


Thread pool optimization solution in PHP high concurrency processing
With the rapid development of the Internet and the continuous growth of user needs, high concurrency has become a key issue in modern Web application development. an important question. In PHP, handling high concurrent requests is a challenge due to its single-threaded nature. In order to solve this problem, introducing the concept of thread pool is an effective optimization solution.
Thread pool is a reusable collection of threads used to perform a large number of concurrent tasks. Its basic idea is to separate the creation, destruction and management of threads and reduce system overhead by reusing threads. In PHP, we can leverage multi-process extensions to implement thread pools. Let's take a look at how to use thread pools to optimize high-concurrency processing.
First, we need to install the pthreads extension, which is a multi-threaded extension for PHP. It can be installed through the following command:
pecl install pthreads
After the installation is complete, add the following configuration in the php.ini file:
extension=pthreads.so
In this example, we will use a simple task queue to simulate Handling of high concurrent requests. First, we define a Task
class to encapsulate the logic of the task:
class Task extends Threaded { private $url; public function __construct($url) { $this->url = $url; } public function run() { // 处理任务逻辑,这里以发送HTTP请求为例 $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $this->url); curl_exec($ch); curl_close($ch); } }
Next, we define a ThreadPool
class to manage the creation of the thread pool And task scheduling:
class ThreadPool { private $threadCount; private $pool; public function __construct($threadCount) { $this->threadCount = $threadCount; $this->pool = new Pool($this->threadCount); } public function dispatch($task) { $this->pool->submit($task); } public function wait() { $this->pool->shutdown(); } }
In the above code, we use the Pool
class to create a thread pool and submit tasks to the thread pool through the submit
method. shutdown
The method is used to wait for all tasks to be executed and close the thread pool.
Finally, we can test the effect of the thread pool through the following code example:
$urls = [ 'https://example.com/1', 'https://example.com/2', 'https://example.com/3', // 更多URL... ]; $threadPool = new ThreadPool(5); // 创建一个5个线程的线程池 foreach ($urls as $url) { $task = new Task($url); $threadPool->dispatch($task); // 提交任务到线程池中 } $threadPool->wait(); // 等待任务执行完成 echo "All tasks completed!";
In the above example, we created a thread pool containing 5 threads and submitted several Task. The thread pool automatically schedules the execution of tasks until all tasks are completed.
By using the thread pool, we can greatly improve the processing efficiency of high concurrent requests. Multiple tasks can be executed concurrently, reducing waiting time and reducing the load on the server.
In actual applications, we can adjust the size of the thread pool according to specific business needs and server performance to obtain the best performance optimization effect.
To sum up, the thread pool is an effective optimization solution for handling high concurrent requests in PHP. By properly using thread pools, we can improve the concurrent processing capabilities of web applications, improve user experience, and reduce server load pressure.
The above is the detailed content of Thread pool optimization solution in PHP high concurrency processing. For more information, please follow other related articles on the PHP Chinese website!
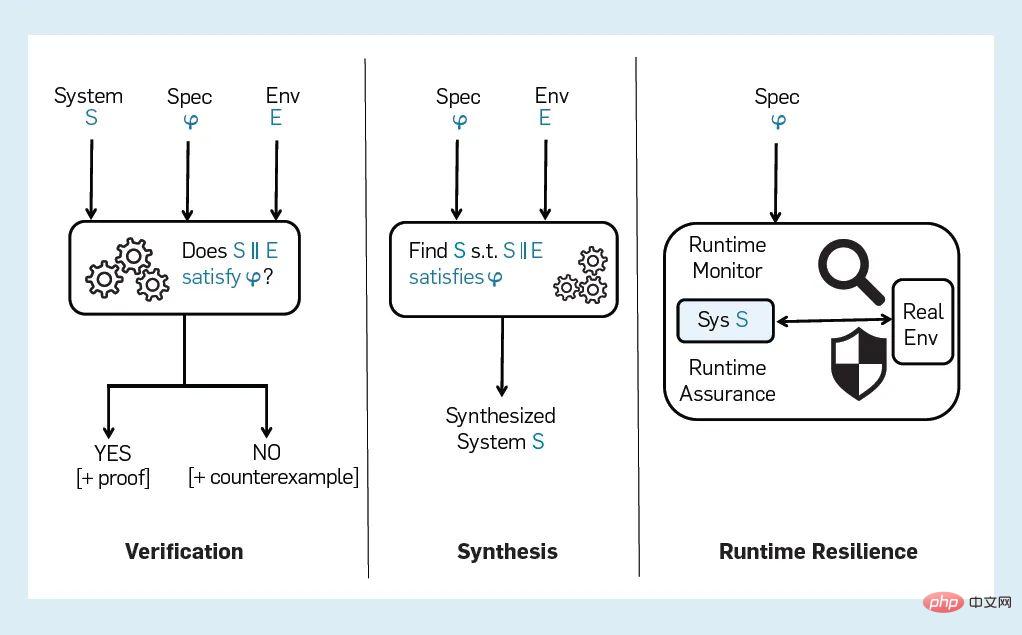
人工智能试图模仿人类智能的计算系统,包括人类一些与智能具有直观联系的功能,例如学习、解决问题以及理性地思考和行动。在广义地解释上,AI 一词涵盖了许多密切相关的领域如机器学习。那些大量使用 AI 的系统在医疗保健、交通运输、金融、社交网络、电子商务和教育等领域都产生了重大的社会影响。这种日益增长的社会影响,也带来了一系列风险和担忧,包括人工智能软件中的错误、网络攻击和人工智能系统安全等方面。因此,AI 系统的验证问题以及更广泛的可信 AI 的话题已经开始引起研究界的关注。“可验证 AI”已经被确
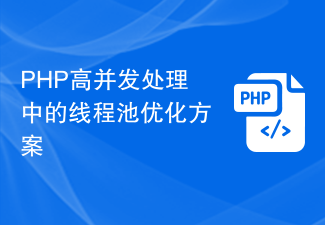
PHP高并发处理中的线程池优化方案随着互联网的快速发展和用户需求的不断增长,高并发成为了现代Web应用开发中的一个重要问题。在PHP中,由于其单线程的特性,处理高并发请求是一项挑战。为了解决这个问题,引入线程池的概念是一个有效的优化方案。线程池是一种可重复利用的线程集合,用于执行大量的并发任务。它的基本思想是将线程的创建、销毁和管理分离出来,通过复用线程来减
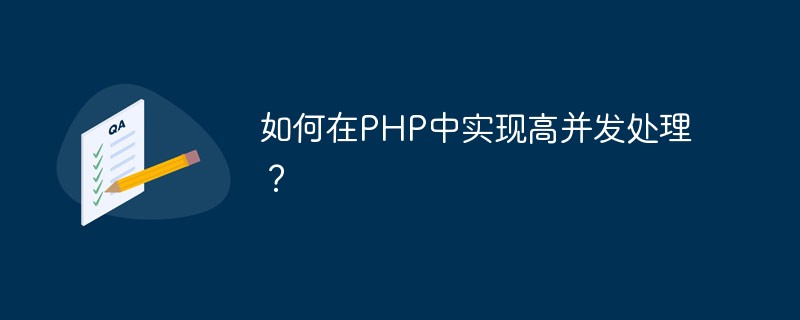
随着互联网的快速发展,Web应用程序也变得越来越受欢迎。在这些应用程序中,高并发性能是至关重要的。PHP是一种流行的服务器端脚本语言,可以用于开发Web应用程序。在本篇文章中,我们将讨论如何在PHP中实现高并发处理。什么是高并发处理?高并发处理是指能够处理大量并发请求的能力。在Web应用程序中,许多用户同时尝试访问同一资源,如数据库、文件存储或计算资源。当访
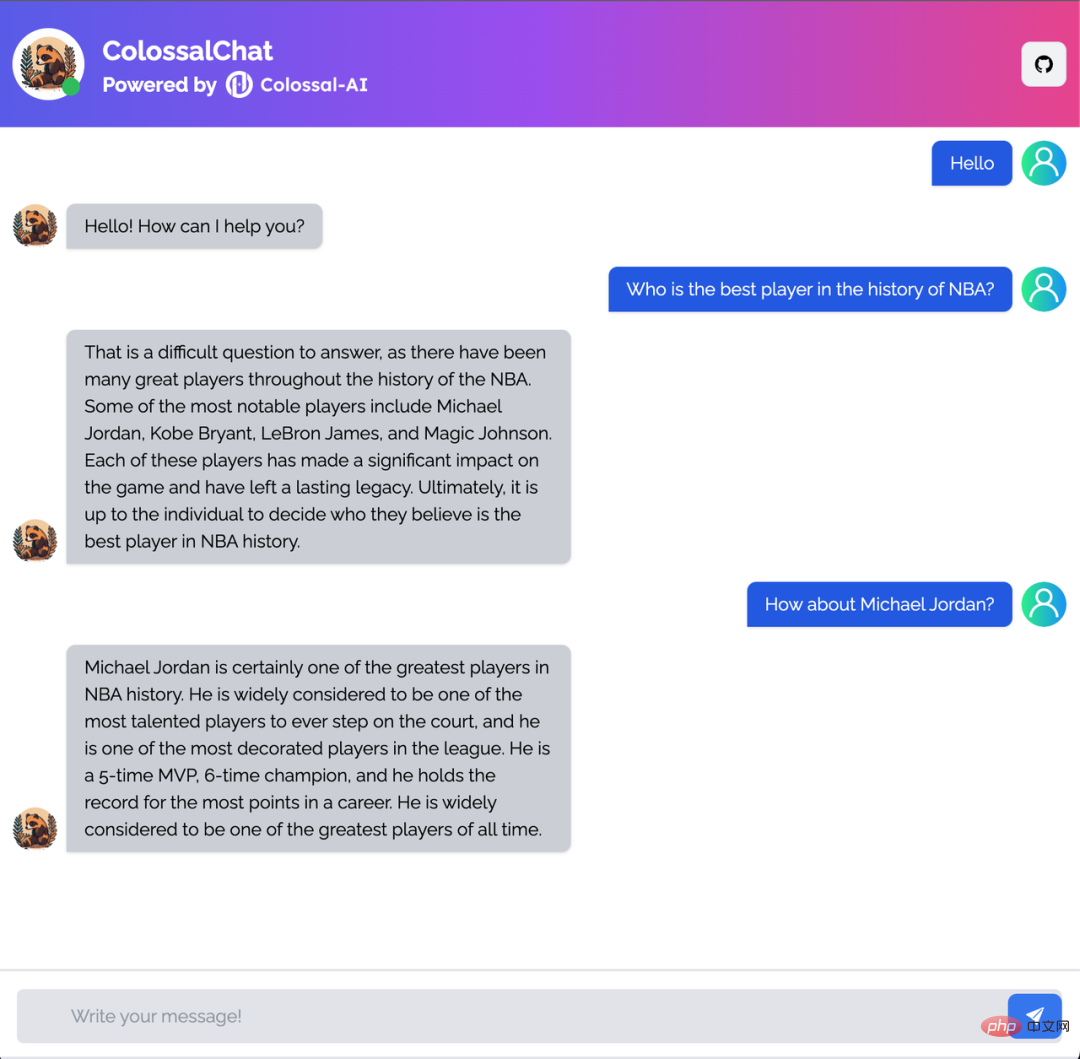
以ChatGPT、GPT4为代表的AI应用和大模型火爆全球,被视为开启了新的科技工业革命和AGI(通用人工智能)的新起点。不仅科技巨头间你追我赶,争相推出新品,许多学术界、工业界的AI大佬也纷纷投入投身相关创业浪潮。生成式AI正以“天”为单位,快速迭代,持续狂飙!然而,OpenAI并未将其开源,它们背后的技术细节有哪些?如何快速跟进、追赶并参与到此轮技术浪潮中?如何降低AI大模型构建和应用的高昂成本?如何保护核心数据与知识产权不会因使用第三方大模型API外泄?作为当下最受
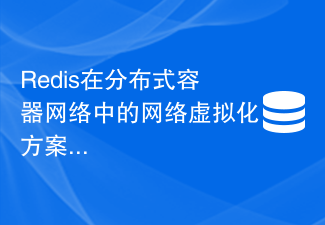
Redis是一个高性能的键值对存储系统,常用于数据缓存、会话存储和消息传递等场景,以其性能优异和方便易用的特性受到广泛关注。在容器化业务越来越普及的今天,如何将Redis应用于容器化场景中,是一个亟待解决的问题。其中,网络虚拟化技术是实现Redis在容器中部署的关键。容器化业务中的网络虚拟化在容器化业务中,容器是一个轻量级的虚拟化技术,可以在同一主机上承载多
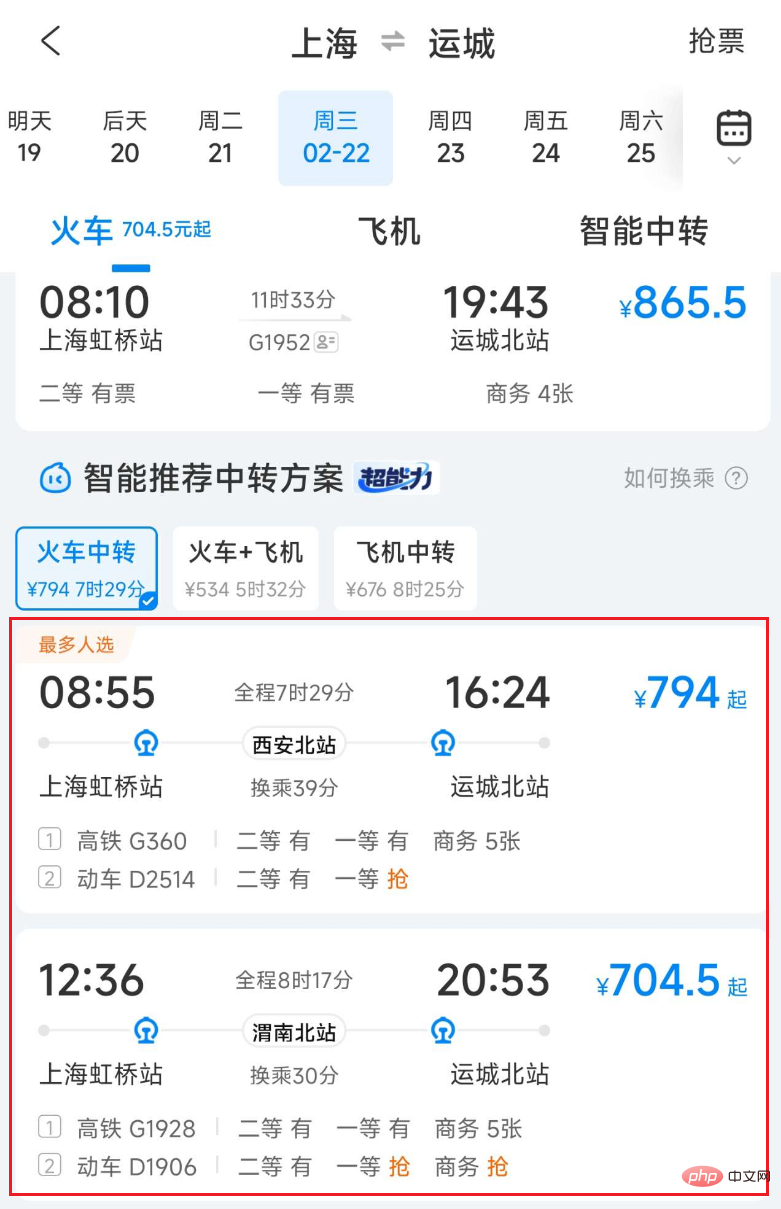
作者简介简言,携程后端开发经理,关注技术架构、性能优化、交通规划等领域。一、背景介绍由于交通规划和运力资源的限制,用户查询的两地之间可能没有直达交通,或者在重大节假日时,直达交通都已售罄。不过,通过火车、飞机、汽车、船舶等两程或多程中转的方式,用户仍然可以到达目的地。此外,中转交通有时在价格和耗时方面更具有优势。例如,对于从上海到运城,通过火车中转可能比直达火车更加快捷和便宜。图1携程火车中转交通列表在提供中转交通方案时,很重要的一个环节是将两程或多程的火车、飞机、汽车、船舶等拼接起来组成可行的
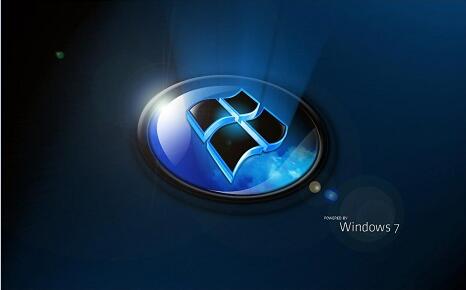
我们在安装新系统的时候,经常会遇到需要分区的情况,很多人不知道c盘应该分多少。一般来说,c盘50g就完全够用了,而且部分系统在安装时会自带分区方案,按照那个来就可以。如果有ssd的话,可以直接将ssd设置为c盘。win7500g硬盘c盘分多少答:c盘一般作为系统盘,还可以放一些常用软件,推荐50g或者100g。1、其实硬盘分区主要是为了使用方便,尤其是在找系统文件之类文件的情况下更容易寻找。2、一般来说我们都把c盘当做系统盘,所以可以把常用软件和系统放在c盘,可以3、剩下根据我们的个人需求进行分
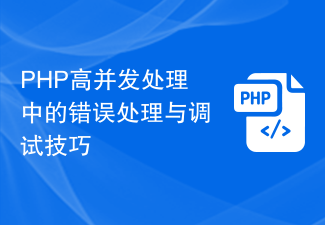
PHP高并发处理中的错误处理与调试技巧在现代互联网应用中,高并发是一个常见的挑战。PHP作为一种广泛使用的服务器端编程语言,也面临着高并发的压力。在处理高并发时,错误处理和调试是必不可少的技巧。本文将介绍几种在PHP高并发处理中常用的错误处理和调试技巧,并附上相应的代码示例。使用异常处理在高并发处理中,错误的发生是无法避免的。为了更好地处理错误,可以使用PH


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
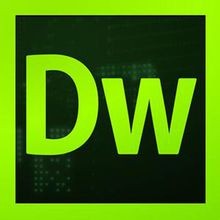
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
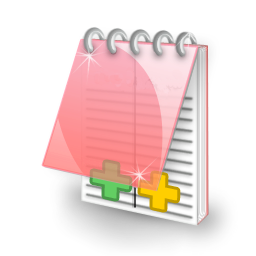
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!
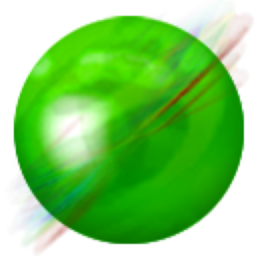
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
