


How to use Python to develop the data statistics function of CMS system
Introduction: With the rapid development of the Internet, content management systems (CMS) are widely used in websites, blogs and other platforms to help users quickly build and manage Website content. As developers, we need to add various practical functions to the CMS system, among which data statistics is a very important one. This article will introduce how to use Python to develop the data statistics function of the CMS system, and attach code examples to help readers better implement this function.
1. Target requirement analysis
Before developing the data statistics function, we first need to clarify the specific requirements. Different websites may have different statistical needs, and we need to carry out customized development according to the actual situation. The following are some common statistical requirements:
- Visit statistics: count the total number of visits to the website every day, week, and month, so as to understand the traffic status of the website.
- Page visit statistics: Count the independent visits of each page, including the number of visitors, the number of visits and other information, to facilitate the analysis of the popularity of the website content.
- User behavior statistics: Statistics of user behavior data, such as the number of registrations, logins, comments, etc., to help optimize the user experience of the website.
2. Technical Solution Design
Before designing the technical solution, we need to determine a key issue: the storage method of data. Usually, the data statistics function needs to save the statistical results in the database for later query and analysis. Here we choose MySQL as the database and use Python's database operation library pymysql
to perform data access operations.
- Visit statistics
For traffic statistics, we can record user visits by inserting a piece of statistical code at the entrance of the website. This statistical code can be implemented using Python's Flask framework.
The following is a sample code:
from flask import Flask, request import pymysql app = Flask(__name__) db = pymysql.connect(host='localhost', user='root', password='123456', database='cms') @app.route('/') def index(): # 记录访问数据 cursor = db.cursor() sql = "INSERT INTO visit (ip, page) VALUES ('%s', '%s')" % (request.remote_addr, request.path) cursor.execute(sql) db.commit() cursor.close() # 返回页面内容 return 'Hello, World!' if __name__ == '__main__': app.run()
The above code uses the Flask framework to create a simple website and records the user's visit data in the MySQL databasevisit
table.
- Page visit statistics
For page visit statistics, we can insert a statistical code block in the back-end code of each page to record visits and other data . The following is a sample code:
from flask import Flask, request import pymysql app = Flask(__name__) db = pymysql.connect(host='localhost', user='root', password='123456', database='cms') @app.route('/page1') def page1(): # 记录页面访问数据 cursor = db.cursor() sql = "INSERT INTO page (page, ip) VALUES ('%s', '%s')" % (request.path, request.remote_addr) cursor.execute(sql) db.commit() cursor.close() # 返回页面内容 return 'This is page 1' @app.route('/page2') def page2(): # 记录页面访问数据 cursor = db.cursor() sql = "INSERT INTO page (page, ip) VALUES ('%s', '%s')" % (request.path, request.remote_addr) cursor.execute(sql) db.commit() cursor.close() # 返回页面内容 return 'This is page 2' if __name__ == '__main__': app.run()
The above code uses the Flask framework to create two pages, and records the access data of each page in the page
table in the MySQL database.
- User Behavior Statistics
For user behavior statistics, we can insert a statistical code into the corresponding operation code to record data such as the number of operations. The following is a sample code:
from flask import Flask, request import pymysql app = Flask(__name__) db = pymysql.connect(host='localhost', user='root', password='123456', database='cms') @app.route('/register', methods=['POST']) def register(): # 记录注册数据 cursor = db.cursor() sql = "INSERT INTO action (action, count) VALUES ('register', 1)" cursor.execute(sql) db.commit() cursor.close() # 返回注册成功的消息 return 'Register success' @app.route('/login', methods=['POST']) def login(): # 记录登录数据 cursor = db.cursor() sql = "INSERT INTO action (action, count) VALUES ('login', 1)" cursor.execute(sql) db.commit() cursor.close() # 返回登录成功的消息 return 'Login success' if __name__ == '__main__': app.run()
The above code uses the Flask framework to create two interfaces for registration and login, and records the number of each operation in the action
table in the MySQL database.
3. Query and analysis of statistical results
After completing the statistics and storage of data, we still need to write code to query and analyze the statistical results. The following is a sample code:
import pymysql db = pymysql.connect(host='localhost', user='root', password='123456', database='cms') # 查询网站的总访问量 def get_total_visits(): cursor = db.cursor() sql = "SELECT COUNT(*) FROM visit" cursor.execute(sql) result = cursor.fetchone() cursor.close() return result[0] # 查询指定页面的访问量 def get_page_visits(page): cursor = db.cursor() sql = "SELECT COUNT(*) FROM page WHERE page='%s'" % page cursor.execute(sql) result = cursor.fetchone() cursor.close() return result[0] # 查询指定操作的次数 def get_action_count(action): cursor = db.cursor() sql = "SELECT count FROM action WHERE action='%s'" % action cursor.execute(sql) result = cursor.fetchone() cursor.close() return result[0] if __name__ == '__main__': print("网站总访问量:", get_total_visits()) print("页面访问量:") print(" - 页面1:", get_page_visits('/page1')) print(" - 页面2:", get_page_visits('/page2')) print("注册次数:", get_action_count('register')) print("登录次数:", get_action_count('login'))
The above code uses the pymysql library to connect to the database and writes several functions to query data for different statistical results.
Summary: Through the above code examples, we show how to use Python to develop the data statistics function of the CMS system. Of course, this is just a simple example, and specific needs and functions can be expanded and customized according to actual conditions. I hope this article can inspire and help readers in the process of developing CMS systems.
The above is the detailed content of How to use Python to develop the data statistics function of CMS system. For more information, please follow other related articles on the PHP Chinese website!
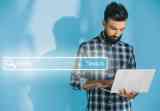
Long URLs, often cluttered with keywords and tracking parameters, can deter visitors. A URL shortening script offers a solution, creating concise links ideal for social media and other platforms. These scripts are valuable for individual websites a
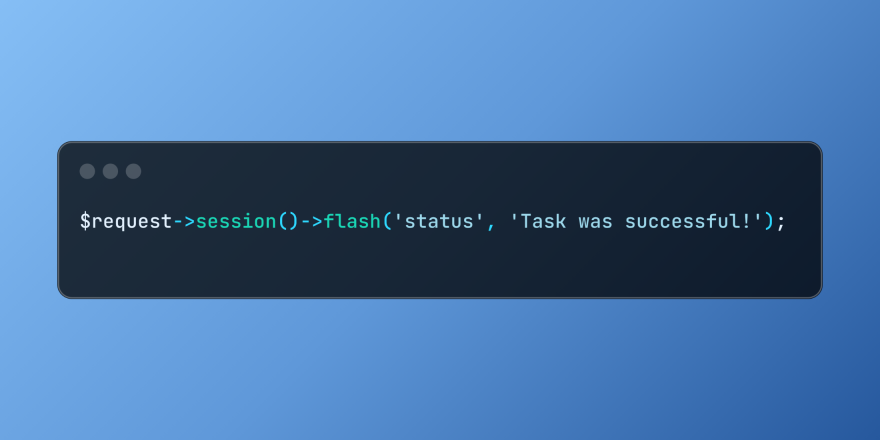
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
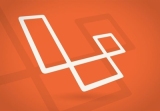
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
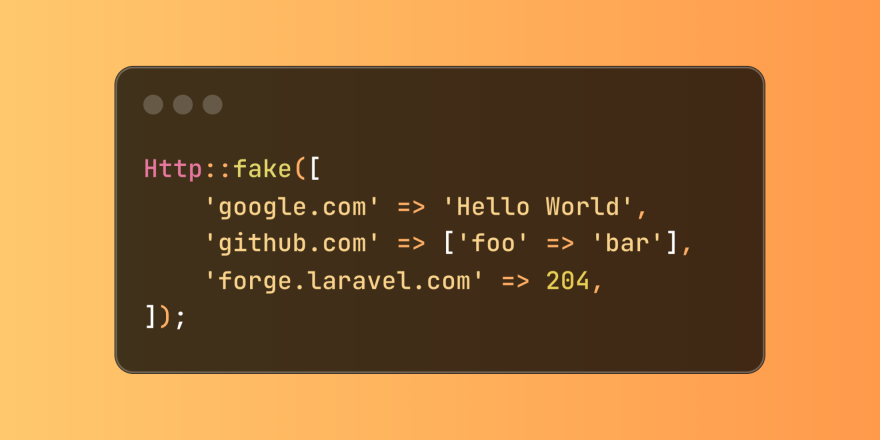
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
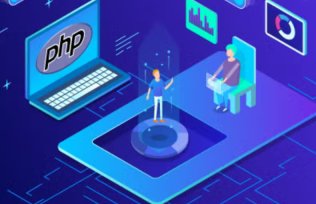
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
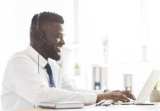
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
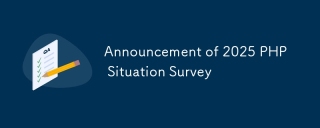
The 2025 PHP Landscape Survey investigates current PHP development trends. It explores framework usage, deployment methods, and challenges, aiming to provide insights for developers and businesses. The survey anticipates growth in modern PHP versio
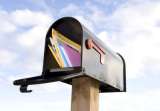
In this article, we're going to explore the notification system in the Laravel web framework. The notification system in Laravel allows you to send notifications to users over different channels. Today, we'll discuss how you can send notifications ov


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
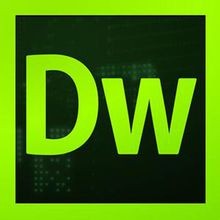
Dreamweaver CS6
Visual web development tools
