Error handling in Golang: How to avoid panic?
Error handling in Golang: How to avoid panic?
In Golang, error handling is a very important task. Handling errors correctly not only improves the robustness of your program, it also makes your code more readable and maintainable. In error handling, a very common problem is the occurrence of panic. This article will introduce the concept of panic and discuss how to avoid panic and how to handle errors correctly.
What is panic?
In Golang, panic is an abnormal situation that causes the program to stop running immediately and output panic details. When the program encounters an error that cannot continue, such as array out of bounds, division by zero, etc., panic is usually triggered. For example:
func main() { fmt.Println("Start") panic("Something went wrong!") fmt.Println("End") }
The panic statement in the above code will cause the program to stop immediately and output "Something went wrong!", while the fmt.Println("End") code will never be executed.
Why should we avoid panic?
Although panic can help us find errors quickly, too many panics will lead to reduced program reliability. When there are too many panics in our program, it may have catastrophic consequences, such as program crash, data loss, etc. Therefore, we should try to avoid the occurrence of panic and solve the problem through error handling.
How to avoid panic?
The following are some common ways to avoid panic.
- Use if statements to check for possible error operations instead of using panic. For example:
if num > 0 { result := 10 / num fmt.Println(result) } else { fmt.Println("num must be greater than 0") }
By using an if statement to check the value of num, we can avoid a divide-by-zero panic.
- Use the panic/recover mechanism to handle exceptions. Panic/recover is an error handling mechanism provided in Golang. It allows us to actively trigger panic in the code and capture and handle panic through recover. For example:
func handleError() { if r := recover(); r != nil { fmt.Println("Recovered from panic:", r) } } func main() { defer handleError() panic("Something went wrong!") }
By using defer handleError() in the main function, we can call the handleError() function when a panic occurs and handle the panic there.
- Use error type instead of panic. When designing a function or method, we can use the error type in the return value to indicate errors that may occur. Determine whether an error has occurred by checking the error type, and handle it accordingly. For example:
func divide(a, b int) (int, error) { if b == 0 { return 0, errors.New("divide by zero") } return a / b, nil } func main() { result, err := divide(10, 0) if err != nil { fmt.Println("Error:", err) return } fmt.Println(result) }
In the above code, we use errors.New to create a new error object, representing the error of dividing by zero. In the main function, we first determine whether an error occurs by checking whether err is nil, and then handle it accordingly.
Handle errors correctly
In addition to avoiding panic, it is also very important to handle errors correctly. Here are some common ways to handle errors.
- Return the error object and pass it layer by layer. When an error occurs inside a function, the error can be passed to the upper layer by returning an error object. After the upper layer handles the error, you can choose to return the error to the higher layer, or perform other processing.
- Record error log. In addition to returning error objects, we can also record error logs when errors occur to facilitate subsequent troubleshooting. The log package in Golang provides a rich interface for logging.
- Use error codes. In some specific scenarios, we can use error codes to represent different error types. By checking error codes, errors can be judged and handled more accurately.
Summary
In Golang, avoiding the occurrence of panic is an important task. By using if statements, panic/recover mechanisms, and error types, we can effectively avoid panics and solve problems through error handling. At the same time, it is also very important to handle errors correctly to improve the reliability and robustness of the program. I hope this article will help you understand error handling in Golang, and I wish you can write more robust code!
The above is the detailed content of Error handling in Golang: How to avoid panic?. For more information, please follow other related articles on the PHP Chinese website!
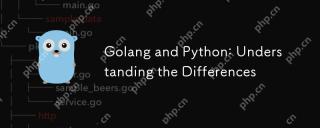
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
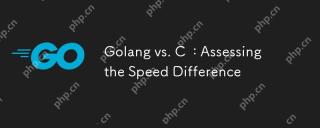
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
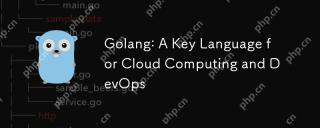
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
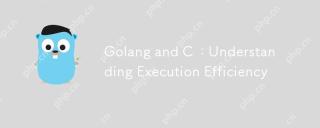
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
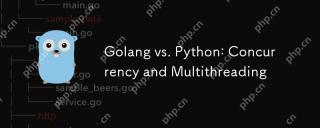
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
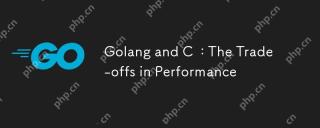
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
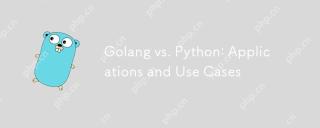
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
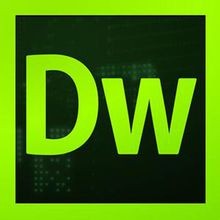
Dreamweaver CS6
Visual web development tools
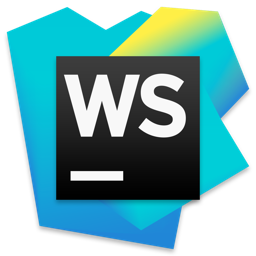
WebStorm Mac version
Useful JavaScript development tools
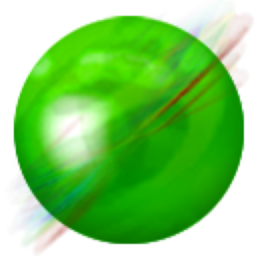
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor