RPC framework design and implementation case of swoole development function
RPC framework design and implementation case of Swoole development function
Introduction:
With the rapid development of the Internet, the demand for distributed systems is growing day by day. In a distributed system, communication between services is essential. RPC (Remote Procedure Call) is an important way to implement distributed systems. As a high-performance network communication framework, Swoole can quickly and efficiently implement the RPC framework. This article will introduce how to design and implement a powerful RPC framework with examples.
1. RPC framework design and ideas
The RPC framework mainly consists of two parts: the client and the server. The server is responsible for providing services, and the client is responsible for initiating requests and receiving processing results. In Swoole, we can use TCP or HTTP protocol to implement RPC communication. Swoole's coroutine technology can effectively improve the concurrency capability of a single machine, making RPC calls more efficient.
When designing the RPC framework, we need to consider the following points:
- Interface definition: Define the interface exposed by the server, including the interface name and parameter list and other information.
- Service registration: The server binds the interface to the implementation class and registers it with the service center for the client to call.
- Service discovery: The client needs to obtain the service provider's address and port information from the service center in order to make remote calls.
- Communication protocol: The client and server need to choose an appropriate communication protocol, such as TCP or Http, etc., as well as the corresponding serialization and deserialization methods.
- Load balancing: When making remote calls, the client needs to select a load balancing strategy to ensure that requests can be distributed evenly to multiple service providers.
2. RPC Framework Implementation Case
The following is a simple example to illustrate how to use Swoole to build an RPC framework.
First, we need to define an interface file, for example named HelloWorldInterface.php, the code is as follows:
<?php interface HelloWorldInterface { public function sayHello($name); }
Next, we need to implement this interface and create an implementation class HelloWorldService.php, the code As follows:
<?php class HelloWorldService implements HelloWorldInterface { public function sayHello($name) { return "Hello, " . $name; } }
Next, we need to register this service on the server side. Introduce the Swoole framework and create the Server.php file. The code is as follows:
<?php require __DIR__ . '/vendor/autoload.php'; class Server { private $server; public function __construct() { $this->server = new SwooleServer('127.0.0.1', 9501, SWOOLE_PROCESS, SWOOLE_SOCK_TCP); $this->server->set([ 'worker_num' => 2, ]); $this->server->on('Receive', [$this, 'onReceive']); } public function onReceive($server, $fd, $from_id, $data) { // 解析客户端发来的数据 $info = json_decode($data, true); if (empty($info['class']) || empty($info['method']) || empty($info['params'])) { return; } // 查找对应的类和方法 $class = $info['class']; $method = $info['method']; // 查找类的实例 $instance = new $class(); // 调用方法,返回结果 $result = call_user_func_array([$instance, $method], $info['params']); $server->send($fd, json_encode($result)); } public function start() { $this->server->start(); } } $server = new Server(); $server->start();
Finally, we can write a client to use this RPC framework to make remote calls. Create the Client.php file with the following code:
<?php require __DIR__ . '/vendor/autoload.php'; class Client { private $client; public function __construct() { $this->client = new SwooleClient(SWOOLE_SOCK_TCP); } public function call($class, $method, $params) { if (!$this->client->connect('127.0.0.1', 9501, -1)) { return false; } // 构造请求参数 $data = [ 'class' => $class, 'method' => $method, 'params' => $params, ]; // 发送请求 $this->client->send(json_encode($data)); // 接收响应 $result = $this->client->recv(); // 关闭连接 $this->client->close(); return json_decode($result, true); } } $client = new Client(); $result = $client->call('HelloWorldService', 'sayHello', ['Swoole']); var_dump($result);
In the above code, we create a Client class and use the Client object provided by Swoole to make remote calls. In the call method, first establish a connection with the server, then construct the request parameters, send the request and receive the response, and finally close the connection.
Run the Server.php and Client.php files to make remote calls and get the results.
Summary:
Through the cases in this article, we have learned the basic ideas and steps of using the Swoole framework to design and implement a powerful RPC framework. In actual development, we can expand and optimize according to specific needs to meet the needs of more complex and high-performance distributed systems. At the same time, Swoole provides rich coroutine and asynchronous IO support, which can better cope with high concurrency scenarios and provide better performance and reliability.
The above is the detailed content of RPC framework design and implementation case of swoole development function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 English version
Recommended: Win version, supports code prompts!
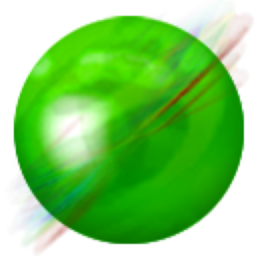
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool