


Performance testing and tuning method of PHP email docking class
- Introduction
With the development of the Internet, email has become a way for people to communicate daily. One of the important ways. When developing a website or application, you often need to use PHP to send and receive emails. In order to improve the efficiency of sending and receiving emails, we can perform performance testing and tuning on the PHP email docking class. This article explains how to conduct these tests and provides some code examples. - Performance Test
Performance testing can help us understand the performance bottlenecks of the email docking class and find out the direction for optimization. The following are some common performance testing methods:
2.1 Stress Test
Stress testing is used to simulate high-concurrency scenarios of sending and receiving emails to test the performance of email docking classes under high load conditions. Performance. You can use tools such as ApacheBench or JMeter to perform stress testing. The following is an example of using ApacheBench to conduct a simple stress test:
// 假设使用PHPMailer作为邮件对接类 require 'vendor/phpmailer/phpmailer/src/PHPMailer.php'; //...初始化邮件对象 // 发送邮件 function sendEmail($mailer, $to, $subject, $body) { $mailer->addAddress($to); $mailer->Subject = $subject; $mailer->Body = $body; return $mailer->send(); } // 压力测试函数 function stressTest($mailer, $to, $subject, $body, $totalRequests) { $successCount = 0; $failureCount = 0; for ($i = 0; $i < $totalRequests; $i++) { echo "Sending email: ", $i+1, " of ", $totalRequests, " "; if (sendEmail($mailer, $to, $subject, $body)) { $successCount++; } else { $failureCount++; } // 休眠一段时间,模拟真实场景下的请求间隔 usleep(mt_rand(1000, 5000)); } echo "Successful requests: ", $successCount, " "; echo "Failed requests: ", $failureCount, " "; } // 压力测试参数 $totalRequests = 100; // 总请求数 $to = "recipient@example.com"; // 收件人邮箱 $subject = "Test Email"; // 邮件主题 $body = "This is a test email."; // 邮件内容 // 创建邮件对象 $mailer = new PHPMailerPHPMailerPHPMailer(); // 进行压力测试 stressTest($mailer, $to, $subject, $body, $totalRequests);
2.2 Concurrency test
Concurrency test is used to test the performance of the email docking class when processing multiple requests at the same time. Multiple threads or processes can be used to simulate concurrent requests. The following is an example of using multi-threading for simple concurrency testing:
// 假设使用SwiftMailer作为邮件对接类 require 'vendor/swiftmailer/swiftmailer/lib/swift_required.php'; //...初始化邮件对象 // 并发发送邮件 function sendEmail($mailer, $to, $subject, $body) { $mailer->setTo($to); $mailer->setSubject($subject); $mailer->setBody($body); return $mailer->send(); } // 并发测试函数 function concurrentTest($mailer, $to, $subject, $body, $concurrency) { $totalRequests = $concurrency; $doneCount = 0; $successCount = 0; $failureCount = 0; // 创建并发线程 $threads = []; for ($i = 0; $i < $concurrency; $i++) { $threads[$i] = new Thread('sendEmail', $mailer, $to, $subject, $body); $threads[$i]->start(); } // 等待所有线程完成 foreach ($threads as $thread) { $thread->join(); $doneCount++; if ($thread->getReturn()) { $successCount++; } else { $failureCount++; } } echo "Total requests: ", $totalRequests, " "; echo "Successful requests: ", $successCount, " "; echo "Failed requests: ", $failureCount, " "; } // 并发测试参数 $concurrency = 10; // 并发数 $to = "recipient@example.com"; // 收件人邮箱 $subject = "Test Email"; // 邮件主题 $body = "This is a test email."; // 邮件内容 // 创建邮件对象 $mailer = Swift_Mailer::newInstance(Swift_SmtpTransport::newInstance('localhost', 25)); // 进行并发测试 concurrentTest($mailer, $to, $subject, $body, $concurrency);
- Performance Tuning
Performance testing can help us find performance bottlenecks in the email docking class and then optimize them. The following are some common performance tuning methods:
3.1 Mail Queue
Put the emails to be sent into the queue, and use multi-process or scheduled tasks to process the emails in the queue. This can separate email sending from the logic of the application, improving the concurrency and response speed of email sending.
// 将邮件放入队列 function enqueueEmail($to, $subject, $body) { // 添加到邮件队列,保存到数据库或文件中 } // 从队列中发送邮件 function processEmailQueue() { // 从数据库或文件中读取待发送的邮件 // 使用邮件对接类发送邮件 // 更新队列状态,标记邮件为已发送 } // 将邮件加入队列 enqueueEmail("recipient@example.com", "Test Email", "This is a test email."); // 每隔一段时间处理邮件队列 processEmailQueue();
3.2 Send emails in batches
Merging multiple emails into one email for sending can reduce the number of connections to the mail server and improve performance. The following is an example of sending emails in batches using SMTP:
// 假设使用Guzzle作为HTTP客户端 require 'vendor/guzzlehttp/guzzle/src/Client.php'; //...初始化Guzzle客户端 // 使用Guzzle发送HTTP请求 function sendHttpRequest($client, $method, $url, $headers, $body = "") { $request = $client->createRequest($method, $url, $headers, $body); $response = $client->send($request); return $response; } // 批量发送邮件 function sendBatchEmail($client, $to, $subject, $body) { // 将多个邮件合并为一个邮件 $emails = implode(", ", $to); $subject = "Multiple Emails: " . $subject; $body = "Multiple Email Bodies: " . implode(" ", $body); // 发送邮件 $url = "https://smtp.example.com/send"; $headers = [ "Content-Type" => "application/json", // 添加其他必要的请求头信息 ]; $data = [ "to" => $emails, "subject" => $subject, "body" => $body ]; $response = sendHttpRequest($client, "POST", $url, $headers, json_encode($data)); return $response->getStatusCode() == 200; } // 使用Guzzle发送HTTP请求的参数 $client = new GuzzleHttpClient(); $baseUrl = "https://api.example.com"; // API基础地址 $headers = [ // 添加请求头信息 ]; // 批量发送邮件参数 $to = ["recipient1@example.com", "recipient2@example.com"]; // 收件人邮箱 $subject = "Test Email"; // 邮件主题 $body = ["This is the first email.", "This is the second email."]; // 邮件内容 // 进行批量发送 $response = sendBatchEmail($client, $to, $subject, $body);
- Summary
This article introduces how to perform performance testing and tuning of the PHP email docking class, and provides some code examples. Performance testing can help us understand the performance bottlenecks of email docking classes, and performance tuning can improve the efficiency of sending and receiving emails. Through reasonable performance testing and tuning, the performance and user experience of the application in email processing can be improved. Hope this article is helpful to everyone.
The above is the detailed content of Performance testing and tuning methods for PHP email docking class. For more information, please follow other related articles on the PHP Chinese website!
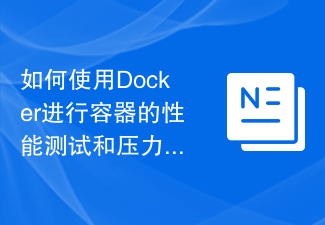
如何使用Docker进行容器的性能测试和压力测试,需要具体代码示例引言容器虚拟化技术的兴起使得应用程序的部署和运行更加灵活和高效,其中最受欢迎的工具之一就是Docker。作为一种轻量级的容器化平台,Docker提供了一种方便的方式来打包、分发和运行应用程序,但是如何对容器的性能进行测试和评估,特别是在高负载情况下的压力测试,是很多人关心的问题。本文将介绍
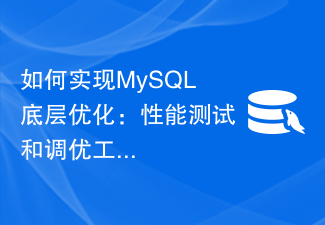
如何实现MySQL底层优化:性能测试和调优工具的高级使用与分析引言MySQL是一种常用的关系型数据库管理系统,广泛应用于各种Web应用和大型软件系统中。为了确保系统的运行效率和性能,我们需要进行MySQL的底层优化。本文将介绍如何使用性能测试和调优工具进行高级使用和分析,并提供具体的代码示例。一、性能测试工具的选择和使用性能测试工具是评估系统性能和瓶颈的重要
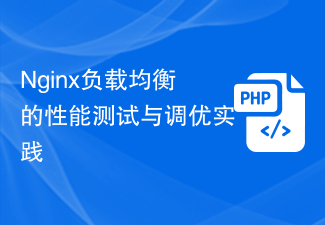
Nginx负载均衡的性能测试与调优实践概述:Nginx作为一款高性能的反向代理服务器,常用于负载均衡的应用场景。本文将介绍如何进行Nginx负载均衡的性能测试,并通过调优实践提升其性能。性能测试准备:在进行性能测试之前,我们需要准备一台或多台具备较好性能的服务器,安装Nginx,并配置反向代理与负载均衡。测试工具选择:为了模拟真实的负载情况,我们可以使用常见
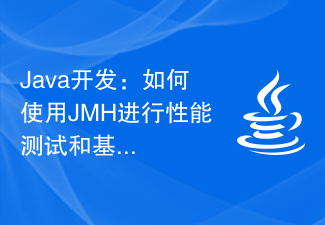
Java开发:如何使用JMH进行性能测试和基准测试引言:在Java开发过程中,我们经常需要测试代码的性能和效率。为了准确地评估代码的性能,我们可以使用JMH(JavaMicrobenchmarkHarness)工具,它是专门为Java开发者设计的一款性能测试和基准测试的工具。本文将介绍如何使用JMH进行性能测试和基准测试,并提供一些具体的代码示例。一、什
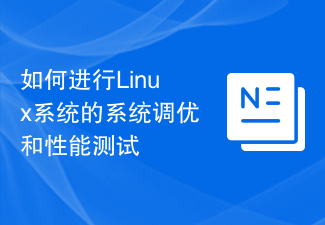
操作系统的性能优化是保证系统高效运行的关键之一。在Linux系统中,我们可以通过各种方法进行性能调优和测试,以确保系统的最佳性能表现。本文将介绍如何进行Linux系统的系统调优和性能测试,并提供相应的具体代码示例。一、系统调优系统调优是通过调整系统的各项参数,来优化系统的性能。以下是一些常见的系统调优方法:1.修改内核参数Linux系统的内核参数控制着系统运
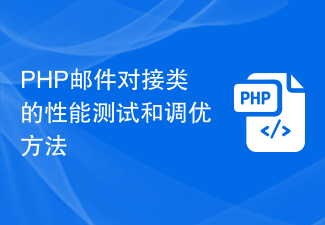
PHP邮件对接类的性能测试和调优方法引言随着互联网的发展,电子邮件已成为人们日常沟通的重要方式之一。在开发网站或应用程序时,经常需要使用PHP来发送和接收电子邮件。为了提高邮件发送和接收的效率,我们可以对PHP邮件对接类进行性能测试和调优。本文将介绍如何进行这些测试,并提供一些代码示例。性能测试性能测试可以帮助我们了解邮件对接类的性能瓶颈,并找出优化的方向。
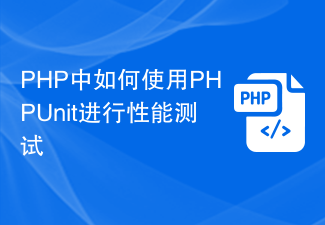
PHPUnit是PHP中非常流行的单元测试框架,它也可以用作性能测试。本文将介绍如何使用PHPUnit进行性能测试。首先,需要了解PHPUnit的一些基本概念。PHPUnit中的测试用例(TestCase)被定义为一个类,该类继承了PHPUnitFrameworkTestCase类。测试用例类中有一个或多个测试方法(testmethods),每个测试方法使
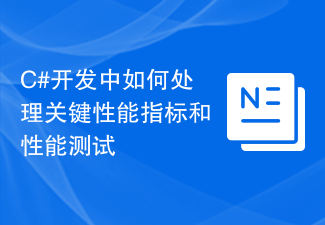
C#开发中如何处理关键性能指标和性能测试,需要具体代码示例在C#开发中,性能是一个非常重要的考虑因素。当我们开发一个项目时,无论是桌面应用程序、Web应用程序还是移动应用程序,我们都希望它能够运行得足够快,并且在使用过程中不会出现卡顿或延迟的情况。因此,我们需要关注和处理关键性能指标,并进行性能测试来确保应用的高性能和稳定性。处理关键性能指标处理关键性能指标


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
