


Utilize swoole development functions to achieve high-concurrency network communication
Using Swoole development functions to achieve high-concurrency network communication
Abstract: Swoole is a high-performance network communication framework based on PHP language, with coroutines, asynchronous IO, Features such as multi-processing are suitable for developing highly concurrent network applications. This article will introduce how to use Swoole to develop high-concurrency network communication functions and give some code examples.
- Introduction
With the rapid development of the Internet, the requirements for network communication are becoming higher and higher, especially in high-concurrency scenarios. Traditional PHP development faces the problem of weak concurrent processing capabilities, and Swoole provides us with an efficient and easy-to-use solution. - Asynchronous IO and coroutines
Swoole handles high-concurrency network communication through asynchronous IO and coroutines. Asynchronous IO allows the program to perform other tasks while waiting for IO to be completed, while coroutines can achieve efficient switching of multiple tasks. The combination of these two features gives Swoole the ability to handle high concurrency. -
Installation and configuration of Swoole
The installation of Swoole is very simple. You can install the Swoole extension by executing the following command in the terminal:pecl install swoole
After the installation is completed, in php. Add the following configuration to the ini file:
extension=swoole.so
-
Basic usage of Swoole
Next, we will use a simple example to illustrate the basic usage of Swoole. First, we need to create a Swoole server, the code is as follows:// 创建服务器对象 $server = new SwooleHTTPServer("127.0.0.1", 9501); // 设置回调函数 $server->on('request', function ($request, $response) { $response->header("Content-Type", "text/plain"); $response->end("Hello World "); }); // 启动服务器 $server->start();
The above code creates a server based on the HTTP protocol and sets a callback function. In the callback function, we can handle the client's request and return the response.
-
Concurrency processing
Swoole's asynchronous IO and coroutine features make concurrent processing simple. We can use coroutines to handle multiple requests at the same time. The code is as follows:use SwooleCoroutine; Coroutine::create(function () { $cli = new SwooleCoroutineHttpClient('www.baidu.com', 80); $cli->set(['timeout' => 10]); $cli->get('/'); echo $cli->body; }); Coroutine::create(function () { $cli = new SwooleCoroutineHttpClient('www.google.com', 80); $cli->set(['timeout' => 10]); $cli->get('/'); echo $cli->body; });
The above code creates two coroutines, sends HTTP requests to Baidu and Google respectively, and outputs the return results. Highly concurrent network communication can be easily achieved using coroutines.
-
Multi-process processing
In addition to coroutines, Swoole also provides multi-process processing functions. We can handle multiple requests at the same time through multiple processes. The code is as follows:$server = new SwooleServer("127.0.0.1", 9502); $server->set([ 'worker_num' => 4, ]); $server->on('receive', function ($server, $fd, $from_id, $data) { $pid = pcntl_fork(); if ($pid > 0) { // 主进程 $server->send($fd, 'Hello from main process'); } elseif ($pid == 0) { // 子进程 $server->send($fd, 'Hello from sub process'); exit(); } else { echo "fork failed"; } }); $server->start();
The above code creates a server with 4 worker processes, and each process can handle one request at the same time. Concurrent processing capabilities can be effectively improved through multiple processes.
- Summary
This article introduces how to use Swoole to develop high-concurrency network communication functions and gives some code examples. Through Swoole's asynchronous IO and coroutine features, we can easily achieve high concurrency processing capabilities. Using Swoole to develop high-concurrency network applications will improve the performance and stability of the system. Therefore, Swoole is an indispensable tool for PHP developers.
Reference materials:
[Swoole official document](https://www.swoole.com/)
[PHP process management-multi-process simulation concurrency](https:// www.swoole.com/)
The above is the detailed content of Utilize swoole development functions to achieve high-concurrency network communication. For more information, please follow other related articles on the PHP Chinese website!
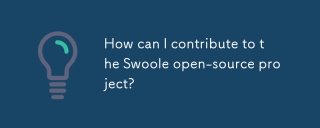
The article outlines ways to contribute to the Swoole project, including reporting bugs, submitting features, coding, and improving documentation. It discusses required skills and steps for beginners to start contributing, and how to find pressing is
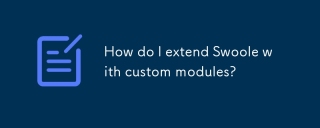
Article discusses extending Swoole with custom modules, detailing steps, best practices, and troubleshooting. Main focus is enhancing functionality and integration.
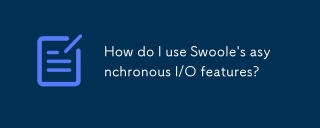
The article discusses using Swoole's asynchronous I/O features in PHP for high-performance applications. It covers installation, server setup, and optimization strategies.Word count: 159
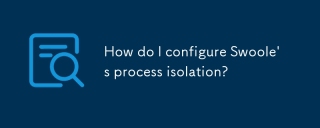
Article discusses configuring Swoole's process isolation, its benefits like improved stability and security, and troubleshooting methods.Character count: 159
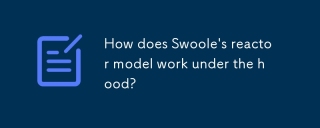
Swoole's reactor model uses an event-driven, non-blocking I/O architecture to efficiently manage high-concurrency scenarios, optimizing performance through various techniques.(159 characters)
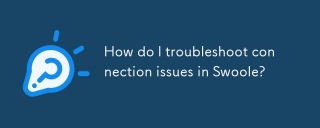
Article discusses troubleshooting, causes, monitoring, and prevention of connection issues in Swoole, a PHP framework.
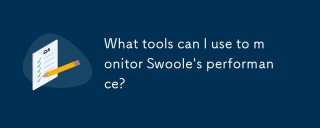
The article discusses tools and best practices for monitoring and optimizing Swoole's performance, and troubleshooting methods for performance issues.
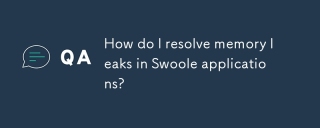
Abstract: The article discusses resolving memory leaks in Swoole applications through identification, isolation, and fixing, emphasizing common causes like improper resource management and unmanaged coroutines. Tools like Swoole Tracker and Valgrind


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
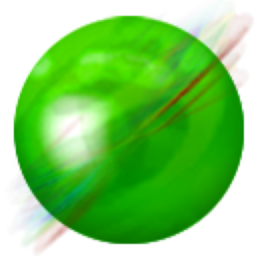
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
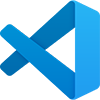
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.