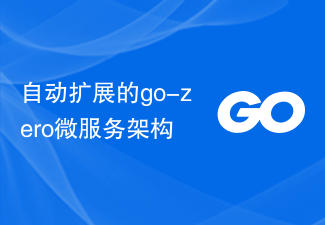
近年来,随着云计算和微服务架构的普及,越来越多的企业和开发者开始使用微服务架构来搭建自己的应用。然而,微服务架构也存在着一些问题,比如服务的扩展、管理、监控等方面。为了解决这些问题,很多开发者开始使用go-zero微服务框架。go-zero是一款基于Go语言开发的微服务框架,它提供了一系列的组件和工具,帮助开发者快速构建、管理和扩展自己的微服务。其中最重要的
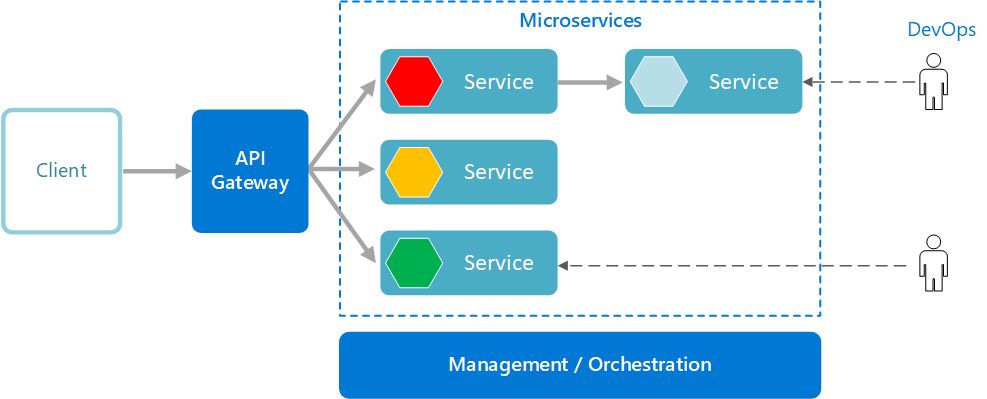
在选择适合微服务架构的编程语言时,Python是其中一种选择。它具有活跃的社区、更好的原型设计以及在开发人员中受欢迎等好处。它有一些限制,因此可以使用其他语言来避免它们。快速开发架构风格回顾与统计两种主要的开发架构风格是单体架构和微服务架构。Monolithic具有一体化的原则,并作为一个整体结构发挥作用,最适合小型开发项目或初创企业。当一个平台增长并且业务需要复杂的应用程序时,将其拆分为微服务架构是合理的。一些语言和框架更适合构建微服务架构。Java、Javascript和Python被列为微
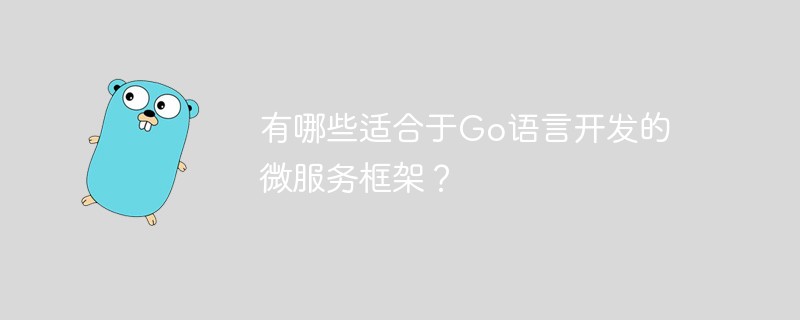
随着微服务架构的兴起,越来越多的开发者开始探索如何将应用程序拆分成小而独立的服务,并将它们组合成一个更大的应用。Go语言因其高效、简洁和并发性能出色的特点,成为了其中一个热门的用于微服务开发的语言。而本文将介绍一些适合于Go语言开发的微服务框架。GinGin是一款快速、灵活和轻量级的Web框架,具有丰富的功能和优雅的API。它通过HTTP路由机制和中间件来帮
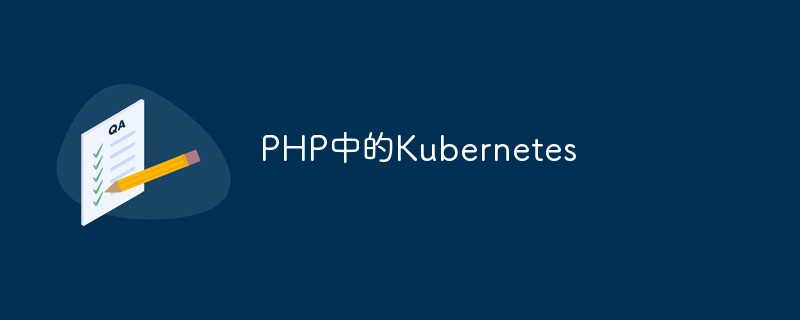
Kubernetes是近年来非常火热的容器编排和管理工具,PHP作为一种非常流行的Web开发语言,也需要适应这个趋势,通过Kubernetes来管理自己的应用。在本文中,我们将探讨如何在PHP应用中使用Kubernetes。一、Kubernetes概述Kubernetes是由Google公司开发的一个容器编排和管理工具,用于管理容器化应用。Kubernete
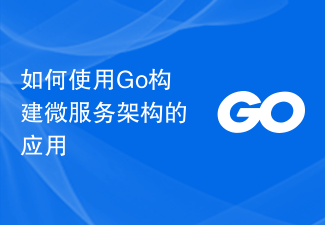
随着软件开发的不断发展,微服务架构已经逐渐成为了一种非常流行的架构模式。而在微服务架构中,Go语言作为一种高性能的编程语言也逐渐受到了越来越多的关注。那么,如何使用Go构建微服务架构的应用呢?下面将通过几个步骤来详细介绍。1.选择合适的Go框架选择合适的Go框架非常重要,它能够让我们更快地构建出一些基础服务,比如HTTP服务、日志服务、数据库服务等等。当前,


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
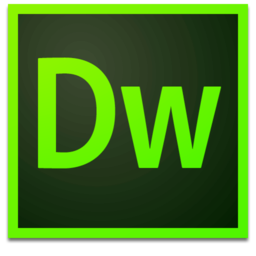
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
