The methods for deduplicating js arrays include using Set, using indexOf, using includes, using filter and using reduce. 1. Use Set, which is characterized by the fact that the elements in the set will not be repeated; 2. Use indexOf to return the first index position of the specified element in the array; 3. Use includes to determine whether an element already exists in the array. 4. Use filter to filter elements; 5. Use reduce to compress elements in an array, etc.
The operating environment of this tutorial: windows10 system, JavaScript ES12 version, DELL G3 computer.
In JavaScript programming, we often encounter situations where we need to perform deduplication operations on arrays. Deduplication refers to removing duplicate elements from an array and retaining only one of them. This article will introduce some common JavaScript array deduplication methods to help readers better understand and apply them.
Method 1: Use Set
The Set object introduced in ES6 is a new data structure that can help us quickly deduplicate arrays. The characteristic of the Set object is that the elements in the set will not be repeated. We can simply convert the array to a Set object, and then convert the Set object back to an array to achieve the purpose of deduplication.
Sample code:
let arr = [1, 2, 2, 3, 3, 4, 5]; let uniqueArr = Array.from(new Set(arr)); console.log(uniqueArr); // [1, 2, 3, 4, 5]
Method 2: Use indexOf
The array object in JavaScript provides an indexOf method, which can return the specified element in The first index position in the array. We can use this method to determine whether an element already exists in the new array, and if it does not exist, add it to it.
Sample code:
let arr = [1, 2, 2, 3, 3, 4, 5]; let uniqueArr = []; for (let i = 0; i < arr.length; i++) { if (uniqueArr.indexOf(arr[i]) === -1) { uniqueArr.push(arr[i]); } } console.log(uniqueArr); // [1, 2, 3, 4, 5]
Method 3: Use includes
The includes method introduced in ES7 is similar to the indexOf method. It can be used to determine an Whether the element already exists in the array. We can use this method to perform array deduplication operations.
Sample code:
let arr = [1, 2, 2, 3, 3, 4, 5]; let uniqueArr = []; for (let i = 0; i < arr.length; i++) { if (!uniqueArr.includes(arr[i])) { uniqueArr.push(arr[i]); } } console.log(uniqueArr); // [1, 2, 3, 4, 5]
Method 4: Use filter
The array object in JavaScript provides a filter method, which can be used to filter elements . We can use this method to perform array deduplication and only retain the first occurrence of the element.
Sample code:
let arr = [1, 2, 2, 3, 3, 4, 5]; let uniqueArr = arr.filter((item, index) => { return arr.indexOf(item) === index; }); console.log(uniqueArr); // [1, 2, 3, 4, 5]
Method 5: Use reduce
The array object in JavaScript provides a reduce method, which can Elements are "compressed" into a single value. We can use this method to perform array deduplication operations.
Sample code:
let arr = [1, 2, 2, 3, 3, 4, 5]; let uniqueArr = arr.reduce((prev, curr) => { if (!prev.includes(curr)) { prev.push(curr); } return prev; }, []); console.log(uniqueArr); // [1, 2, 3, 4, 5]
Conclusion:
This article introduces the commonly used array deduplication methods in JavaScript, including using Set, indexOf, and includes , filter and reduce methods. Readers can choose the appropriate method to perform array deduplication according to the actual situation. In actual development, array deduplication is a very basic and commonly used operation. Mastering these methods can process array data more efficiently and improve program performance and readability. .
The above is the detailed content of What are the methods to remove duplicates from js arrays?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
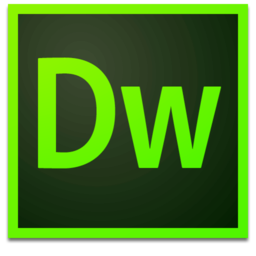
Dreamweaver Mac version
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
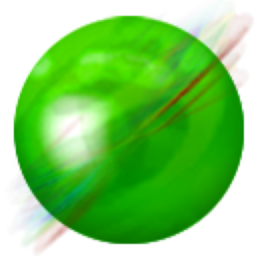
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!