Building a real-time chat room based on JavaScript
With the rapid development of the Internet, people are paying more and more attention to instant messaging and real-time interactive experience. As a common instant messaging tool, real-time chat rooms are very important to both individuals and businesses. This article will introduce how to build a simple real-time chat room using JavaScript and provide corresponding code examples.
We first need a front-end page as the UI interface of the chat room. Here is a simple HTML structure example:
<!DOCTYPE html> <html> <head> <title>实时聊天室</title> <style> #messages { height: 400px; overflow: scroll; border: 1px solid grey; } </style> </head> <body> <div id="messages"></div> <input type="text" id="input" placeholder="输入消息"> <button onclick="sendMessage()">发送</button> </body> </html>
In the above code, we have created a <div> element to display the message and set a fixed height and scrollbar style . Next, we added a text box and a button where the user can type a message and a button to send it. <p>Next, we need to write the corresponding JavaScript code to handle the logic of the real-time chat room. The following is a simple implementation example: </p><pre class='brush:php;toolbar:false;'>// 创建一个WebSocket连接
const socket = new WebSocket('ws://localhost:3000');
// 当连接建立时执行
socket.onopen = function(event) {
console.log('已连接到服务器');
};
// 当收到服务器消息时执行
socket.onmessage = function(event) {
const messages = document.getElementById('messages');
const message = document.createElement('div');
message.innerText = event.data;
messages.appendChild(message);
// 滚动到最底部
messages.scrollTop = messages.scrollHeight;
};
// 发送消息
function sendMessage() {
const input = document.getElementById('input');
const message = input.value;
socket.send(message);
input.value = '';
}</pre><p> In the above code, we use the WebSocket API in JavaScript to establish a real-time connection with the server. When the connection is successfully established, we will receive an <code>onopen
event. When a message is received from the server, the onmessage
event will be triggered. We will add the received message to the message display area and automatically scroll to the bottom by setting the scroll bar position.
Finally, we need to create a WebSocket server on the server side to process and forward messages. Here is an example using Node.js and the WebSocket library:
const WebSocket = require('ws'); // 创建WebSocket服务器 const wss = new WebSocket.Server({ port: 3000 }); // 当有新的WebSocket连接建立时执行 wss.on('connection', function(ws) { console.log('有新的连接'); // 当收到消息时执行 ws.on('message', function(message) { console.log('收到消息: ' + message); // 将消息广播给所有连接的客户端 wss.clients.forEach(function(client) { client.send(message); }); }); });
In the above code, we have used the WebSocket library to create a WebSocket server. When a new connection is established, the connection
event will be triggered. When a message is received, the message
event will be triggered, and we will broadcast the received message to all connected clients.
With the above simple code example, we can implement a real-time chat room based on JavaScript. When the user enters a message and clicks the send button, the message is sent to the server through the WebSocket connection and forwarded by the server to all connected clients. After the client receives the message, it displays it in the UI interface. The whole process realizes the function of real-time communication.
Of course, the above example is just a simple implementation. In an actual real-time chat room, other functions such as disconnection and reconnection, user authentication, private chat, etc. need to be handled. I hope this article can provide you with a basic idea and code example to help you build your own real-time chat room.
The above is the detailed content of Build a real-time chat room based on JavaScript. For more information, please follow other related articles on the PHP Chinese website!
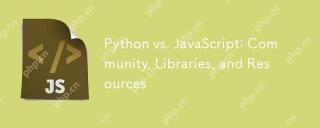
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
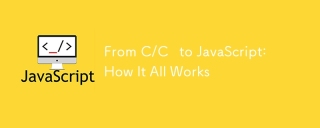
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
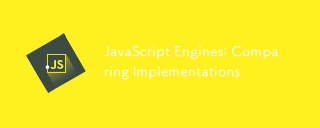
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
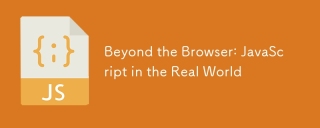
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
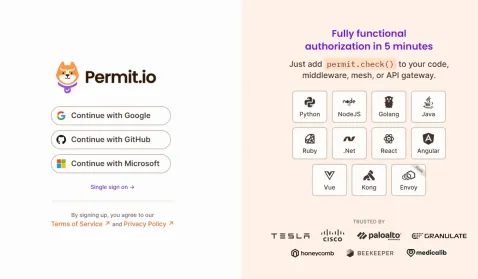
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
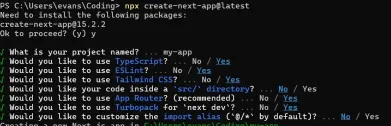
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
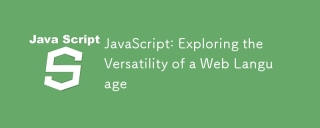
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
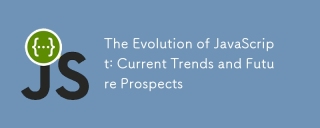
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
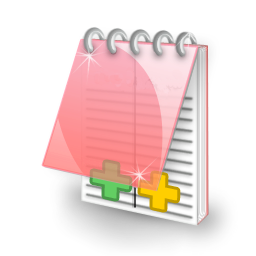
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
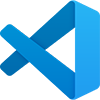
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software