


Efficient connection and interaction between Swoole development functions and MySQL database
With the rapid development of the Internet and the widespread popularity of applications, high-performance server-side development frameworks have become more and more important. Swoole is a high-performance network communication engine and server-side framework based on PHP, which can greatly improve the performance and concurrency capabilities of PHP applications. In development, efficient connection and interaction with the database is a very important part. This article will introduce how to use Swoole to achieve efficient connection and interaction with MySQL database, and give corresponding code examples.
First of all, we need to introduce Swoole and MySQL related extensions into the project.
require_once 'path/to/swoole/autoload.php'; use SwooleCoroutine as Co; use SwooleDatabaseMySQLiConfig; use SwooleDatabaseMySQLiException; use SwooleDatabaseMySQLPool;
Next, we need to configure the MySQL connection parameters and create a connection pool.
$mysqlConfig = new MySQLiConfig([ 'host' => 'localhost', 'port' => 3306, 'user' => 'root', 'password' => 'password', 'database' => 'test', ]); $pool = new MySQLPool($mysqlConfig, 10);
The above code creates a MySQL connection pool with a size of 10. You can adjust the size of the connection pool as needed. Next, we can use Swoole's coroutine to achieve efficient connection and interaction.
Coun(function () use ($pool) { $conn = $pool->get(); if ($conn == false) { echo "Failed to get connection from pool."; return; } $result = $conn->query("SELECT * FROM table"); if ($result == false) { echo "Failed to execute query."; return; } while ($row = $result->fetch_assoc()) { echo $row['column1']; } $pool->put($conn); });
The above code uses coroutines to obtain a MySQL connection from the connection pool, then performs query operations, and finally puts the connection back into the connection pool. By using coroutines, we can efficiently reuse MySQL connections, reduce connection creation and destruction overhead, and improve overall performance.
In addition, Swoole also provides some other functions to optimize database connections and interactions:
- Swoole’s connection pool management mechanism can automatically detect the health status of the connection. When the connection is abnormal, It will automatically reconnect when disconnected to maintain the stability and reliability of the connection.
- Swoole uses an asynchronous and non-blocking method for database connection and interaction, which greatly improves concurrency capabilities. In high-concurrency scenarios, multiple requests can be processed simultaneously, improving system throughput.
- Swoole supports the use of coroutines for database transaction management, which can simplify the use and control of transactions, and provides an exception handling mechanism to ensure the consistency and reliability of transactions.
To sum up, Swoole provides efficient MySQL connection and interaction functions. By using connection pools and coroutines, connection reuse and asynchronous non-blocking interaction can be achieved, improving system performance. and concurrency capabilities. In actual application development, we can configure the size of the connection pool according to specific needs and scenarios, and use various interfaces and functions provided by Swoole according to specific business logic to achieve efficient MySQL database connection and interaction.
I hope this article can help readers understand and use the Swoole development function to efficiently connect and interact with the MySQL database. If you have any questions or concerns, please feel free to ask and communicate. Thanks!
The above is the detailed content of Efficient connection and interaction between swoole development functions and MySQL database. For more information, please follow other related articles on the PHP Chinese website!
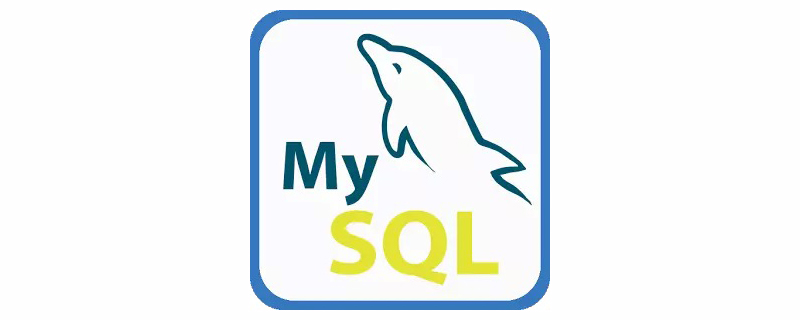
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于架构原理的相关内容,MySQL Server架构自顶向下大致可以分网络连接层、服务层、存储引擎层和系统文件层,下面一起来看一下,希望对大家有帮助。
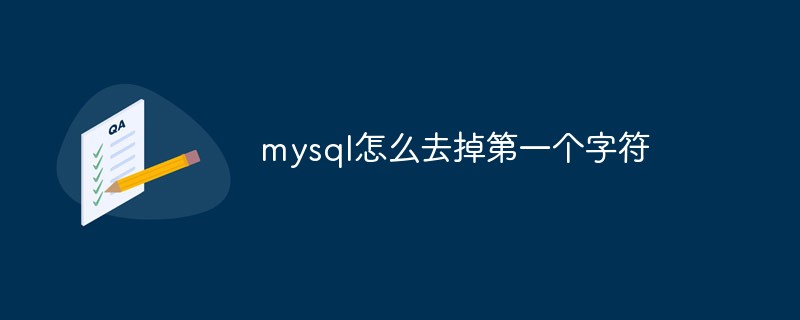
方法:1、利用right函数,语法为“update 表名 set 指定字段 = right(指定字段, length(指定字段)-1)...”;2、利用substring函数,语法为“select substring(指定字段,2)..”。
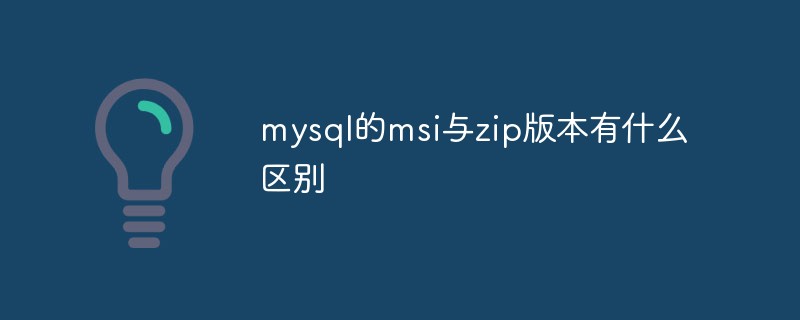
mysql的msi与zip版本的区别:1、zip包含的安装程序是一种主动安装,而msi包含的是被installer所用的安装文件以提交请求的方式安装;2、zip是一种数据压缩和文档存储的文件格式,msi是微软格式的安装包。
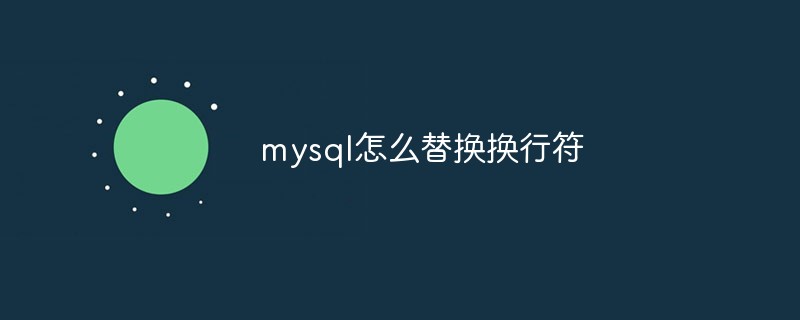
在mysql中,可以利用char()和REPLACE()函数来替换换行符;REPLACE()函数可以用新字符串替换列中的换行符,而换行符可使用“char(13)”来表示,语法为“replace(字段名,char(13),'新字符串') ”。
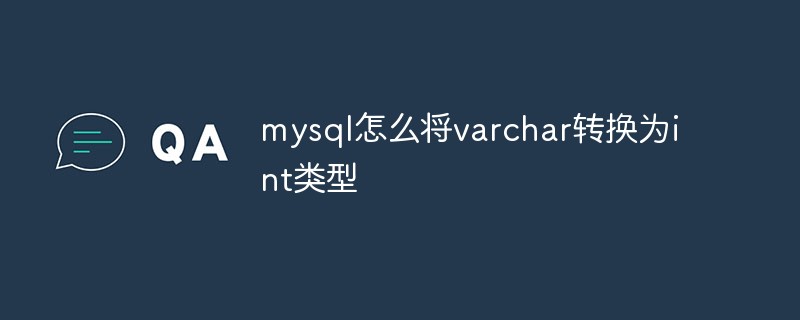
转换方法:1、利用cast函数,语法“select * from 表名 order by cast(字段名 as SIGNED)”;2、利用“select * from 表名 order by CONVERT(字段名,SIGNED)”语句。
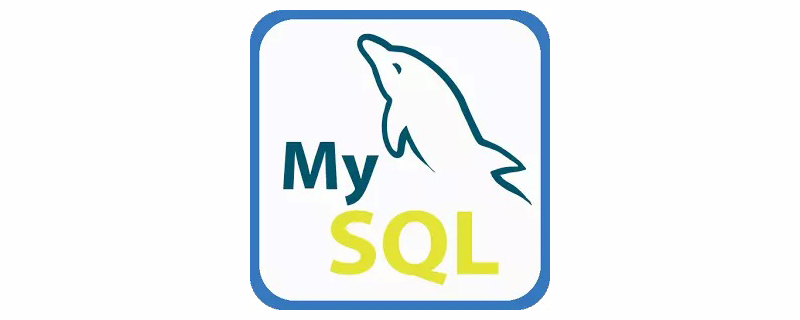
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于MySQL复制技术的相关问题,包括了异步复制、半同步复制等等内容,下面一起来看一下,希望对大家有帮助。
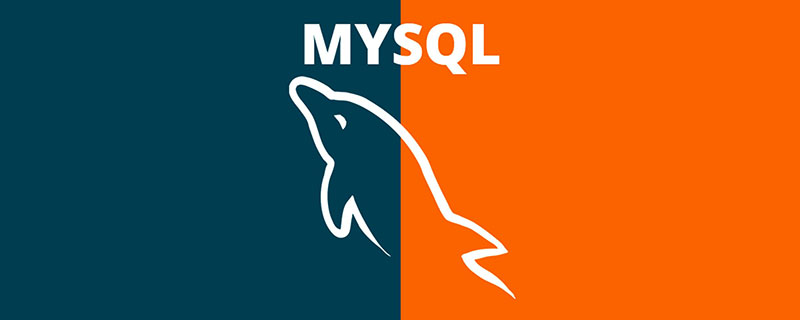
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了mysql高级篇的一些问题,包括了索引是什么、索引底层实现等等问题,下面一起来看一下,希望对大家有帮助。
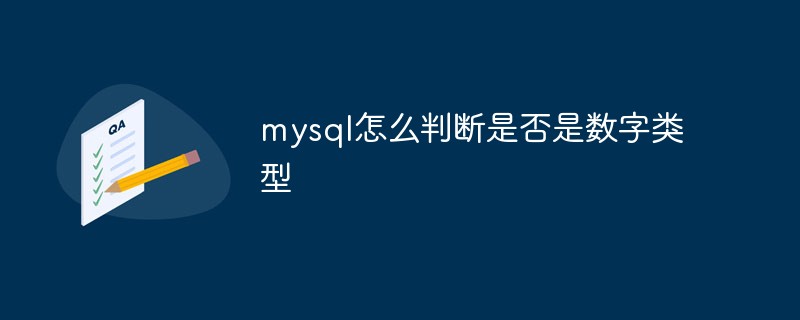
在mysql中,可以利用REGEXP运算符判断数据是否是数字类型,语法为“String REGEXP '[^0-9.]'”;该运算符是正则表达式的缩写,若数据字符中含有数字时,返回的结果是true,反之返回的结果是false。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
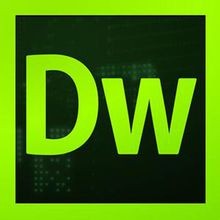
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
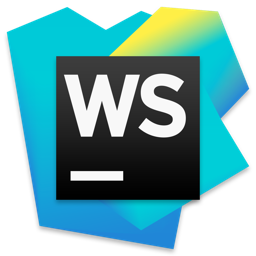
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
