Tips for using Golang for network request testing
Tips for using Golang for network request testing
Introduction:
When developing network applications, testing network requests is very important work segments. As a powerful programming language, Golang provides a wealth of network request testing tools and methods. This article will introduce some techniques for using Golang for network request testing and give corresponding code examples.
1. Golang’s network request library
In Golang, there are many excellent network request libraries to choose from, the most commonly used of which is the net/http
package. net/http
Provides a simple and intuitive interface that can easily send and process HTTP requests. Below is a simple example showing how to use net/http
to send a GET request and print the response.
package main import ( "fmt" "net/http" "io/ioutil" ) func main() { resp, err := http.Get("https://example.com") if err != nil { fmt.Println("请求失败", err) return } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { fmt.Println("读取响应失败", err) return } fmt.Println(string(body)) }
In the above code, we use the http.Get
function to send a GET request and obtain the response result. Read the response Body content through the ioutil.ReadAll
function and output it in string form.
2. Send a POST request with parameters
Sometimes, we need to send a POST request with parameters, and Golang also provides the corresponding method. The following code example shows how to use the net/http
package to send a POST request with parameters and read the response.
package main import ( "fmt" "net/http" "io/ioutil" "strings" ) func main() { url := "https://example.com" payload := strings.NewReader("name=John&age=20") req, err := http.NewRequest("POST", url, payload) if err != nil { fmt.Println("创建请求失败", err) return } req.Header.Set("Content-Type", "application/x-www-form-urlencoded") client := &http.Client{} resp, err := client.Do(req) if err != nil { fmt.Println("请求失败", err) return } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { fmt.Println("读取响应失败", err) return } fmt.Println(string(body)) }
In the above code, we use the http.NewRequest
function to create a POST request and set the request header through req.Header
. Use the Do
method of http.Client
to send the request, and read the response content through ioutil.ReadAll
.
3. Simulate the server side
Sometimes, when testing network requests, we hope to simulate a server side to test the sending and processing of requests. In Golang, you can use the httptest
package to achieve this function. The following code example shows how to use the httptest
package to simulate a server side and handle GET requests.
package main import ( "fmt" "net/http" "net/http/httptest" ) func main() { handler := http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "Hello, World!") }) server := httptest.NewServer(handler) defer server.Close() resp, err := http.Get(server.URL) if err != nil { fmt.Println("请求失败", err) return } defer resp.Body.Close() fmt.Println("HTTP状态码:", resp.StatusCode) fmt.Println("响应内容:") fmt.Println(resp.Header) }
In the above code, we use http.HandlerFunc
to create an HTTP processing function, which will return "Hello, World!" to the client as the response content. Then use the httptest.NewServer
function to create a simulation server, and obtain the server's URL address through server.URL
. A GET request was sent using http.Get
, and the response status code and header information were printed.
Conclusion:
This article introduces some techniques for using Golang for network request testing and gives corresponding code examples. I hope readers can learn from this article how to use Golang to send and process network requests, thereby improving testing efficiency and quality.
The above is the detailed content of Tips for using Golang for network request testing. For more information, please follow other related articles on the PHP Chinese website!
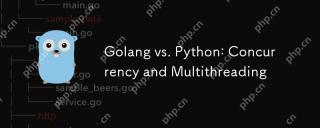
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
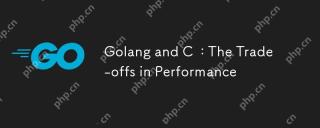
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
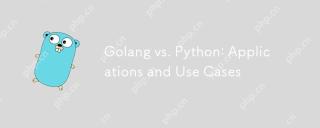
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
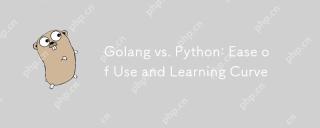
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
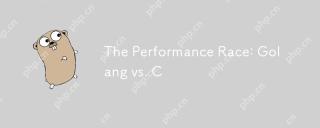
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
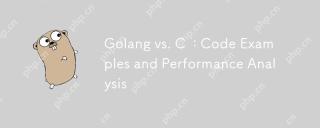
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
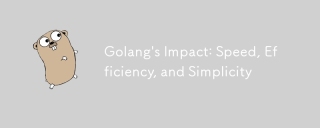
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
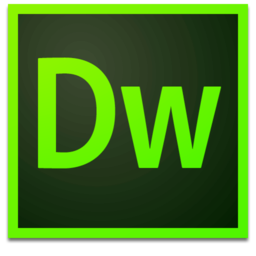
Dreamweaver Mac version
Visual web development tools
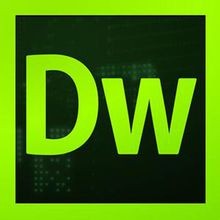
Dreamweaver CS6
Visual web development tools