Error handling in Golang: Use the Wrap function to add error context
Introduction:
In the software development process, error handling is a very important link. A good error handling mechanism can help us quickly diagnose and deal with various problems and improve the reliability and maintainability of software. As a modern programming language, Golang provides a simple and powerful error handling mechanism. The Wrap function is a very useful tool that can help us add error context and trace the source of the problem in the error stack. This article will introduce error handling and the use of Wrap function in Golang in detail, and provide some practical code examples.
Basics of error handling:
In Golang, error handling is implemented by returning a value of type error. The error type in Golang is an interface, defined as follows:
type error interface { Error() string }
We can customize and implement our own error types according to business needs. When a function returns a value of type error, we usually use an if statement to determine whether it is nil to determine whether an error has occurred. The following is a simple example:
func Divide(a, b int) (int, error) { if b == 0 { return 0, errors.New("divisor cannot be zero") } return a / b, nil } func main() { result, err := Divide(10, 0) if err != nil { fmt.Println("error:", err) return } fmt.Println("result:", result) }
In the above example, we define a Divide function to divide two numbers. When the divisor is 0, we return an error value created using the errors.New function. In the main function, we determine whether an error occurs by determining whether err is nil, and print the error message.
Use the Wrap function to add error context:
Although we can help the caller diagnose the problem by returning a meaningful error message, in more complex scenarios we may need more contextual information. For example, when an error occurs when calling function A, we want to know which part of the logic of function A caused the error. At this time, we can use the Wrap function to add error context.
Golang's errors package provides a function called Wrap, which is defined as follows:
func Wrap(err error, message string) error
The Wrap function receives an error type value and a string, and it will return a new An error value containing the original error and additional contextual information. Here is an example:
func FuncA() error { // do something return errors.New("error in FuncA") } func FuncB() error { err := FuncA() if err != nil { return errors.Wrap(err, "error in FuncB") } // do something return nil } func main() { err := FuncB() if err != nil { fmt.Println("error:", err) return } }
In the above example, we defined two functions FuncA and FuncB. FuncA represents a problematic function, which returns a simple error value. In FuncB, we call FuncA and use the Wrap function to add contextual information to the error. In the main function, we print the error with contextual information to better diagnose the problem.
By using the Wrap function, we can add multiple levels of contextual information to errors. Here is a more complex example:
func FuncA() error { return errors.Errorf("error in FuncA: %w", errors.New("something went wrong")) } func FuncB() error { err := FuncA() if err != nil { return errors.Wrap(err, "error in FuncB") } return nil } func FuncC() error { err := FuncB() if err != nil { return errors.Wrap(err, "error in FuncC") } return nil } func main() { err := FuncC() if err != nil { fmt.Println("error:", err) return } }
In the above example, we used the errors.Errorf function in the FuncA function, which allows us to add formatted contextual information to errors. By using the %w placeholder we can include the original error, thus forming an error chain. In the main function, we print the error with multiple levels of context information.
Summary:
In this article, we introduced the error handling mechanism and the use of the Wrap function in Golang. Using the Wrap function can help us add contextual information to errors to better diagnose problems. By using the Wrap function properly, we can build a clear error stack and trace the source of the problem. I hope this article will help you understand and apply Golang's error handling.
The above is the detailed content of Error handling in Golang: Add error context using Wrap function. For more information, please follow other related articles on the PHP Chinese website!
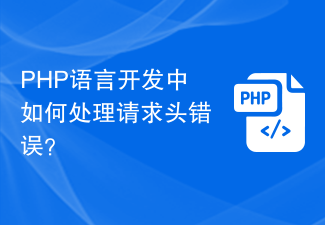
在PHP语言开发中,请求头错误通常是由于HTTP请求中的一些问题导致的。这些问题可能包括无效的请求头、缺失的请求体以及无法识别的编码格式等。而正确处理这些请求头错误是保证应用程序稳定性和安全性的关键。在本文中,我们将讨论一些处理PHP请求头错误的最佳实践,帮助您构建更加可靠和安全的应用程序。检查请求方法HTTP协议规定了一组可用的请求方法(例如GET、POS
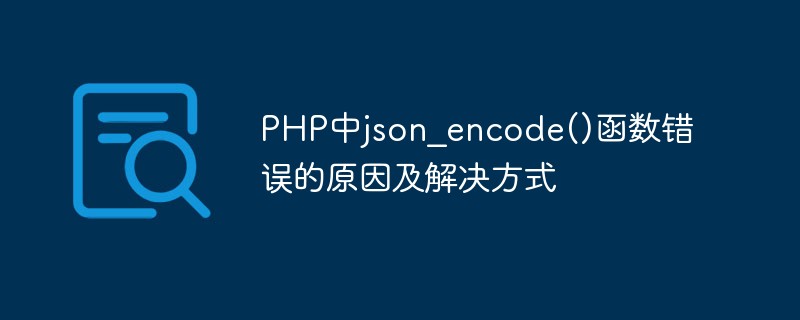
随着Web应用程序的不断发展,数据交互成为了一个非常重要的环节。其中,JSON(JavaScriptObjectNotation)是一种轻量级的数据交换格式,广泛用于前后端数据交互。在PHP中,json_encode()函数可以将PHP数组或对象转换为JSON格式字符串,json_decode()函数可以将JSON格式字符串转换为PHP数组或对象。然而,
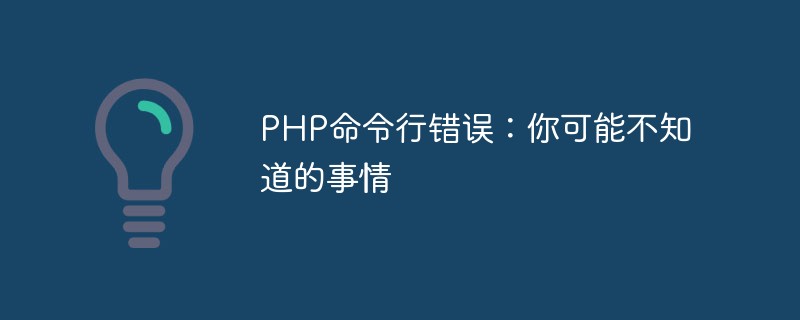
本文将介绍关于PHP命令行错误的一些你可能不知道的事情。PHP作为一门流行的服务器端语言,一般运行在Web服务器上,但它也可以在命令行上直接运行,比如在Linux或者MacOS系统下,我们可以在终端中输入“php”命令来直接运行PHP脚本。不过,就像在Web服务器中一样,当我们在命令行中运行PHP脚本时,也会遇到一些错误。以下是一些你可能不知道的有关PHP命
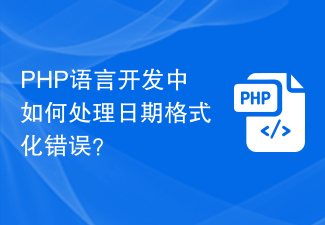
在PHP语言开发中,日期格式化错误是一个常见的问题。正确的日期格式对于程序员来说十分重要,因为它决定着代码的可读性、可维护性和正确性。本文将分享一些处理日期格式化错误的技巧。了解日期格式在处理日期格式化错误之前,我们必须先了解日期格式。日期格式是由各种字母和符号组成的字符串,用于表示特定的日期和时间格式。在PHP中,常见的日期格式包括:Y:四位数年份(如20
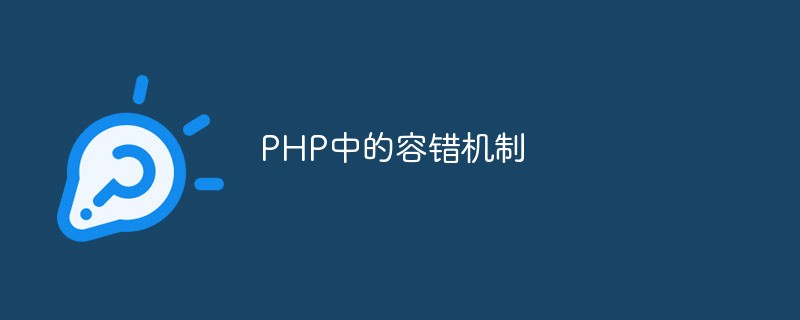
在编写程序时总会存在各种各样的错误和异常。任何编程语言都需要有良好的容错机制,PHP也不例外。PHP有许多内置的错误和异常处理机制,可以让开发者更好地管理其代码,并正确地处理各种问题。下面就让我们一起来了解一下PHP中的容错机制。错误级别PHP中有四个错误级别:致命错误、严重错误、警告和通知。每个错误级别都有一个不同的符号表示,以帮助识别和处理错误:E_ER
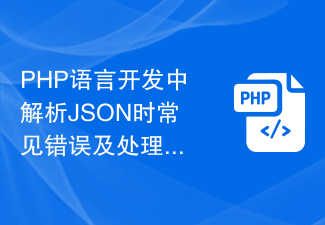
在PHP语言开发中,常常需要解析JSON数据,以便进行后续的数据处理和操作。然而,在解析JSON时,很容易遇到各种错误和问题。本文将介绍常见的错误和处理方法,帮助PHP开发者更好地处理JSON数据。一、JSON格式错误最常见的错误是JSON格式不正确。JSON数据必须符合JSON规范,即数据必须是键值对的集合,并使用大括号({})和中括号([])来包含数据。
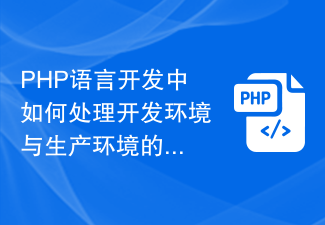
随着互联网的快速发展,开发人员的任务也随之多样化和复杂化。特别是对于PHP语言开发人员而言,在开发过程中面临的最常见问题之一就是在开发环境和生产环境中,数据不一致的错误问题。因此,在开发PHP应用程序时,如何处理这些错误是开发人员必须面对的一个重要问题。开发环境和生产环境的区别首先需要明确的是,开发环境和生产环境是不同的,它们有着不同的设置和配置。在开发环境
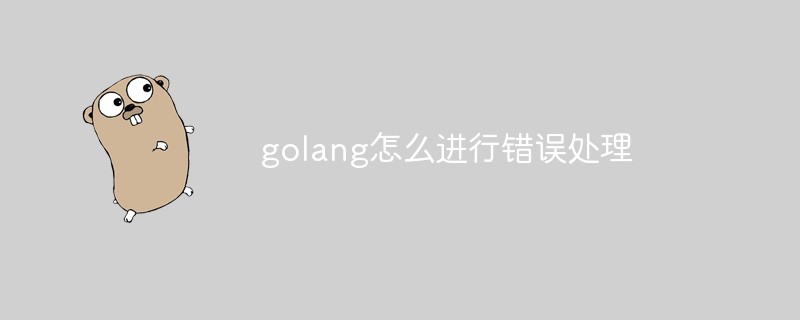
Golang通常有三种错误处理方式:错误哨兵(Sentinel Error)、错误类型断言和记录错误调用栈。错误哨兵指的是用特定值的变量作为错误处理分支的判定条件。错误类型用于路由错误处理逻辑,和错误哨兵有异曲同工的作用,由类型系统来提供错误种类的唯一性。错误黑盒指的是不过分关心错误类型,将错误返回给上层;当需要采取行动时,要针对错误的行为进行断言,而非错误类型。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
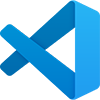
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
