


Use PHP and coreseek to implement fast chat history search function
In modern social networks and instant messaging applications, chat history is a very important information resource. However, when chat records exceed a certain amount, how to quickly and accurately search and find the content becomes a challenge. This article will introduce how to use PHP and coreseek to implement a fast chat history search function, and provide relevant code examples.
- Preparation
Before we begin, we need to install and configure coreseek, a Chinese full-text retrieval tool based on the open source search engine Sphinx. For specific installation steps, please refer to coreseek’s official documentation. - Data preparation
We assume that there is already a database that stores chat records, and there is a chat table containing the following fields: id, user_id, content and created_at. The id field is the unique identifier of the chat record, user_id represents the user ID of the sender, content represents the chat content, and created_at represents the chat time. - Create search index
In the coreseek configuration file, we need to specify the fields and data sources that need to be indexed. Create a configuration file named chat (for example: chat.conf) with the following content:
source chat { type = mysql sql_host = localhost sql_user = root sql_pass = sql_db = your_database sql_port = 3306 sql_query = SELECT id, content, created_at FROM chat sql_field_string = content sql_attr_timestamp = created_at } index chat_index { source = chat path = /path/to/index charset_type = utf-8 }
Among them, your_database needs to be replaced with your actual database name, and /path/to/index needs to be replaced with Your actual index storage path.
After saving the configuration file, run the following command to generate a search index:
$ indexer -c chat.conf
- Write PHP code
Next, we can implement the search function of chat records through PHP code. The following is a sample code for a simple search form and search result display:
<html> <head> <title>聊天记录搜索</title> </head> <body> <h1 id="聊天记录搜索">聊天记录搜索</h1> <form method="GET" action="search.php"> <input type="text" name="keyword" placeholder="请输入搜索关键词"> <input type="submit" value="搜索"> </form> <?php if(isset($_GET['keyword'])) { $keyword = $_GET['keyword']; // 连接到coreseek索引 $link = mysql_connect('localhost:9306'); mysql_select_db('chat_index', $link); // 执行搜索 $result = mysql_query("SELECT * FROM chat_index WHERE MATCH('$keyword') LIMIT 10", $link); if(mysql_num_rows($result) > 0) { // 显示搜索结果 echo '<h2 id="搜索结果">搜索结果</h2>'; while($row = mysql_fetch_assoc($result)) { echo '<p>内容:' . $row['content'] . '</p>'; echo '<p>时间:' . $row['created_at'] . '</p>'; echo '<hr>'; } } else { echo '未找到相关记录'; } // 关闭连接 mysql_close($link); } ?> </body> </html>
In the above code, we obtain the search keywords entered by the user through $_GET['keyword'] and connect to coreseek index. Then, execute a SQL query statement similar to SELECT * FROM chat_index WHERE MATCH('$keyword') LIMIT 10 to achieve keyword matching search.
Finally, output the content and time in the search results through a loop.
- Testing and Optimization
After completing the above steps, we can run the search.php file in the browser to test the search function. If the search results are inaccurate or display slowly, you can try to optimize coreseek or adjust the query statement.
To sum up, using PHP and coreseek can quickly realize the search function of chat records. Through reasonable configuration and optimization, we can improve search accuracy and response speed, and enhance user experience.
Note: The above code is only an example. In actual situations, it needs to be appropriately modified and improved according to specific needs.
The above is the detailed content of Use PHP and coreseek to implement fast chat history search function. For more information, please follow other related articles on the PHP Chinese website!
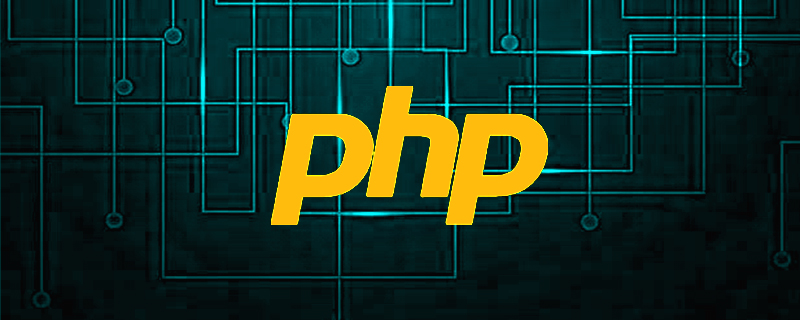
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
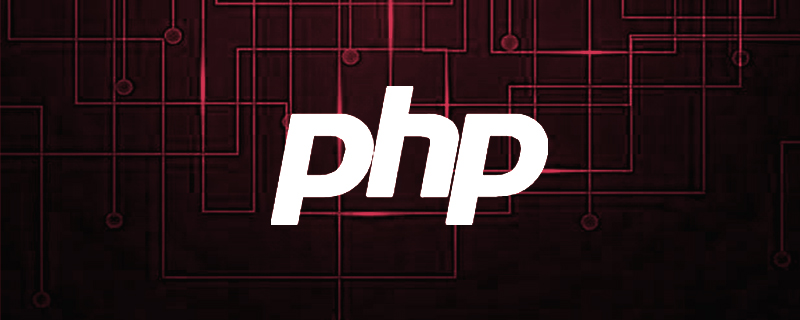
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
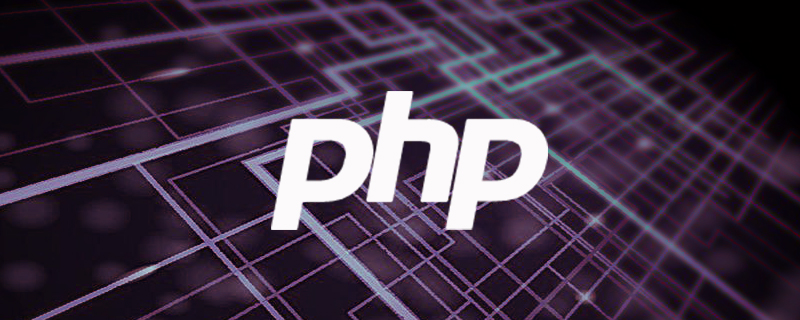
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
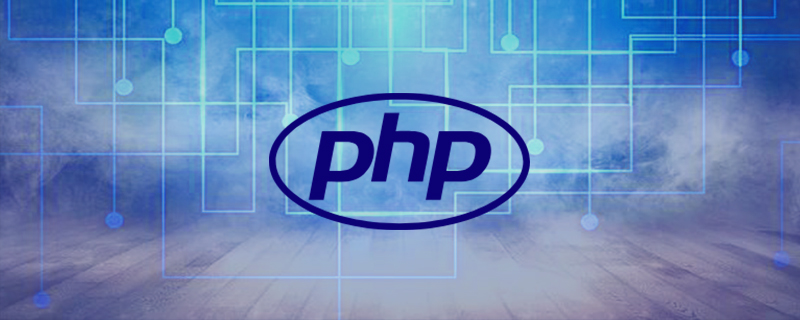
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
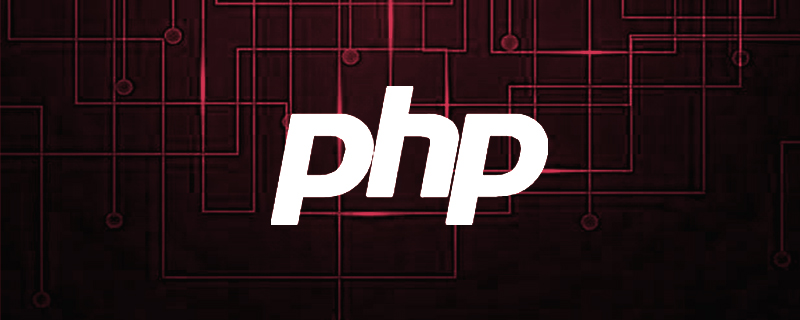
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
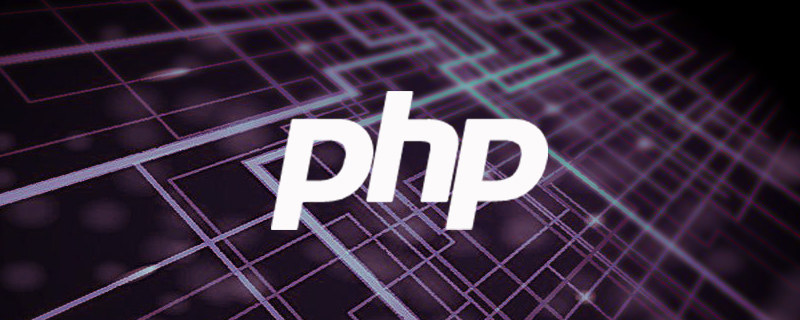
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
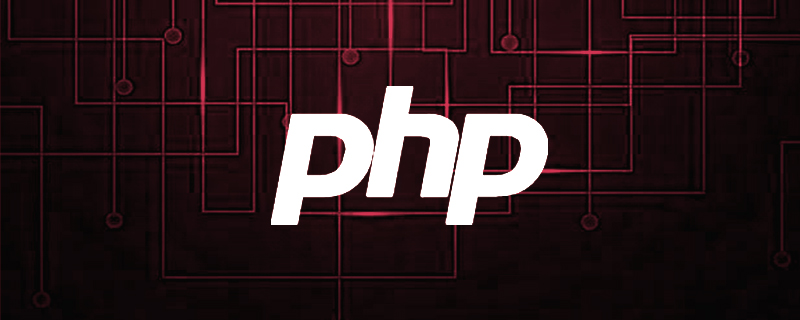
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
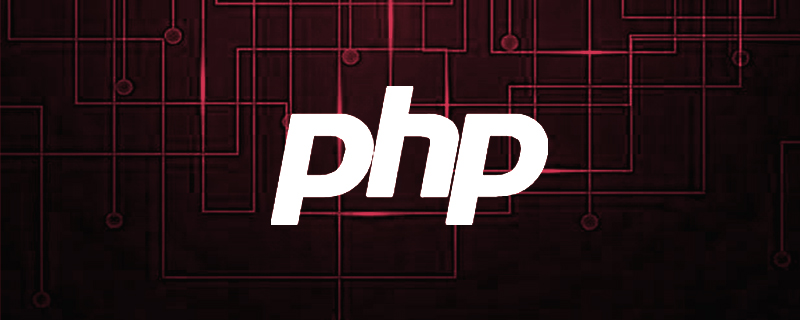
查找方法:1、用strpos(),语法“strpos("字符串值","查找子串")+1”;2、用stripos(),语法“strpos("字符串值","查找子串")+1”。因为字符串是从0开始计数的,因此两个函数获取的位置需要进行加1处理。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
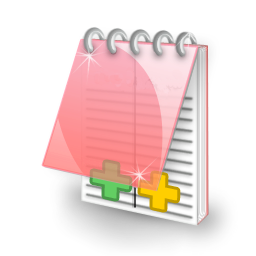
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
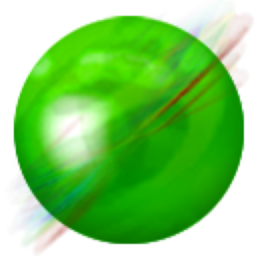
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
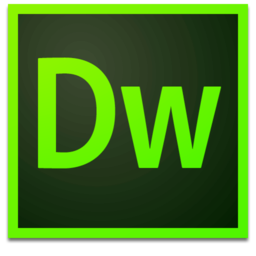
Dreamweaver Mac version
Visual web development tools
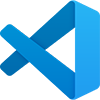
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
