


How to use PHP crawler to solve the verification code identification problem?
Introduction:
In web crawler development, verification code identification is a commonly encountered problem. Verification codes are usually used to verify user identities or prevent malicious crawling of data, but for automated crawlers, verification codes often become an insurmountable obstacle. In this article, we will introduce how to use PHP crawler classes to solve the verification code identification problem and provide corresponding code examples.
1. Understand the verification code
The verification code (CAPTCHA) is an image verification technology used to distinguish computers and humans. Common verification code types include numeric verification codes, letter verification codes, picture selection verification codes, etc. For ordinary users, these verification codes are easy to identify, but for automated crawlers, identifying these verification codes becomes complicated.
2. Solution
In order to solve the verification code identification problem, we can use some third-party verification code identification services, such as coding platforms or machine learning models. These services generally provide API interfaces and return recognition results by uploading verification code images. This article will take the coding platform as an example to introduce how to integrate the verification code recognition function into the PHP crawler.
- Register and obtain the API key of the coding platform
Go to the official website of the coding platform to register an account and log in, enter the personal center, and obtain the API key. Save the API key, you will need it later. -
Install third-party HTTP request library and crawler library
Use Composer to easily install third-party libraries. Execute the following command in the project directory:composer require guzzlehttp/guzzle composer require symfony/dom-crawler
-
Write the crawler class
<?php require 'vendor/autoload.php'; use GuzzleHttpClient; use SymfonyComponentDomCrawlerCrawler; class CrawlerExample { private $client; public function __construct() { $this->client = new Client([ // 配置HTTP请求库,可添加代理、设置请求超时等 ]); } // 获取需要识别的验证码图片 private function getVerificationCode() { $response = $this->client->request('GET', 'http://example.com/verification_code_url'); $content = $response->getBody()->getContents(); $crawler = new Crawler($content); // 获取验证码图片的URL $imageUrl = $crawler->filter('img#verification_code')->attr('src'); return $imageUrl; } // 通过打码平台识别验证码 private function recognizeVerificationCode($imageUrl, $apiKey) { $response = $this->client->request('POST', 'http://api.dama2.com:7766/app/d2Url', [ 'form_params' => [ 'url' => $imageUrl, 'appID' => $apiKey, ], ]); $result = $response->getBody()->getContents(); return $result; } // 主逻辑 public function run($apiKey) { $imageUrl = $this->getVerificationCode(); $result = $this->recognizeVerificationCode($imageUrl, $apiKey); // 进行后续操作,如提交表单等 } } $example = new CrawlerExample(); $example->run('your_api_key'); ?>
- Run the crawler
Replacehttp:// in the code example.com/verification_code_url
is the actual verification code image URL. Replaceyour_api_key
with the API key obtained on the coding platform. Run the script and the crawler will automatically obtain the verification code and identify it. -
Other Notes
- The URL of the verification code image may change and needs to be adjusted accordingly according to the actual situation.
- Coding platforms generally charge a certain fee, and the cost needs to be considered.
- It is necessary to set a reasonable request interval and exception handling mechanism to avoid crawling failures caused by excessive access frequency or network abnormalities.
Conclusion:
This article introduces how to use PHP crawler class to solve the verification code identification problem. By using the API service of a third-party coding platform, the verification code recognition function can be easily integrated into the crawler. Of course, there are still situations where special types of verification codes cannot be recognized, in which case other technical means or manual intervention may be needed to solve the problem.
The above is the detailed content of How to use PHP crawler to solve the verification code identification problem?. For more information, please follow other related articles on the PHP Chinese website!
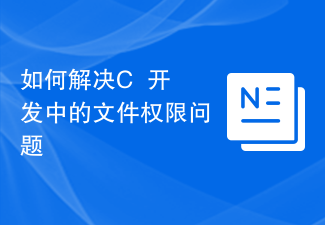
如何解决C++开发中的文件权限问题在C++开发过程中,文件权限问题是一个常见的挑战。在许多情况下,我们需要以不同的权限访问和操作文件,例如读取、写入、执行和删除文件。本文将介绍一些解决C++开发中文件权限问题的方法。一、了解文件权限在解决文件权限问题之前,我们首先需要了解文件权限的基本概念。文件权限指的是文件的拥有者、拥有组和其他用户对文件的访问权限。在Li
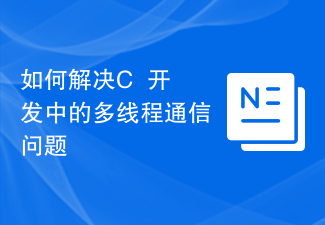
如何解决C++开发中的多线程通信问题多线程编程是现代软件开发中常见的一种编程方式,它可以使程序在执行过程中同时进行多个任务,提高了程序的并发性和响应能力。然而,多线程编程也会带来一些问题,其中一个重要的问题就是多线程之间的通信。在C++开发中,多线程通信指的是不同线程之间进行数据或消息的传递和共享。正确有效的多线程通信对于保证程序的正确性和性能至关重要。本文
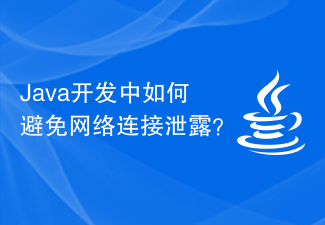
如何解决Java开发中的网络连接泄露问题随着信息技术的高速发展,网络连接在Java开发中变得越来越重要。然而,Java开发中的网络连接泄露问题也逐渐凸显出来。网络连接泄露会导致系统性能下降、资源浪费以及系统崩溃等问题,因此解决网络连接泄露问题变得至关重要。网络连接泄露是指在Java开发中未正确关闭网络连接,导致连接资源无法释放,从而使系统无法正常工作。解决网
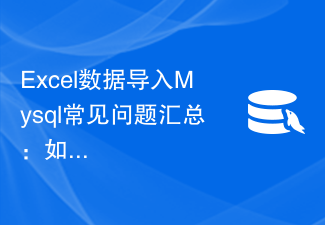
Excel数据导入Mysql常见问题汇总:如何解决字段类型不匹配的问题?导入数据是数据库管理中一个非常常见的操作,而Excel作为一款常用的数据处理工具,通常被用于数据的收集和整理。然而,在将Excel数据导入到Mysql数据库时,可能会遇到字段类型不匹配的问题。本文将围绕这个问题展开讨论,并提供一些解决方案。首先,我们来了解一下字段类型不匹配的问题出现的原
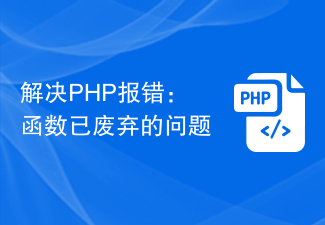
解决PHP报错:函数已废弃的问题在使用PHP进行开发或维护过程中,时常会遇到一些老旧代码或第三方库的问题,其中之一就是函数已废弃的警告或错误。PHP在进行版本升级时,通常会将某些函数标记为已废弃(deprecated),并在后续版本中逐步移除或替换。这样做是为了提醒开发者使用更可靠、更高效的方式来实现相同的功能。本文将介绍如何解决PHP报错中的函数已废弃问题
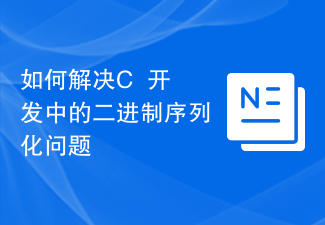
如何解决C++开发中的二进制序列化问题序列化在软件开发中是一个常见的概念,它将数据结构或对象转换成一种字节流的形式,以便在不同平台或不同语言中进行传输或存储。二进制序列化是一种快速且高效的序列化方式,特别在C++开发中广泛应用。然而,二进制序列化也会带来一些挑战,例如跨平台兼容性、数据结构变化等问题。本文将探讨在C++开发中如何解决二进制序列化问题。首先,针
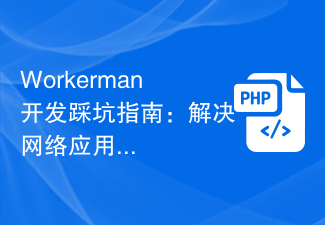
Workerman开发踩坑指南:解决网络应用中常见问题的经验总结与分享引言:在网络应用开发过程中,我们经常会遇到一些棘手的问题。本文将结合实际经验,提供一些解决这些问题的经验总结和分享。我们将以Workerman作为开发框架,并提供相关代码示例。一、EventLoop的理解与优化Workerman是一个基于EventLoop的开发框架,了解EventL
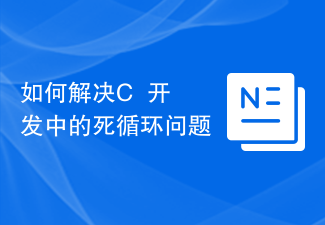
如何解决C++开发中的死循环问题在C++开发中,死循环是一种非常常见却又非常棘手的问题。当程序陷入死循环时,会导致程序无法正常执行,甚至可能导致系统崩溃。因此,解决死循环问题是C++开发中必不可少的技能之一。本文将介绍一些常见的解决死循环问题的方法。检查循环条件死循环的最常见原因之一就是循环条件不正确。当循环条件一直为真时,循环就会一直执行下去,导致陷入死循


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
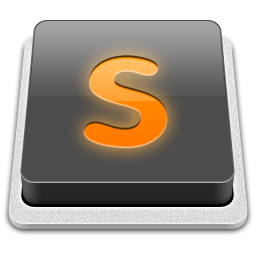
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
