Analyzing Vue's server-side communication skills: how to avoid data loss
Analysis of Vue’s server-side communication skills: How to avoid data loss
Vue is a popular JavaScript framework that provides excellent front-end data binding and Componentization capabilities. In Vue applications, communicating with the server is a very common need. However, due to network delays, server errors and other reasons, abnormalities may occur during data sending and receiving, and even data loss may occur. This article will discuss some tips to help you avoid data loss issues in Vue applications.
- Use Promise to handle asynchronous requests
In Vue, tool libraries such as Axios or Fetch are usually used to send asynchronous requests. However, when handling asynchronous requests, we may encounter data loss issues due to network delays or server errors. To solve this problem, we can use Promise to handle asynchronous requests and use the catch method to catch exceptions.
axios.get('/api/data') .then(response => { // 处理后续逻辑 }) .catch(error => { // 处理异常情况 });
By using Promise instead of callback functions, we can better handle exceptions of asynchronous requests and avoid data loss problems.
- Using the retry mechanism of Axios
Axios is a very popular Promise-based HTTP client library. It provides a built-in retry mechanism to help us deal with Network request failure. By setting the maxRetry times and retryDelay delay time, we can automatically retry when the request fails.
axios.get('/api/data', { retry: { maxRetry: 3, retryDelay: 1000 } }) .then(response => { // 处理后续逻辑 }) .catch(error => { // 处理异常情况 });
Using Axios' retry mechanism can help us maintain data integrity in the event of network instability or server errors.
- Using WebSocket for real-time communication
In some scenarios, we need to achieve real-time communication and ensure data integrity. WebSocket is a TCP-based communication protocol that provides a two-way communication channel that can maintain a long-lasting connection and transmit data in real time. Vue can communicate with the server in real time through WebSocket to ensure the timeliness and integrity of data.
const socket = new WebSocket('ws://localhost:8080'); socket.onopen = event => { // 连接建立成功 }; socket.onmessage = event => { const message = JSON.parse(event.data); // 处理接收到的数据 }; socket.onclose = event => { // 连接关闭 }; socket.onerror = event => { // 处理错误情况 };
By using WebSocket, real-time communication can be achieved in Vue applications and data loss due to network delays or other abnormal conditions can be avoided.
When handling server-side communication in Vue applications, we must be aware of the possibility of data loss. By using Promise to handle asynchronous requests, using Axios' retry mechanism, and using WebSocket for real-time communication, we can effectively avoid data loss problems. These tips can help us build a stable and reliable server communication system in Vue applications.
Note: The code examples in this article are for reference only, please make appropriate adjustments and modifications according to actual needs.
The above is the detailed content of Analyzing Vue's server-side communication skills: how to avoid data loss. For more information, please follow other related articles on the PHP Chinese website!

Vue.js improves user experience through multiple functions: 1. Responsive system realizes real-time data feedback; 2. Component development improves code reusability; 3. VueRouter provides smooth navigation; 4. Dynamic data binding and transition animation enhance interaction effect; 5. Error processing mechanism ensures user feedback; 6. Performance optimization and best practices improve application performance.

Vue.js' role in web development is to act as a progressive JavaScript framework that simplifies the development process and improves efficiency. 1) It enables developers to focus on business logic through responsive data binding and component development. 2) The working principle of Vue.js relies on responsive systems and virtual DOM to optimize performance. 3) In actual projects, it is common practice to use Vuex to manage global state and optimize data responsiveness.

Vue.js is a progressive JavaScript framework released by You Yuxi in 2014 to build a user interface. Its core advantages include: 1. Responsive data binding, automatic update view of data changes; 2. Component development, the UI can be split into independent and reusable components.

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 English version
Recommended: Win version, supports code prompts!
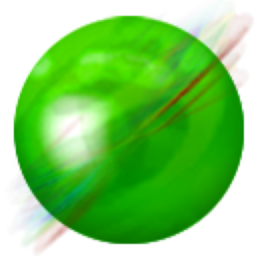
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool