Common problems and solutions to PHP data caching
Caching is one of the important means to improve the performance of web applications. In PHP development, rational use of data caching can significantly reduce access to external resources such as databases and APIs, thereby improving the response speed and scalability of the system. However, data caching also brings some common problems. This article will discuss these problems and provide corresponding solutions.
- Cache breakdown
Cache breakdown means that under high concurrency conditions, a certain cache key becomes invalid, and all concurrent requests directly access the database or other external resources, resulting in Resources are instantly overloaded. The way to solve this problem is to use a mutex lock to ensure that only one thread can update the cache, and other threads need to wait.
$key = 'cache_key'; $value = cache_get($key); if (!$value) { acquire_mutex_lock(); // 再次尝试从缓存中获取数据 $value = cache_get($key); if (!$value) { $value = fetch_data_from_database_or_api(); cache_set($key, $value); } release_mutex_lock(); }
- Cache penetration
Cache penetration refers to accessing data that does not exist in the cache, causing each request to directly access the database or other external resources, causing Database performance degrades. The solution to this problem is to use a Bloom Filter to filter out data that obviously does not exist before querying.
$key = 'cache_key'; $value = cache_get($key); if (!$value) { if (bloom_filter_contains($key)) { $value = fetch_data_from_database_or_api(); cache_set($key, $value); } else { $value = null; } }
- Cache avalanche
Cache avalanche means that within a certain period of time, a large number of cache keys expire at the same time, resulting in a large number of requests to directly access the database or other external resources, causing Resource bottlenecks or even collapse. The way to solve this problem is to set the cache expiration time to fluctuate randomly to avoid a large number of caches from invalidating at the same time.
$key = 'cache_key'; $value = cache_get($key); if (!$value) { acquire_mutex_lock(); // 再次尝试从缓存中获取数据 $value = cache_get($key); if (!$value) { $value = fetch_data_from_database_or_api(); // 设置缓存失效时间随机波动,避免大量缓存同时失效 cache_set($key, $value, random_expiration_time()); } release_mutex_lock(); }
- Cache update delay
After the data is updated, the data in the cache is not refreshed in time, resulting in inconsistent data obtained. The way to solve this problem is to actively update the cache when the data is updated.
function update_data($data) { // 更新数据库中的数据 update_database($data); // 清除缓存 $key = 'cache_key'; cache_delete($key); // 主动更新缓存 $value = fetch_data_from_database_or_api(); cache_set($key, $value); }
- Concurrent reading and writing issues with the same cache Key
When multiple processes or threads read and write the same cache Key at the same time, data exceptions or conflicts may occur. The way to solve this problem is to use distributed lock (Distributed Lock) to ensure that only one process or thread can perform write operations at the same time.
$key = 'cache_key'; if (acquire_distributed_lock($key)) { $value = cache_get($key); if (!$value) { $value = fetch_data_from_database_or_api(); cache_set($key, $value); } // 释放分布式锁 release_distributed_lock($key); } else { // 加锁失败,等待一段时间后重试或进行其他处理 }
Summary
Proper use of data cache can significantly improve the performance and scalability of web applications, but it will also bring about some common problems. We can effectively solve these problems by using technical means such as mutex locks, Bloom filters, random expiration times, proactively updated caches, and distributed locks. In actual development, choosing an appropriate solution based on specific circumstances can improve the stability and performance of the system.
The above is the detailed content of Common problems and solutions to PHP data caching. For more information, please follow other related articles on the PHP Chinese website!
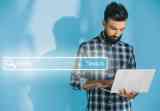
Long URLs, often cluttered with keywords and tracking parameters, can deter visitors. A URL shortening script offers a solution, creating concise links ideal for social media and other platforms. These scripts are valuable for individual websites a
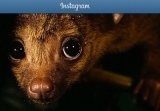
Following its high-profile acquisition by Facebook in 2012, Instagram adopted two sets of APIs for third-party use. These are the Instagram Graph API and the Instagram Basic Display API.As a developer building an app that requires information from a
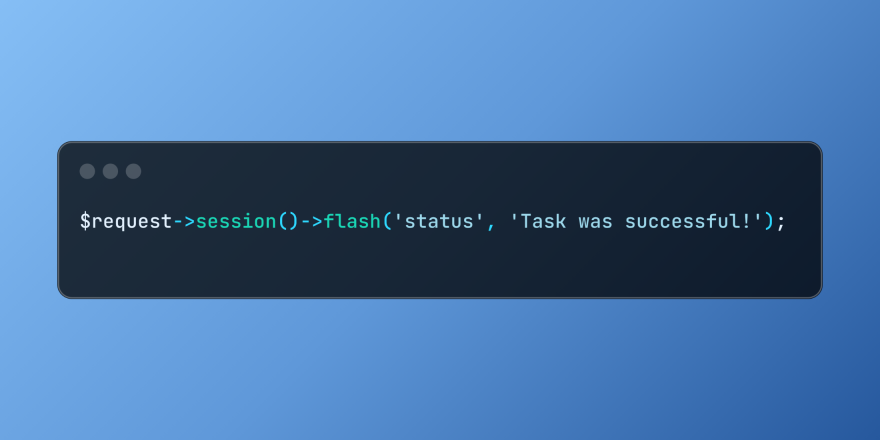
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
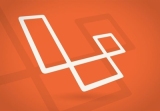
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
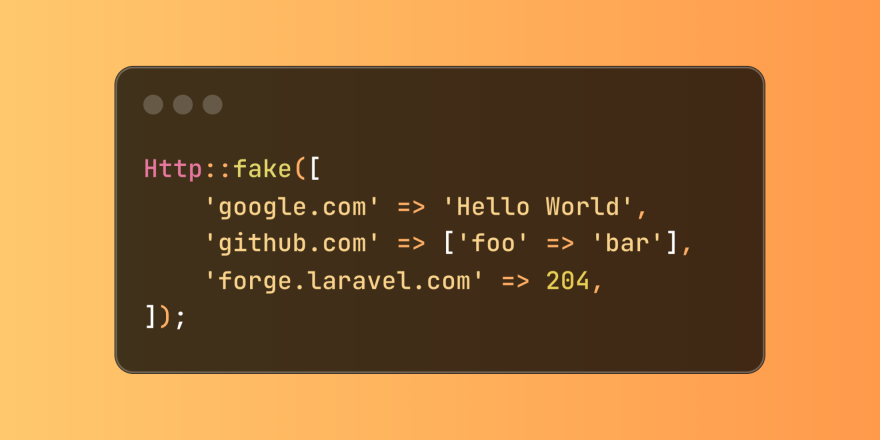
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
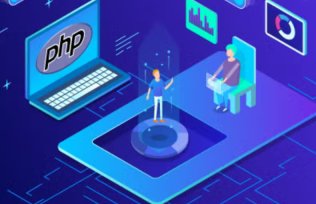
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
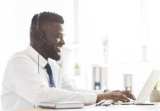
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
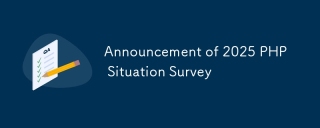
The 2025 PHP Landscape Survey investigates current PHP development trends. It explores framework usage, deployment methods, and challenges, aiming to provide insights for developers and businesses. The survey anticipates growth in modern PHP versio


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
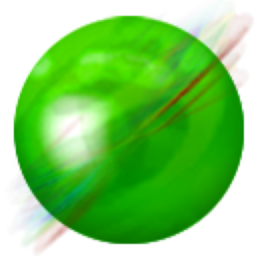
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
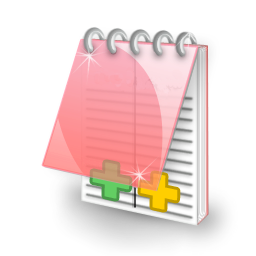
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
