


Analysis of Vue and server-side communication: how to handle timeout requests
Exploration of Vue and server-side communication: Methods of handling timeout requests
Introduction:
In the Vue development process, it is very difficult to communicate with the back-end server. Common situation. However, sometimes requests may time out due to network delays or other reasons. This article will discuss how to handle timeout requests in Vue and provide corresponding code examples.
1. Use Axios for requests
In Vue, we usually use Axios as the HTTP client library to make network requests. Axios provides a series of methods to send requests, and timeouts can be set. The following is a sample code that uses Axios to send a GET request and set the timeout:
import axios from 'axios'; axios.get('/api/data', { timeout: 5000 }) .then(response => { console.log(response.data); }) .catch(error => { if (error.code === 'ECONNABORTED') { console.log('请求超时'); } else { console.log('请求失败'); } });
In the above code, we set the timeout in milliseconds by setting the timeout attribute in the request configuration. If the request is not completed within the specified time, Axios will throw an error, and the code attribute value of the error object is 'ECONNABORTED', which we can use to determine whether the request has timed out.
2. Set the global timeout
In addition to setting the timeout in each request, we can also set the timeout globally in the Vue configuration. This way, the same timeout is applied to all requests sent through Axios. The following is a sample code for setting the global timeout:
import axios from 'axios'; axios.defaults.timeout = 5000;
In the above code, we set the global timeout by modifying the axios.defaults.timeout property. In this way, there is no need to set a timeout wherever HTTP requests need to be sent.
3. Handling timeout requests
When the request times out, we can handle this situation according to actual needs. Here are some common ways to handle timed out requests:
- Resend the request: We can try to resend the request to ensure the integrity of the data. Before resending the request, you can consider adding a retry counter to prevent repeated requests and waste of resources. The following is a sample code to resend a request:
import axios from 'axios'; function requestWithRetry(url, maxRetry) { return axios.get(url, { timeout: 5000 }) .then(response => { console.log(response.data); }) .catch(error => { if (error.code === 'ECONNABORTED' && maxRetry > 0) { return requestWithRetry(url, maxRetry - 1); } else { console.log('请求失败'); } }); } requestWithRetry('/api/data', 3);
In the above code, we define a requestWithRetry function, which will retry when the request times out, and the maximum number of retries is maxRetry . If the request exceeds the retry limit, "Request Failed" will be printed.
- Prompt the user for network exception: We can give the user a prompt on the page indicating that the request has timed out and may be due to network problems. The following is a sample code that prompts the user when the request times out:
axios.get('/api/data', { timeout: 5000 }) .then(response => { console.log(response.data); }) .catch(error => { if (error.code === 'ECONNABORTED') { alert('网络连接超时,请检查网络设置!'); } else { console.log('请求失败'); } });
In the above code, we use the alert function to pop up a prompt box to tell the user that the request has timed out and may be due to network problems. .
Conclusion:
This article introduces the method of handling timeout requests in Vue and provides corresponding code examples. Of course, in actual development, we need to decide how to handle timeout requests based on specific needs. Whether it is to resend the request or prompt the user for a network exception, the choice needs to be based on the actual situation. Only by properly handling timeout requests can the user experience and system stability be improved.
(Note: The above sample code is for demonstration purposes only. In actual application, please make corresponding adjustments according to project needs.)
The above is the detailed content of Analysis of Vue and server-side communication: how to handle timeout requests. For more information, please follow other related articles on the PHP Chinese website!

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.

Vue.js and React each have their own advantages: Vue.js is suitable for small applications and rapid development, while React is suitable for large applications and complex state management. 1.Vue.js realizes automatic update through a responsive system, suitable for small applications. 2.React uses virtual DOM and diff algorithms, which are suitable for large and complex applications. When selecting a framework, you need to consider project requirements and team technology stack.

Vue.js and React each have their own advantages, and the choice should be based on project requirements and team technology stack. 1. Vue.js is community-friendly, providing rich learning resources, and the ecosystem includes official tools such as VueRouter, which are supported by the official team and the community. 2. The React community is biased towards enterprise applications, with a strong ecosystem, and supports provided by Facebook and its community, and has frequent updates.

Netflix uses React to enhance user experience. 1) React's componentized features help Netflix split complex UI into manageable modules. 2) Virtual DOM optimizes UI updates and improves performance. 3) Combining Redux and GraphQL, Netflix efficiently manages application status and data flow.

Vue.js is a front-end framework, and the back-end framework is used to handle server-side logic. 1) Vue.js focuses on building user interfaces and simplifies development through componentized and responsive data binding. 2) Back-end frameworks such as Express and Django handle HTTP requests, database operations and business logic, and run on the server.

Vue.js is closely integrated with the front-end technology stack to improve development efficiency and user experience. 1) Construction tools: Integrate with Webpack and Rollup to achieve modular development. 2) State management: Integrate with Vuex to manage complex application status. 3) Routing: Integrate with VueRouter to realize single-page application routing. 4) CSS preprocessor: supports Sass and Less to improve style development efficiency.

Netflix chose React to build its user interface because React's component design and virtual DOM mechanism can efficiently handle complex interfaces and frequent updates. 1) Component-based design allows Netflix to break down the interface into manageable widgets, improving development efficiency and code maintainability. 2) The virtual DOM mechanism ensures the smoothness and high performance of the Netflix user interface by minimizing DOM operations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
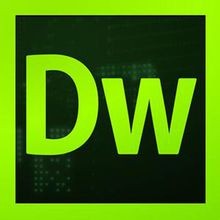
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
