


How to use Vue form processing to implement character replacement in form fields
How to use Vue form processing to implement character replacement in form fields
When developing web applications, forms are an essential part. In some scenarios, we may need to replace characters entered by the user to meet data format requirements or implement certain functions. As a popular front-end framework, Vue.js provides powerful data binding and processing capabilities, making form processing more convenient. This article will introduce how to use Vue.js to implement the character replacement function of form fields and provide code examples.
First, we need to create a new Vue instance and define the initial value of the form field and the replacement rules in the data attribute. Suppose we have a form field called inputContent, and we need to replace all spaces in it with horizontal lines. The code is as follows:
<div id="app"> <input v-model="inputContent" type="text"> <p>{{ replacedContent }}</p> </div>
new Vue({ el: '#app', data: { inputContent: '', replacedContent: '' } });
Next, we need to add a calculated property to the Vue instance to implement character replacement logic. Computed properties automatically update based on the form field's value and return the replaced result. The code is as follows:
new Vue({ el: '#app', data: { inputContent: '', replacedContent: '' }, computed: { replacedContent: function() { return this.inputContent.replace(/s/g, '-'); // 使用正则表达式将空格替换为横线 } } });
In the above code, we use the replace method in JavaScript and a regular expression /s/g to match spaces. After replacing spaces with dashes, the computed property returns the final replacement result.
Finally, we need to display the actual replacement results on the page. Through data binding, we can display the value of the calculated property replacedContent in the page. The code is as follows:
<div id="app"> <input v-model="inputContent" type="text"> <p>{{ replacedContent }}</p> </div>
After clicking Run, you will find that entering any characters in the input box will replace the spaces with horizontal lines and display them in the paragraph below. This is the basic process of using Vue.js to implement character replacement.
In addition to replacing spaces, we can also customize other character replacement rules according to actual needs. Various character replacement functions can be achieved by modifying regular expressions and replaced characters.
To sum up, Vue.js provides us with a convenient and fast way to process form data, including character replacement. By defining form fields, writing calculated properties and data binding, we can easily implement character replacement functions for form fields. I hope this article can help you better understand and apply the form processing capabilities of Vue.js.
The reference code is as follows:
<!DOCTYPE html> <html> <head> <title>Vue Form Character Replacement</title> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> </head> <body> <div id="app"> <input v-model="inputContent" type="text"> <p>{{ replacedContent }}</p> </div> <script> new Vue({ el: "#app", data: { inputContent: '', replacedContent: '' }, computed: { replacedContent: function() { return this.inputContent.replace(/s/g, '-'); // 使用正则表达式将空格替换为横线 } } }) </script> </body> </html>
This is a basic example, you can modify and extend it appropriately according to your project needs. Hope this article is helpful to you.
The above is the detailed content of How to use Vue form processing to implement character replacement in form fields. For more information, please follow other related articles on the PHP Chinese website!

The future trends and forecasts of Vue.js and React are: 1) Vue.js will be widely used in enterprise-level applications and have made breakthroughs in server-side rendering and static site generation; 2) React will innovate in server components and data acquisition, and further optimize the concurrency model.

Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
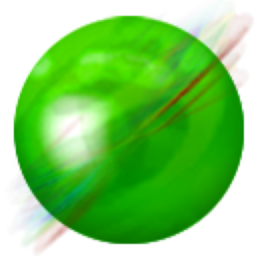
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!
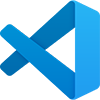
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
