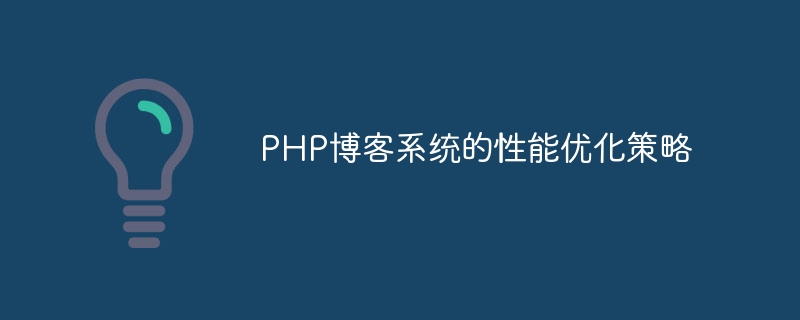
Performance Optimization Strategy of PHP Blog System
With the continuous development of the Internet, blogs have become an important tool for people to record and share their lives. As a blog system developed based on PHP, performance optimization is an important aspect to ensure user experience and improve system carrying capacity. This article will introduce some performance optimization strategies for PHP blog systems and give code examples.
- Using cache
Cache is an effective way to improve system performance. In the blog system, we can use cache to store some frequently accessed data, such as article lists, popular articles, etc. PHP provides a variety of caching technologies, such as Memcached, Redis, etc. The following is a code example that uses Memcached to cache an article list:
// 获取文章列表
function getArticleList() {
$cacheKey = 'article_list';
$articleList = memcached_get($cacheKey);
if (!$articleList) {
// 从数据库中获取文章列表
$articleList = getArticlesFromDB();
// 将文章列表存入缓存
memcached_set($cacheKey, $articleList, $expireTime);
}
return $articleList;
}
- Database Optimization
The database is the core storage component of the blog system. Optimizing the database can significantly improve the performance of the system. The following lists some common database optimization strategies:
- Reasonably design the database structure to avoid redundancy and repeated design.
- Choose appropriate indexes to improve database query efficiency.
- To avoid frequent database queries, you can use caching or load some commonly used data into memory in advance.
- Perform batch operations to reduce the number of database connections and queries.
- Front-end optimization
The front-end is the first layer of interface that users come into contact with. By optimizing the front-end, the page loading speed of the blog system can be improved. Here are some front-end optimization strategies:
- Merge and compress CSS and JS files to reduce page load time.
- Use CSS Sprites to reduce the number of image requests.
- Use asynchronous loading technology, such as Ajax, to reduce page blocking.
- Compress page content and reduce transfer size.
- Asynchronous processing
In the blog system, some operations may be time-consuming, such as sending emails, generating image thumbnails, etc. In order not to block user requests, asynchronous processing can be used to improve the system's concurrency and response speed. The following is a code example that uses message queues to implement asynchronous processing:
// 发送电子邮件
function sendEmail($email) {
// 将邮件信息发送到消息队列中
message_queue_push('email_queue', $email);
}
// 异步处理邮件队列
function handleEmailQueue() {
while (true) {
$email = message_queue_pop('email_queue');
// 发送邮件
sendEmail($email);
}
}
- Cache database query results
For some frequently accessed database query results, you can cache them to Reduce the number of database accesses. The following is a code example that uses Redis to cache database query results:
// 获取文章详情
function getArticleDetail($id) {
$cacheKey = 'article_detail:' . $id;
$articleDetail = redis_get($cacheKey);
if (!$articleDetail) {
// 从数据库中获取文章详情
$articleDetail = getArticleDetailFromDB($id);
// 将文章详情存入Redis缓存
redis_set($cacheKey, $articleDetail, $expireTime);
}
return $articleDetail;
}
The above are some optimization strategies and code examples to improve the performance of the PHP blog system. Through the rational use of caching, database optimization, front-end optimization, asynchronous processing and caching database query results, the performance and user experience of the blog system can be greatly improved. I hope this article will be helpful to developers and practical applications.
The above is the detailed content of Performance optimization strategies for PHP blog system. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn