


Code generation for supplier credit evaluation function in PHP inventory management system
In the inventory management system, supplier credit evaluation is one of the most important functions one. Credit evaluation of suppliers can help companies select suppliers with integrity and stable supply capabilities, thereby improving procurement efficiency and reducing procurement risks. This article will introduce how to use PHP code to implement the supplier credit evaluation function and give corresponding code examples.
- Database design
First, we need to design the corresponding database table to store supplier information and credit evaluation data. Suppose we have two tables: supplier and credit_evaluation. The supplier table is used to store basic information about suppliers, and the credit_evaluation table is used to store credit evaluation related data.
CREATE TABLE supplier ( id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(255) NOT NULL, contact_person VARCHAR(255) NOT NULL, contact_number VARCHAR(255) NOT NULL ); CREATE TABLE credit_evaluation ( id INT PRIMARY KEY AUTO_INCREMENT, supplier_id INT NOT NULL, evaluation_date DATE NOT NULL, evaluation_score INT NOT NULL, FOREIGN KEY (supplier_id) REFERENCES supplier(id) );
- Supplier credit evaluation function code example
Let’s implement the code for the supplier credit evaluation function below. First, we need to establish a database connection.
<?php // 数据库连接配置 $servername = "localhost"; $username = "root"; $password = "secret"; $dbname = "inventory_management"; // 创建数据库连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检查连接是否成功 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } ?>
Next, we can implement the input function of supplier information.
<?php // 供应商信息录入 if($_SERVER["REQUEST_METHOD"] == "POST") { $name = $_POST['name']; $contact_person = $_POST['contact_person']; $contact_number = $_POST['contact_number']; $sql = "INSERT INTO supplier (name, contact_person, contact_number) VALUES ('$name', '$contact_person', '$contact_number')"; if ($conn->query($sql) === TRUE) { echo "供应商信息录入成功!"; } else { echo "供应商信息录入失败:" . $conn->error; } } ?>
Then, we can implement the supplier credit evaluation function.
<?php // 供应商信用评估 if($_SERVER["REQUEST_METHOD"] == "POST") { $supplier_id = $_POST['supplier_id']; $evaluation_date = $_POST['evaluation_date']; $evaluation_score = $_POST['evaluation_score']; $sql = "INSERT INTO credit_evaluation (supplier_id, evaluation_date, evaluation_score) VALUES ('$supplier_id', '$evaluation_date', '$evaluation_score')"; if ($conn->query($sql) === TRUE) { echo "信用评估成功!"; } else { echo "信用评估失败:" . $conn->error; } } ?>
Finally, we can also implement the function of querying the credit evaluation data of specific suppliers.
<?php // 查询供应商信用评估数据 $sql = "SELECT supplier.name, credit_evaluation.evaluation_date, credit_evaluation.evaluation_score FROM supplier INNER JOIN credit_evaluation ON supplier.id = credit_evaluation.supplier_id WHERE supplier.id = 1"; $result = $conn->query($sql); if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { echo "供应商姓名:" . $row["name"]. " - 评估日期:" . $row["evaluation_date"]. " - 评估分数:" . $row["evaluation_score"]. "<br>"; } } else { echo "暂无评估数据"; } ?>
Through the above code example, we can implement the supplier credit evaluation function. Users can enter supplier information and evaluation data, and the system will perform credit evaluation based on the evaluation data and support querying the evaluation data of specific suppliers.
Summary:
By generating code for the supplier credit evaluation function in the PHP inventory management system, we can learn how to design database tables and use PHP to implement supplier information entry, credit evaluation and data query and other functions. These functions help improve the procurement efficiency of the inventory management system and reduce procurement risks.
The above is the detailed content of Code generation for supplier credit evaluation function in PHP inventory management system. For more information, please follow other related articles on the PHP Chinese website!
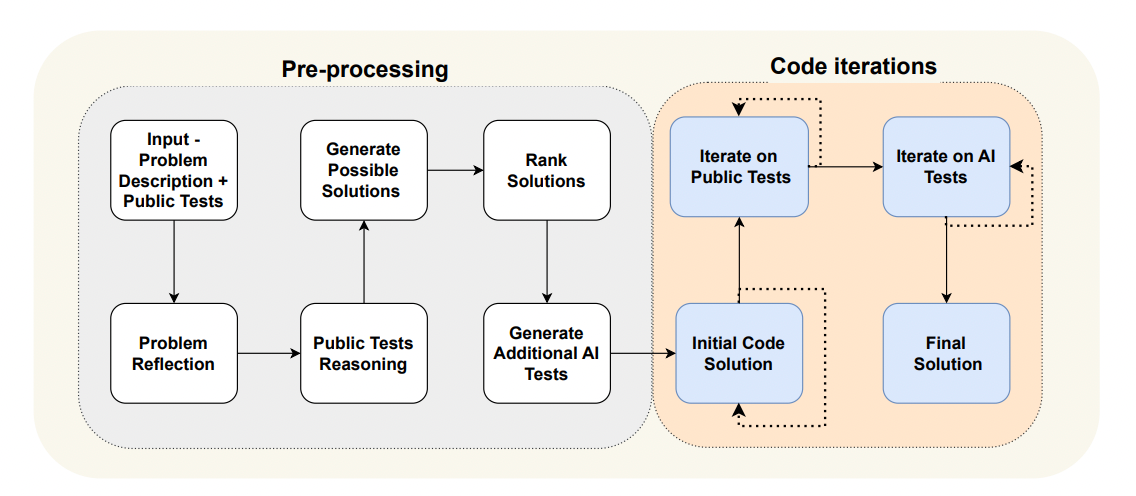
一篇新论文的作者提出了一种“强化”代码生成的方法。代码生成是人工智能中一项日益重要的能力。它通过训练机器学习模型,根据自然语言描述自动生成计算机代码。这一技术具有广泛的应用前景,可以将软件规格转化为可用的代码,自动化后端开发,并协助人类程序员提高工作效率。然而,生成高质量代码对AI系统仍然具有挑战性,与翻译或总结等语言任务相比。代码必须准确地符合目标编程语言的语法,能够优雅地处理各种极端情况和意外输入,并精确地处理问题描述中的许多小细节。即使是其他领域看似无害的小错误也可能完全破坏程序的功能,导
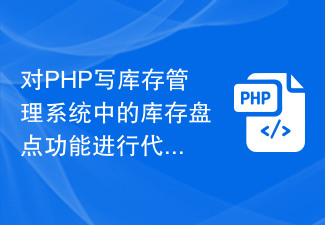
对PHP写库存管理系统中的库存盘点功能进行代码生成在现代企业中,库存是一个非常重要的资源。准确管理库存对于企业的顺利运营非常关键。为了更好地管理库存,许多企业使用库存管理系统来跟踪库存的变化,并实时更新库存记录。其中,库存盘点功能是库存管理系统中的一个重要组成部分。本文将为您介绍如何使用PHP编写库存管理系统中的库存盘点功能,并提供代码示例。首先,我们需要明
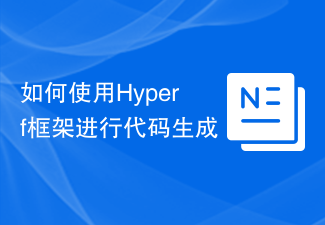
如何使用Hyperf框架进行代码生成一、介绍Hyperf框架是基于Swoole2.0+的高性能微服务框架。它内置了基于Hyperf框架的代码生成器,可以帮助我们快速生成常见的代码文件,提高开发效率。本文将介绍如何使用Hyperf框架的代码生成功能,包括控制器、模型和验证器的生成。二、安装与配置安装Hyperf框架首先,我们需要通过Composer来安装Hyp
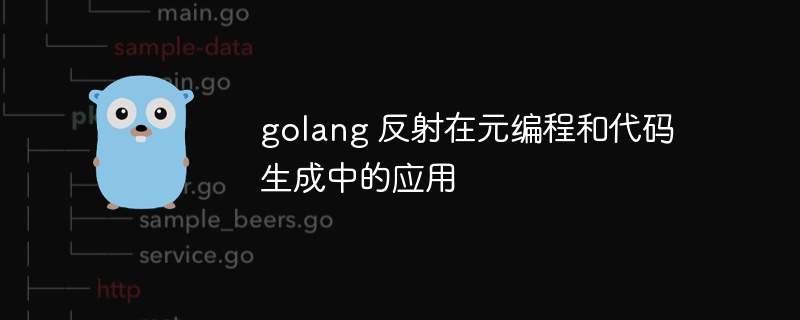
反射在Go语言中的元编程和代码生成中十分有用:元编程:允许程序在运行时创建新类型、函数和变量,修改现有类型结构。代码生成:可以动态生成代码片段,并在运行时执行它们,例如生成实现特定接口的函数。
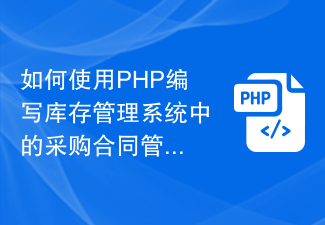
如何使用PHP编写库存管理系统中的采购合同管理功能代码随着电子商务的蓬勃发展,库存管理对于企业来说变得越发重要。在库存管理中,采购合同管理功能对于企业来说尤为关键。本文将介绍如何使用PHP编写库存管理系统中的采购合同管理功能代码。采购合同管理是库存管理系统中的一个重要模块,它负责管理供应商与企业之间的采购合同。这个模块需要实现以下功能:创建采购合同、查看采购
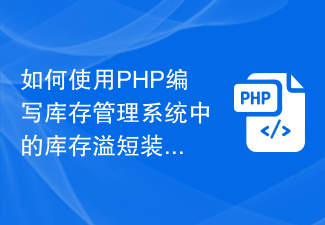
如何使用PHP编写库存管理系统中的库存溢短装功能代码引言:库存管理是每个企业都要面对的重要任务。一个优秀的库存管理系统能够帮助企业提高库存运营效率、降低成本以及减少库存短缺和溢出的风险。本文将介绍如何使用PHP编写库存管理系统中的库存溢短装功能代码,以实现库存数量的监控和控制,帮助企业实现高效的库存管理。一、库存溢短装功能的实现思路在编写库存溢短装功能代码之
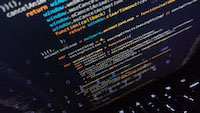
python元编程基础Python元编程是动态地操作Python代码的能力,这使得Python成为一门非常强大的语言。元编程可以通过以下几种方式实现:类装饰器:类装饰器是一种修改类定义的装饰器。它可以用来添加或修改类的属性和方法,也可以用来控制类的实例化过程。defadd_method_to_class(cls):defnew_method(self):print("Thisisanewmethod")setattr(cls,"new_method",new_method)returncls@a
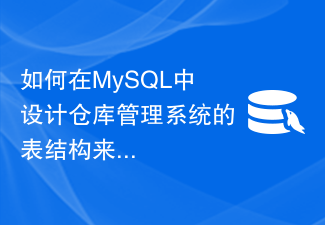
如何在MySQL中设计仓库管理系统的表结构来管理库存入库和出库?随着电子商务的迅猛发展,仓库管理系统成为了许多企业不可或缺的一部分。在仓库管理系统中,库存的入库和出库是两个非常重要的环节。因此,设计一个合适的表结构来管理库存的入库和出库就显得至关重要了。本篇文章将详细介绍如何在MySQL中设计仓库管理系统的表结构来管理库存的入库和出库,并提供相应的代码示例。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
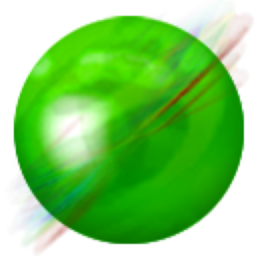
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
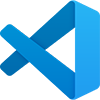
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
