Principles for selecting testing frameworks in Golang
Test framework selection principles in Golang
Introduction:
With the continuous development and growth of the Golang (Go for short) language, more and more developers are beginning to choose Go as their preferred programming language . During the development process, testing is a critical step in ensuring code quality. Choosing a suitable testing framework plays an important role in the success of the project. This article will introduce some principles for choosing a Golang testing framework and give corresponding code examples.
1. Introduction
Go language has a built-in lightweight testing framework, namely the testing package. It provides a set of functions and utilities for writing test cases. In addition to the built-in testing package, Go also has many excellent third-party testing frameworks, such as GoConvey, Testify, etc. Choosing a suitable testing framework can improve the usability and maintainability of testing, thereby improving development efficiency.
2. Selection Principle
- Project Requirements
The selection of testing framework should be based on the specific needs of the project. Different projects may have different requirements for testing frameworks, such as test case writing and management, test report generation, concurrent testing support, etc. Therefore, before choosing a testing framework, you need to clarify the project requirements and compare the functions of each testing framework. - Community Activity
Choosing a testing framework with high community activity can bring more benefits, such as better technical support, problem solutions, and reading materials. An active testing framework usually has more developers contributing, giving more comprehensive test coverage. In addition, an active community can provide more test plugins and extensions to meet the specific needs of the project. - Documentation Completeness
The documentation completeness of the testing framework directly affects the developer's learning curve and usage experience. Document content should be clear, concise, and easy to understand. At the same time, a good document should provide rich sample code to help developers better understand and use the testing framework.
3. Sample Code
Below we will introduce two very popular Golang testing frameworks: GoConvey and Testify based on the sample code.
- GoConvey
GoConvey is a minimalist Golang testing framework known for its simple coding style, friendly user interface and rich assertion library. The following is an example of testing using GoConvey:
package main import ( "testing" . "github.com/smartystreets/goconvey/convey" ) func TestAddition(t *testing.T) { Convey("Given two numbers", t, func() { a := 2 b := 3 Convey("When adding them together", func() { result := a + b Convey("The result should be 5", func() { So(result, ShouldEqual, 5) }) }) }) }
In the above code, we use GoConvey's syntax style, combined with the assertion library. Through the two functions Convey
and So
, we can build test cases and make assertions. Run the test function and we can see the test results in GoConvey's user interface.
- Testify
Testify is another popular Golang testing framework that provides a rich set of assertion functions and utilities. The following is an example of testing using Testify:
package main import ( "testing" "github.com/stretchr/testify/assert" ) func TestMultiplication(t *testing.T) { result := multiply(2, 3) assert.Equal(t, 6, result, "The result should be 6") } func multiply(a, b int) int { return a * b }
In the above code, we use Testify’s assertion function assert.Equal
to make assertions. The assert.Equal
function compares the actual result with the expected result and outputs an error message if they are inconsistent. In this way, we can easily make assertions and ensure logical correctness.
Conclusion:
When choosing the Golang testing framework, we should consider factors such as project needs, community activity, and documentation completeness. GoConvey and Testify are two powerful and easy-to-use testing frameworks, which are suitable for different demand scenarios. By rationally selecting the testing framework, we can improve the efficiency and quality of testing and ensure the success of the project.
(word count: 748)
The above is the detailed content of Principles for selecting testing frameworks in Golang. For more information, please follow other related articles on the PHP Chinese website!
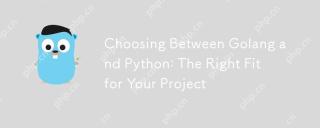
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
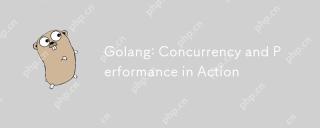
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
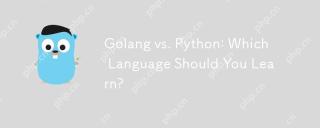
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
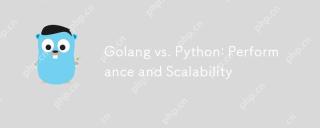
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
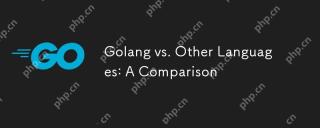
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
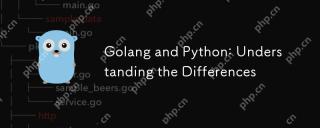
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
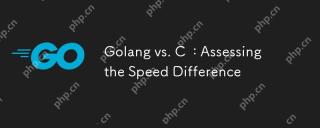
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
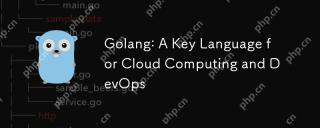
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
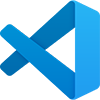
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
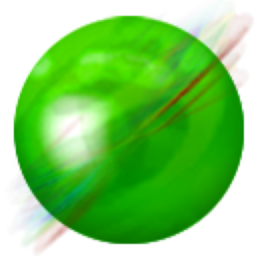
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment