


Analysis of Vue’s server-side communication optimization: how to reduce network latency
In recent years, with the rapid development of the Internet, performance optimization of web applications has become a design and an integral part of the development process. Among them, server-side communication optimization is one of the key factors to reduce network latency and improve user experience. This article will focus on the Vue framework and analyze how to optimize server-side communication in terms of reducing the number of HTTP requests, compressing files, and using cache to achieve faster loading speeds and better user experience.
1. Reduce the number of HTTP requests
In a web application, each HTTP request will cause a certain delay. Therefore, reducing the number of HTTP requests is one of the effective ways to reduce network latency.
In Vue, you can reduce the number of HTTP requests by using lazy loading of routes. By splitting the components corresponding to different routes into independent files and lazily loading them when needed, on-demand loading can be achieved and the number of HTTP requests during initial page loading can be reduced. The following is a sample code:
const Home = () => import('@/views/Home.vue') const About = () => import('@/views/About.vue') const Contact = () => import('@/views/Contact.vue') const routes = [ { path: '/', name: 'home', component: Home }, { path: '/about', name: 'about', component: About }, { path: '/contact', name: 'contact', component: Contact } ] const router = new VueRouter({ routes })
2. Compressed files
The size of network transmission is also one of the important factors affecting network delay. In Vue applications, you can reduce the size of network transfers by compressing files.
Vue provides a webpack plug-in - CompressionWebpackPlugin, which can be used to Gzip compress static resources. We can use this plug-in in the configuration file of the Vue project to compress the packaged files to reduce the network transmission size. The following is a sample code:
// vue.config.js const CompressionWebpackPlugin = require('compression-webpack-plugin') module.exports = { configureWebpack: { plugins: [ new CompressionWebpackPlugin({ test: /.js$|.css$/, threshold: 10240, deleteOriginalAssets: false }) ] } }
3. Use caching
In server-side communication, caching can reduce the number of requests, thereby reducing network latency. Vue provides an http library called axios that can communicate with the server. We can use browser caching by setting the caching policy in the request header.
The following is a sample code:
import axios from 'axios' axios.get('/api/data', { headers: { 'Cache-Control': 'max-age=3600' } })
In the above code, by setting the value of the Cache-Control field in the request header to max-age=3600, tell the browser the cache of the request The validity period is 3600 seconds.
By reducing the number of HTTP requests, compressing files, and using caching, you can optimize the server-side communication of your Vue application, thereby reducing network latency and improving page loading speed and user experience. In actual development, we can also choose other optimization strategies based on specific needs. But no matter what optimization method is used, reasonable server-side communication optimization can provide users with a faster and smoother web experience.
The above is the detailed content of Analyzing Vue's server-side communication optimization: how to reduce network latency. For more information, please follow other related articles on the PHP Chinese website!
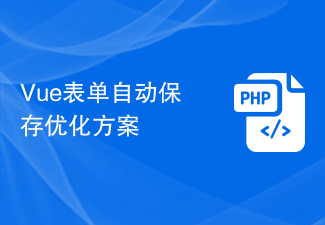
如何优化Vue开发中的表单自动保存问题在Vue开发中,表单自动保存是一个常见的需求。当用户在填写表单时,我们希望能够在用户离开页面或者关闭浏览器时自动保存表单数据,以免用户的输入信息丢失。本文将介绍如何通过优化来解决Vue开发中的表单自动保存问题。使用Vue组件的生命周期钩子函数Vue组件的生命周期钩子函数提供了在组件生命周期中执行自定义逻辑的能力。我们可以
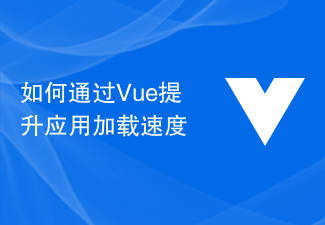
如何通过Vue提升应用加载速度Vue是一款流行的JavaScript框架,用于构建用户界面。Vue拥有丰富的功能和强大的性能优化选项,可以帮助我们提升应用的加载速度。本文将介绍一些通过Vue可以使用的优化技巧,以加快应用的加载速度。使用CDN引入Vue:将Vue引入为外部资源的方式,可以利用CDN来提高加载速度。在HTML文件的头部,通过以下代码引入Vue:
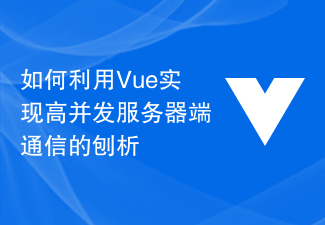
如何利用Vue实现高并发服务器端通信的刨析在现代互联网应用程序中,高并发服务器端通信是一个非常重要的话题。当大量用户同时访问一个网站或者应用程序时,服务器需要处理大量的请求,并保持与多个客户端的稳定和快速的通信。Vue是一种流行的JavaScript框架,它提供了一种简单而强大的方式来构建前端界面。但是,在处理高并发服务器端通信时,Vue的一些默认设置可能会
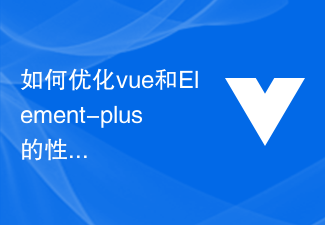
如何优化Vue和ElementPlus的性能,提升网页加载速度在现代Web应用程序中,性能是一个非常重要的考量因素。当我们使用Vue.js和ElementPlus构建应用程序时,我们需要确保网页的加载速度快,以提供用户良好的体验。本文将介绍一些优化Vue和ElementPlus性能的方法,并提供相应的代码示例。使用生产环境构建在部署Web应用程序之前,
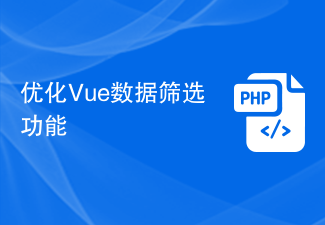
如何优化Vue开发中的数据筛选功能问题在Vue开发中,数据筛选是一个常见的需求。无论是展示数据给用户,还是根据用户的选择来进行数据过滤,数据筛选功能都是一个必不可少的组成部分。然而,在处理大量数据或复杂的筛选条件时,数据筛选功能可能会面临性能问题。本文将介绍一些优化Vue开发中的数据筛选功能的方法,帮助开发者提升应用的性能和用户体验。使用计算属性进行数据筛选
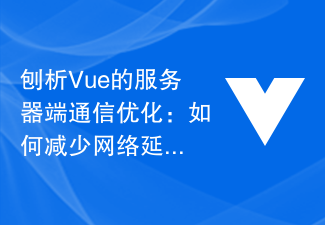
刨析Vue的服务器端通信优化:如何减少网络延迟近年来,随着互联网的快速发展,web应用程序的性能优化已经成为设计和开发过程中不可或缺的一部分。其中,服务器端通信优化是减少网络延迟和提升用户体验的关键因素之一。本文将围绕Vue框架,从减少HTTP请求数、压缩文件以及使用缓存等方面分析如何优化服务器端通信,以实现更快的加载速度和更好的用户体验。一、减少HTTP请
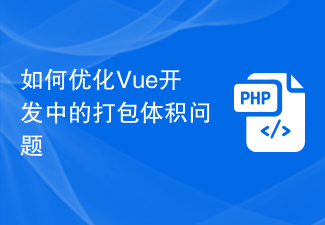
如何优化Vue开发中的打包体积问题随着前端技术的不断发展,越来越多的开发者选择使用Vue作为前端框架来开发项目。Vue具有简单易用、高效灵活的特点,深受开发者的喜爱。然而,在Vue开发中,打包体积问题是一个常见的挑战,特别是在大型项目中。本文将介绍一些优化Vue开发中的打包体积问题的方法,帮助开发者提高项目的性能和用户体验。使用ES6模块化ES6的模块化系统
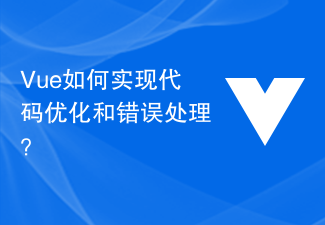
在Vue应用程序的开发过程中,除了实现功能和用户交互体验外,代码的性能和稳定性也是非常重要的。因此,为了让Vue应用程序更加高效、稳定和易于维护,需要对其进行一定的代码优化和错误处理。本篇文章将介绍Vue如何实现代码优化和错误处理。一、优化Vue应用程序使用vue-cli构建应用程序Vue框架提供了vue-cli脚手架工具,可以快速创建Vue应用程序和组件


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
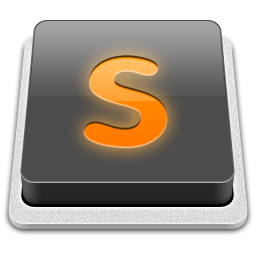
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
