Microservice data encryption and decryption functions written in Java
Microservice data encryption and decryption function written in Java
In today's Internet era, with the popularity of data transmission and the increasing risk of leakage of sensitive information, data security is becoming increasingly important. The rise of microservices architecture provides more flexible and scalable solutions for data encryption and decryption. This article will introduce how to use Java to write a simple but powerful microservice data encryption and decryption function, and give corresponding code examples.
First, we need to choose a reliable encryption algorithm. Here, we choose to use the AES (Advanced Encryption Standard) algorithm, which is a very popular and safe and reliable symmetric encryption algorithm. The AES algorithm supports 128-bit, 192-bit and 256-bit key lengths. We can choose different key lengths according to actual needs. In addition, we also need to choose an appropriate padding mode and encryption mode. Here, we choose to use PKCS5Padding padding mode and CBC encryption mode.
Next, we use Java’s cryptography library to perform encryption and decryption operations. First, we need to generate a key and keep it in a safe place. Here we use the KeyGenerator class provided by Java to generate keys.
import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; public class EncryptionUtils { public static SecretKey generateKey() throws NoSuchAlgorithmException { KeyGenerator keyGenerator = KeyGenerator.getInstance("AES"); keyGenerator.init(128); return keyGenerator.generateKey(); } }
After generating the key, we can use the key to perform encryption and decryption operations. Below is sample code that uses a key to encrypt data.
import javax.crypto.Cipher; import javax.crypto.SecretKey; import javax.crypto.spec.IvParameterSpec; import javax.crypto.spec.SecretKeySpec; import java.nio.charset.StandardCharsets; import java.security.GeneralSecurityException; import java.util.Base64; public class EncryptionUtils { public static String encryptData(String data, SecretKey secretKey, String initVector) throws GeneralSecurityException { Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding"); cipher.init(Cipher.ENCRYPT_MODE, secretKey, new IvParameterSpec(initVector.getBytes(StandardCharsets.UTF_8))); byte[] encryptedData = cipher.doFinal(data.getBytes(StandardCharsets.UTF_8)); return Base64.getEncoder().encodeToString(encryptedData); } }
In the above code, we use Base64 encoding to convert the encrypted data to facilitate transmission and storage on the network. Next, we give example code for decrypting data using a key.
import javax.crypto.Cipher; import javax.crypto.SecretKey; import javax.crypto.spec.IvParameterSpec; import javax.crypto.spec.SecretKeySpec; import java.nio.charset.StandardCharsets; import java.security.GeneralSecurityException; import java.util.Base64; public class EncryptionUtils { public static String decryptData(String encryptedData, SecretKey secretKey, String initVector) throws GeneralSecurityException { Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding"); cipher.init(Cipher.DECRYPT_MODE, secretKey, new IvParameterSpec(initVector.getBytes(StandardCharsets.UTF_8))); byte[] decryptedData = cipher.doFinal(Base64.getDecoder().decode(encryptedData)); return new String(decryptedData, StandardCharsets.UTF_8); } }
In the above code, we use Base64 decoding to restore the encrypted data.
Finally, we can encapsulate the above encryption and decryption functions into a microservice API to facilitate other system calls. Below is a simple microservice example code.
import org.springframework.web.bind.annotation.*; import javax.crypto.SecretKey; import java.security.NoSuchAlgorithmException; import java.util.HashMap; import java.util.Map; @RestController @RequestMapping("/encryption") public class EncryptionController { private SecretKey secretKey; public EncryptionController() throws NoSuchAlgorithmException { this.secretKey = EncryptionUtils.generateKey(); } @PostMapping("/encrypt") public Map<String, String> encryptData(@RequestBody Map<String, String> data) throws GeneralSecurityException { String encryptedData = EncryptionUtils.encryptData(data.get("data"), secretKey, data.get("initVector")); Map<String, String> result = new HashMap<>(); result.put("encryptedData", encryptedData); return result; } @PostMapping("/decrypt") public Map<String, String> decryptData(@RequestBody Map<String, String> data) throws GeneralSecurityException { String decryptedData = EncryptionUtils.decryptData(data.get("encryptedData"), secretKey, data.get("initVector")); Map<String, String> result = new HashMap<>(); result.put("decryptedData", decryptedData); return result; } }
In the above code, we use the Spring framework to implement a simple HTTP interface, where the /encryption/encrypt
interface is used to encrypt data, /encryption/decrypt
Interface is used to decrypt data. The request parameters use JSON format, and the encrypted or decrypted results are returned.
To sum up, we use Java to write a simple but powerful microservice data encryption and decryption function. Use the AES algorithm to encrypt and decrypt data, and encapsulate it into a microservice API that can be called by other systems. This can ensure the security of data during transmission and storage and improve the overall security of the system.
The above is the detailed content of Microservice data encryption and decryption functions written in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
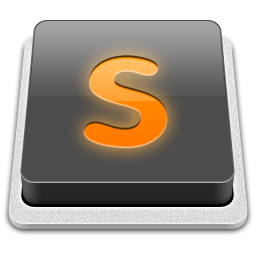
SublimeText3 Mac version
God-level code editing software (SublimeText3)